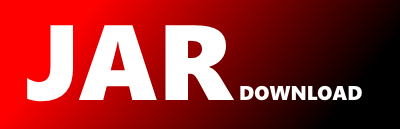
io.bootique.logback.policy.FileNamePatternValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bootique-logback Show documentation
Show all versions of bootique-logback Show documentation
Provides Logback integration with Bootique
package io.bootique.logback.policy;
import ch.qos.logback.classic.LoggerContext;
import ch.qos.logback.core.rolling.helper.DateTokenConverter;
import ch.qos.logback.core.rolling.helper.FileNamePattern;
import ch.qos.logback.core.rolling.helper.IntegerTokenConverter;
import ch.qos.logback.core.rolling.helper.RollingCalendar;
import java.util.Locale;
abstract class FileNamePatternValidator {
private static final String SEE_LOGBACK_APPENDER = "See http://logback.qos.ch/manual/appenders.html";
private static final String MISSING_INTEGER_TOKEN = "Missing integer token, that is %%i, in property \"fileNamePattern\" [%s]";
private static final String UNEXPECTED_INTEGER_TOKEN = "Unexpected integer token, that is %%i, in property \"fileNamePattern\" [%s]";
private static final String MISSING_DATE_TOKEN = "Missing date token, that is %%d, in property \"fileNamePattern\" [%s]";
private static final String UNEXPECTED_DATE_TOKEN = "Unexpected date token, that is %%d, in property \"fileNamePattern\" [%s]";
private static final String INCORRECT_DATE_FORMAT = "Incorrect date format in the date token in property \"fileNamePattern\" [%s]";
private LoggerContext context;
private String fileNamePattern;
private String docRefId;
FileNamePatternValidator(LoggerContext context, String fileNamePattern, String docRefId) {
this.context = context;
this.fileNamePattern = fileNamePattern;
this.docRefId = docRefId;
}
abstract void validate();
void checkPattern(boolean dateTokenExists, boolean integerTokenExists) {
checkPatternMandatory();
checkDateToken(dateTokenExists);
checkIntegerToken(integerTokenExists);
}
void checkPatternMandatory() {
if (fileNamePattern == null || fileNamePattern.isEmpty()) {
throw new IllegalStateException("The property \"fileNamePattern\" is mandatory");
}
}
void checkDateToken(boolean checkOnExisting) {
FileNamePattern pattern = new FileNamePattern(fileNamePattern, context);
DateTokenConverter
© 2015 - 2024 Weber Informatics LLC | Privacy Policy