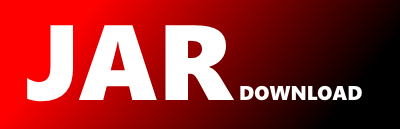
brooklyn.util.xstream.StringKeyMapConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.util.xstream;
import java.util.Map;
import java.util.Map.Entry;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import brooklyn.util.collections.MutableMap;
import brooklyn.util.exceptions.Exceptions;
import brooklyn.util.flags.TypeCoercions;
import brooklyn.util.text.Identifiers;
import com.thoughtworks.xstream.converters.MarshallingContext;
import com.thoughtworks.xstream.converters.UnmarshallingContext;
import com.thoughtworks.xstream.io.ExtendedHierarchicalStreamWriterHelper;
import com.thoughtworks.xstream.io.HierarchicalStreamReader;
import com.thoughtworks.xstream.io.HierarchicalStreamWriter;
import com.thoughtworks.xstream.mapper.Mapper;
/** converter which simplifies representation of a map for string-based keys,
* to value , or value
* @author alex
*
*/
public class StringKeyMapConverter extends MapConverter {
private static final Logger log = LoggerFactory.getLogger(StringKeyMapConverter.class);
// full stop is technically allowed ... goes against "best practice" ...
// but simplifies property maps, and is used elsewhere in xstream's repn
final static String VALID_XML_NODE_NAME_CHARS = Identifiers.JAVA_VALID_NONSTART_CHARS + ".";
public StringKeyMapConverter(Mapper mapper) {
super(mapper);
}
protected boolean isKeyValidForNodeName(String key) {
// return false to always write as type) {
return TypeCoercions.isPrimitiveOrBoxer(type) || String.class.equals(type) || type.isEnum();
}
@Override
protected void unmarshalEntry(HierarchicalStreamReader reader, UnmarshallingContext context, Map map) {
String key = reader.getNodeName();
if (key.equals(getEntryNodeName())) key = reader.getAttribute("key");
if (key==null) {
super.unmarshalEntry(reader, context, map);
} else {
unmarshalStringKey(reader, context, map, key);
}
}
protected void unmarshalStringKey(HierarchicalStreamReader reader, UnmarshallingContext context, Map map, String key) {
String type = reader.getAttribute("type");
Object value;
if (type==null && reader.hasMoreChildren()) {
reader.moveDown();
value = readItem(reader, context, map);
reader.moveUp();
} else {
Class typeC = type!=null ? mapper().realClass(type) : String.class;
try {
value = TypeCoercions.coerce(reader.getValue(), typeC);
} catch (Exception e) {
log.warn("FAILED to coerce "+reader.getValue()+" to "+typeC+": "+e);
throw Exceptions.propagate(e);
}
}
map.put(key, value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy