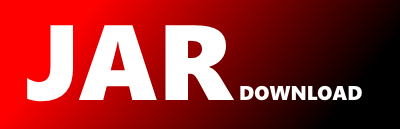
brooklyn.config.render.RendererHints Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.config.render;
import groovy.lang.Closure;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import brooklyn.entity.Entity;
import brooklyn.event.AttributeSensor;
import brooklyn.event.Sensor;
import brooklyn.util.GroovyJavaMethods;
import com.google.common.base.Function;
import com.google.common.base.Objects;
/** registry of hints for displaying items such as sensors, e.g. in the web console */
public class RendererHints {
static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy