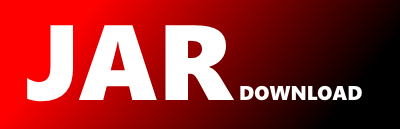
brooklyn.event.feed.http.HttpPollConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.event.feed.http;
import java.net.URI;
import java.util.Map;
import brooklyn.event.AttributeSensor;
import brooklyn.event.feed.FeedConfig;
import brooklyn.event.feed.PollConfig;
import brooklyn.util.collections.MutableMap;
import brooklyn.util.net.URLParamEncoder;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Predicate;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Iterables;
import javax.annotation.Nullable;
public class HttpPollConfig extends PollConfig> {
private String method = "GET";
private String suburl = "";
private Map vars = ImmutableMap.of();
private Map headers = ImmutableMap.of();
private byte[] body;
public static final Predicate DEFAULT_SUCCESS = new Predicate() {
@Override
public boolean apply(@Nullable HttpPollValue input) {
return input != null && input.getResponseCode() >= 200 && input.getResponseCode() <= 399;
}};
public HttpPollConfig(AttributeSensor sensor) {
super(sensor);
super.checkSuccess(DEFAULT_SUCCESS);
}
public HttpPollConfig(HttpPollConfig other) {
super(other);
suburl = other.suburl;
vars = other.vars;
method = other.method;
headers = other.headers;
}
public String getSuburl() {
return suburl;
}
public Map getVars() {
return vars;
}
public String getMethod() {
return method;
}
public byte[] getBody() {
return body;
}
public HttpPollConfig method(String val) {
this.method = val; return this;
}
public HttpPollConfig suburl(String val) {
this.suburl = val; return this;
}
public HttpPollConfig vars(Map val) {
this.vars = val; return this;
}
public HttpPollConfig headers(Map val) {
this.headers = val; return this;
}
public HttpPollConfig body(byte[] val) {
this.body = val; return this;
}
public URI buildUri(URI baseUri, Map baseUriVars) {
String uri = (baseUri != null ? baseUri.toString() : "") + (suburl != null ? suburl : "");
Map allvars = concat(baseUriVars, vars);
if (allvars != null && allvars.size() > 0) {
Iterable args = Iterables.transform(allvars.entrySet(),
new Function,String>() {
@Override public String apply(Map.Entry entry) {
String k = entry.getKey();
String v = entry.getValue();
return URLParamEncoder.encode(k) + (v != null ? "=" + URLParamEncoder.encode(v) : "");
}
});
uri += "?" + Joiner.on("&").join(args);
}
return URI.create(uri);
}
public Map buildHeaders(Map baseHeaders) {
return MutableMap.builder()
.putAll(baseHeaders)
.putAll(headers)
.build();
}
@SuppressWarnings("unchecked")
private Map concat(Map extends K,? extends V> map1, Map extends K,? extends V> map2) {
if (map1 == null || map1.isEmpty()) return (Map) map2;
if (map2 == null || map2.isEmpty()) return (Map) map1;
// TODO Not using Immutable builder, because that fails if duplicates in map1 and map2
return MutableMap.builder().putAll(map1).putAll(map2).build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy