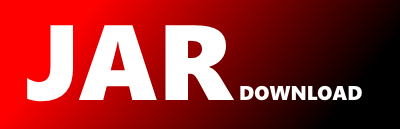
brooklyn.util.task.BasicExecutionContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.util.task;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.Callable;
import java.util.concurrent.Executor;
import java.util.concurrent.ExecutorService;
import brooklyn.management.ExecutionContext;
import brooklyn.management.ExecutionManager;
import brooklyn.management.Task;
import com.google.common.base.Function;
import com.google.common.collect.Maps;
/**
* A means of executing tasks against an ExecutionManager with a given bucket/set of tags pre-defined
* (so that it can look like an {@link Executor} and also supply {@link ExecutorService#submit(Callable)}
*/
public class BasicExecutionContext extends AbstractExecutionContext {
static final ThreadLocal perThreadExecutionContext = new ThreadLocal();
public static BasicExecutionContext getCurrentExecutionContext() { return perThreadExecutionContext.get(); }
/** @deprecated in 0.4.0, use Tasks.current() */
public Task> getCurrentTask() { return BasicExecutionManager.getCurrentTask(); }
final ExecutionManager executionManager;
final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy