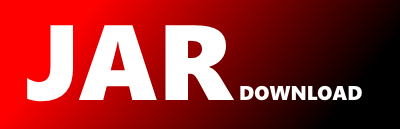
brooklyn.entity.rebind.dto.BasicLocationMemento Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.entity.rebind.dto;
import java.io.Serializable;
import java.util.Map;
import java.util.Set;
import brooklyn.mementos.LocationMemento;
import brooklyn.mementos.TreeNode;
import brooklyn.util.config.ConfigBag;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
/**
* The persisted state of a location.
*
* @author aled
*/
public class BasicLocationMemento extends AbstractTreeNodeMemento implements LocationMemento, Serializable {
private static final long serialVersionUID = -4025337943126838761L;
public static Builder builder() {
return new Builder();
}
public static class Builder extends AbstractTreeNodeMemento.Builder {
protected Map locationConfig = Maps.newLinkedHashMap();
protected Set locationConfigUnused = Sets.newLinkedHashSet();
protected String locationConfigDescription;
protected Set locationConfigReferenceKeys = Sets.newLinkedHashSet();
public Builder from(LocationMemento other) {
super.from((TreeNode)other);
displayName = other.getDisplayName();
locationConfig.putAll(other.getLocationConfig());
locationConfigUnused.addAll(other.getLocationConfigUnused());
locationConfigDescription = other.getLocationConfigDescription();
locationConfigReferenceKeys.addAll(other.getLocationConfigReferenceKeys());
fields.putAll(other.getCustomFields());
return self();
}
public LocationMemento build() {
return new BasicLocationMemento(this);
}
public void copyConfig(ConfigBag config) {
locationConfig.putAll(config.getAllConfig());
locationConfigUnused.addAll(config.getUnusedConfig().keySet());
locationConfigDescription = config.getDescription();
}
}
private Map locationConfig;
private Set locationConfigUnused;
private String locationConfigDescription;
private Set locationConfigReferenceKeys;
// Trusts the builder to not mess around with mutability after calling build()
protected BasicLocationMemento(Builder builder) {
super(builder);
locationConfig = toPersistedMap(builder.locationConfig);
locationConfigUnused = toPersistedSet(builder.locationConfigUnused);
locationConfigDescription = builder.locationConfigDescription;
locationConfigReferenceKeys = toPersistedSet(builder.locationConfigReferenceKeys);
}
@Override
public Map getLocationConfig() {
return fromPersistedMap(locationConfig);
}
@Override
public Set getLocationConfigUnused() {
return fromPersistedSet(locationConfigUnused);
}
@Override
public String getLocationConfigDescription() {
return locationConfigDescription;
}
@Override
public Set getLocationConfigReferenceKeys() {
return fromPersistedSet(locationConfigReferenceKeys);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy