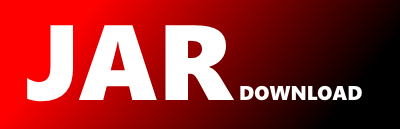
brooklyn.entity.basic.EntityPredicates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.entity.basic;
import javax.annotation.Nullable;
import brooklyn.entity.Entity;
import brooklyn.event.AttributeSensor;
import brooklyn.location.Location;
import com.google.common.base.Objects;
import com.google.common.base.Predicate;
public class EntityPredicates {
public static Predicate idEqualTo(final T val) {
return new Predicate() {
@Override
public boolean apply(@Nullable Entity input) {
return Objects.equal(input.getId(), val);
}
};
}
public static Predicate attributeEqualTo(final AttributeSensor attribute, final T val) {
return new Predicate() {
@Override
public boolean apply(@Nullable Entity input) {
return Objects.equal(input.getAttribute(attribute), val);
}
};
}
/**
* Create a predicate that matches any entity who has an exact match for the given location
* (i.e. {@code entity.getLocations().contains(location)}).
*/
public static Predicate withLocation(final Location location) {
return new Predicate() {
@Override
public boolean apply(@Nullable Entity input) {
return (input != null) && input.getLocations().contains(location);
}
};
}
public static Predicate managed() {
return new Predicate() {
@Override
public boolean apply(@Nullable Entity input) {
return input != null && Entities.isManaged(input);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy