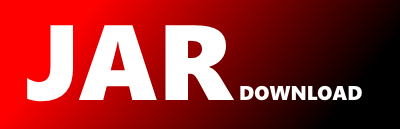
brooklyn.entity.basic.ConfigMapViewWithStringKeys Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.entity.basic;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
import brooklyn.config.ConfigKey;
import brooklyn.event.basic.BasicConfigKey;
import com.google.common.annotations.Beta;
import com.google.common.collect.Sets;
/**
* Internal class that presents a view over a ConfigMap, so it looks like a Map (with the
* keys being the config key names).
*/
@Beta
public class ConfigMapViewWithStringKeys implements Map {
private brooklyn.config.ConfigMap target;
public ConfigMapViewWithStringKeys(brooklyn.config.ConfigMap target) {
this.target = target;
}
@Override
public int size() {
return target.getAllConfig().size();
}
@Override
public boolean isEmpty() {
return target.getAllConfig().isEmpty();
}
@Override
public boolean containsKey(Object key) {
return keySet().contains(key);
}
@Override
public boolean containsValue(Object value) {
return values().contains(value);
}
@Override
public Object get(Object key) {
return target.getConfig(new BasicConfigKey
© 2015 - 2025 Weber Informatics LLC | Privacy Policy