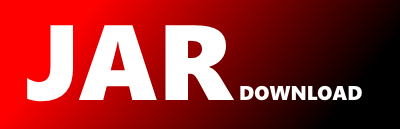
brooklyn.catalog.internal.BasicBrooklynCatalog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.catalog.internal;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.NoSuchElementException;
import javax.annotation.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import brooklyn.catalog.BrooklynCatalog;
import brooklyn.catalog.CatalogItem;
import brooklyn.catalog.CatalogPredicates;
import brooklyn.management.ManagementContext;
import brooklyn.util.collections.MutableMap;
import brooklyn.util.exceptions.Exceptions;
import brooklyn.util.javalang.AggregateClassLoader;
import brooklyn.util.javalang.LoadedClassLoader;
import brooklyn.util.time.Time;
import com.google.common.base.Function;
import com.google.common.base.Preconditions;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Iterables;
public class BasicBrooklynCatalog implements BrooklynCatalog {
private static final Logger log = LoggerFactory.getLogger(BasicBrooklynCatalog.class);
private final ManagementContext mgmt;
private final CatalogDo catalog;
private volatile CatalogDo manualAdditionsCatalog;
private volatile LoadedClassLoader manualAdditionsClasses;
public BasicBrooklynCatalog(final ManagementContext mgmt, final CatalogDto dto) {
this.mgmt = Preconditions.checkNotNull(mgmt, "managementContext");
this.catalog = new CatalogDo(dto);
mgmt.getExecutionManager().submit(MutableMap.of("name", "loading catalog"), new Runnable() {
public void run() {
log.debug("Loading catalog for "+mgmt);
catalog.load(mgmt, null);
if (log.isDebugEnabled())
log.debug("Loaded catalog for "+mgmt+": "+catalog+"; search classpath is "+catalog.getRootClassLoader());
}
});
}
public CatalogDo getCatalog() {
return catalog;
}
protected CatalogItemDo> getCatalogItemDo(String id) {
return catalog.getCache().get(id);
}
@Override
public CatalogItem> getCatalogItem(String id) {
if (id==null) return null;
CatalogItemDo> itemDo = getCatalogItemDo(id);
if (itemDo==null) return null;
return itemDo.getDto();
}
@SuppressWarnings("unchecked")
@Override
public CatalogItem getCatalogItem(Class type, String id) {
if (id==null) return null;
CatalogItem> result = getCatalogItem(id);
if (type==null || type.isAssignableFrom(result.getCatalogItemJavaType()))
return (CatalogItem)result;
return null;
}
public ClassLoader getRootClassLoader() {
return catalog.getRootClassLoader();
}
@SuppressWarnings("unchecked")
@Override
public Class extends T> loadClass(CatalogItem item) {
if (log.isDebugEnabled())
log.debug("Loading class for catalog item "+item);
Preconditions.checkNotNull(item);
CatalogItemDo> loadedItem = getCatalogItemDo(item.getId());
if (loadedItem==null) throw new NoSuchElementException("Unable to load '"+item.getId()+"' to instantiate it");
return (Class extends T>) loadedItem.getJavaClass();
}
@SuppressWarnings("unchecked")
@Override
public Class extends T> loadClassByType(String typeName, Class typeClass) {
Iterable> resultL = getCatalogItems(CatalogPredicates.javaType(Predicates.equalTo(typeName)));
if (Iterables.isEmpty(resultL)) throw new NoSuchElementException("Unable to find catalog item for type "+typeName);
CatalogItem
© 2015 - 2025 Weber Informatics LLC | Privacy Policy