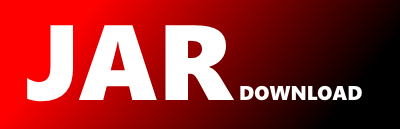
brooklyn.entity.rebind.dto.BrooklynMementoImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.entity.rebind.dto;
import java.io.Serializable;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import brooklyn.BrooklynVersion;
import brooklyn.mementos.BrooklynMemento;
import brooklyn.mementos.EntityMemento;
import brooklyn.mementos.LocationMemento;
import brooklyn.mementos.PolicyMemento;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
public class BrooklynMementoImpl implements BrooklynMemento, Serializable {
private static final long serialVersionUID = -5848083830410137654L;
public static Builder builder() {
return new Builder();
}
public static class Builder {
protected String brooklynVersion = BrooklynVersion.get();
protected List applicationIds = Lists.newArrayList();
protected List topLevelLocationIds = Lists.newArrayList();
protected Map entities = Maps.newLinkedHashMap();
protected Map locations = Maps.newLinkedHashMap();
protected Map policies = Maps.newLinkedHashMap();
public Builder brooklynVersion(String val) {
brooklynVersion = val; return this;
}
public Builder applicationId(String val) {
applicationIds.add(val); return this;
}
public Builder applicationIds(List vals) {
applicationIds.addAll(vals); return this;
}
public Builder topLevelLocationIds(List vals) {
topLevelLocationIds.addAll(vals); return this;
}
public Builder entities(Map vals) {
entities.putAll(vals); return this;
}
public Builder locations(Map vals) {
locations.putAll(vals); return this;
}
public Builder policy(PolicyMemento val) {
policies.put(val.getId(), val); return this;
}
public Builder entity(EntityMemento val) {
entities.put(val.getId(), val); return this;
}
public Builder location(LocationMemento val) {
locations.put(val.getId(), val); return this;
}
public Builder policies(Map vals) {
policies.putAll(vals); return this;
}
public BrooklynMemento build() {
return new BrooklynMementoImpl(this);
}
}
private String brooklynVersion;
private List applicationIds;
private List topLevelLocationIds;
private Map entities;
private Map locations;
private Map policies;
private BrooklynMementoImpl(Builder builder) {
brooklynVersion = builder.brooklynVersion;
applicationIds = builder.applicationIds;
topLevelLocationIds = builder.topLevelLocationIds;
entities = builder.entities;
locations = builder.locations;
policies = builder.policies;
}
@Override
public EntityMemento getEntityMemento(String id) {
return entities.get(id);
}
@Override
public LocationMemento getLocationMemento(String id) {
return locations.get(id);
}
@Override
public PolicyMemento getPolicyMemento(String id) {
return policies.get(id);
}
@Override
public Collection getApplicationIds() {
return ImmutableList.copyOf(applicationIds);
}
@Override
public Collection getEntityIds() {
return Collections.unmodifiableSet(entities.keySet());
}
@Override
public Collection getLocationIds() {
return Collections.unmodifiableSet(locations.keySet());
}
@Override
public Collection getPolicyIds() {
return Collections.unmodifiableSet(policies.keySet());
}
@Override
public Collection getTopLevelLocationIds() {
return Collections.unmodifiableList(topLevelLocationIds);
}
@Override
public Map getEntityMementos() {
return Collections.unmodifiableMap(entities);
}
@Override
public Map getLocationMementos() {
return Collections.unmodifiableMap(locations);
}
@Override
public Map getPolicyMementos() {
return Collections.unmodifiableMap(policies);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy