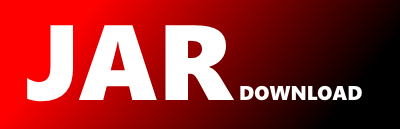
brooklyn.entity.group.DynamicCluster Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.entity.group;
import groovy.lang.Closure;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Set;
import brooklyn.config.ConfigKey;
import brooklyn.entity.Entity;
import brooklyn.entity.Group;
import brooklyn.entity.annotation.Effector;
import brooklyn.entity.annotation.EffectorParam;
import brooklyn.entity.basic.AbstractGroup;
import brooklyn.entity.basic.Attributes;
import brooklyn.entity.basic.ConfigKeys;
import brooklyn.entity.basic.EntityFactory;
import brooklyn.entity.basic.Lifecycle;
import brooklyn.entity.basic.MethodEffector;
import brooklyn.entity.group.zoneaware.BalancingNodePlacementStrategy;
import brooklyn.entity.group.zoneaware.ProportionalZoneFailureDetector;
import brooklyn.entity.proxying.EntitySpec;
import brooklyn.entity.proxying.ImplementedBy;
import brooklyn.event.AttributeSensor;
import brooklyn.event.basic.BasicAttributeSensor;
import brooklyn.event.basic.BasicConfigKey;
import brooklyn.event.basic.BasicNotificationSensor;
import brooklyn.location.Location;
import brooklyn.util.GroovyJavaMethods;
import brooklyn.util.flags.SetFromFlag;
import brooklyn.util.time.Duration;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Multimap;
/**
* A cluster of entities that can dynamically increase or decrease the number of entities.
*/
@ImplementedBy(DynamicClusterImpl.class)
public interface DynamicCluster extends AbstractGroup, Cluster {
@Beta
public interface NodePlacementStrategy {
List locationsForAdditions(Multimap currentMembers, Collection extends Location> locs, int numToAdd);
List entitiesToRemove(Multimap currentMembers, int numToRemove);
}
@Beta
public interface ZoneFailureDetector {
// TODO Would like to add entity-down reporting
// TODO Should we push any of this into the AvailabilityZoneExtension, rather than on the dynamic cluster?
void onStartupSuccess(Location loc, Entity entity);
void onStartupFailure(Location loc, Entity entity, Throwable cause);
boolean hasFailed(Location loc);
}
public static final MethodEffector REPLACE_MEMBER = new MethodEffector(DynamicCluster.class, "replaceMember");
@SetFromFlag("quarantineFailedEntities")
public static final ConfigKey QUARANTINE_FAILED_ENTITIES = new BasicConfigKey(
Boolean.class, "dynamiccluster.quarantineFailedEntities", "Whether to guarantine entities that fail to start, or to try to clean them up", false);
public static final AttributeSensor SERVICE_STATE = Attributes.SERVICE_STATE;
public static final BasicNotificationSensor ENTITY_QUARANTINED = new BasicNotificationSensor(Entity.class, "dynamiccluster.entityQuarantined", "Entity failed to start, and has been quarantined");
public static final AttributeSensor QUARANTINE_GROUP = new BasicAttributeSensor(Group.class, "dynamiccluster.quarantineGroup", "Group of quarantined entities that failed to start");
@SetFromFlag("initialQuorumSize")
ConfigKey INITIAL_QUORUM_SIZE = ConfigKeys.newIntegerConfigKey(
"cluster.initial.quorumSize",
"Initial cluster quorum size - number of initial nodes that must have been successfully started to report success (if < 0, then use value of INITIAL_SIZE)",
-1);
@SetFromFlag("memberSpec")
public static final ConfigKey> MEMBER_SPEC = new BasicConfigKey(
EntitySpec.class, "dynamiccluster.memberspec", "entity spec for creating new cluster members", null);
@SetFromFlag("factory")
public static final ConfigKey FACTORY = new BasicConfigKey(
EntityFactory.class, "dynamiccluster.factory", "factory for creating new cluster members", null);
@SetFromFlag("removalStrategy")
public static final ConfigKey, Entity>> REMOVAL_STRATEGY = new BasicConfigKey(
Function.class, "dynamiccluster.removalstrategy", "strategy for deciding what to remove when down-sizing", null);
@SetFromFlag("customChildFlags")
public static final ConfigKey
© 2015 - 2025 Weber Informatics LLC | Privacy Policy