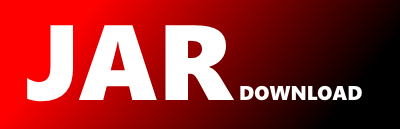
brooklyn.location.basic.LocalhostMachineProvisioningLocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.location.basic;
import static brooklyn.util.GroovyJavaMethods.elvis;
import static brooklyn.util.GroovyJavaMethods.truth;
import java.net.InetAddress;
import java.util.Arrays;
import java.util.Map;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import brooklyn.location.AddressableLocation;
import brooklyn.location.LocationSpec;
import brooklyn.location.OsDetails;
import brooklyn.location.PortRange;
import brooklyn.location.geo.HostGeoInfo;
import brooklyn.util.BrooklynNetworkUtils;
import brooklyn.util.collections.MutableMap;
import brooklyn.util.flags.SetFromFlag;
import brooklyn.util.internal.ssh.process.ProcessTool;
import brooklyn.util.mutex.MutexSupport;
import brooklyn.util.mutex.WithMutexes;
import brooklyn.util.net.Networking;
import brooklyn.util.ssh.BashCommands;
import brooklyn.util.time.Duration;
import brooklyn.util.time.Time;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
/**
* An implementation of {@link brooklyn.location.MachineProvisioningLocation} that can provision a {@link SshMachineLocation} for the
* local host.
*
* By default you can only obtain a single SshMachineLocation for the localhost. Optionally, you can "overload"
* and choose to allow localhost to be provisioned multiple times, which may be useful in some testing scenarios.
*/
public class LocalhostMachineProvisioningLocation extends FixedListMachineProvisioningLocation implements AddressableLocation {
public static final Logger LOG = LoggerFactory.getLogger(LocalhostMachineProvisioningLocation.class);
@SetFromFlag("count")
int initialCount;
@SetFromFlag
Boolean canProvisionMore;
@SetFromFlag
InetAddress address;
private static Set portsInUse = Sets.newLinkedHashSet();
private static HostGeoInfo cachedHostGeoInfo;
/**
* Construct a new instance.
*
* The constructor recognises the following properties:
*
* - count - number of localhost machines to make available
*
*/
public LocalhostMachineProvisioningLocation() {
this(Maps.newLinkedHashMap());
}
/**
* @param properties the properties of the new instance.
* @deprecated since 0.6
* @see #LocalhostMachineProvisioningLocation()
*/
public LocalhostMachineProvisioningLocation(Map properties) {
super(properties);
}
public LocalhostMachineProvisioningLocation(String name) {
this(name, 0);
}
public LocalhostMachineProvisioningLocation(String name, int count) {
this(MutableMap.of("name", name, "count", count));
}
public static LocationSpec spec() {
return LocationSpec.create(LocalhostMachineProvisioningLocation.class);
}
public void configure(Map flags) {
super.configure(flags);
if (!truth(name)) { name = "localhost"; }
if (!truth(address)) address = getLocalhostInetAddress();
// TODO should try to confirm this machine is accessible on the given address ... but there's no
// immediate convenience in java so early-trapping of that particular error is deferred
if (canProvisionMore==null) {
if (initialCount>0) canProvisionMore = false;
else canProvisionMore = true;
}
if (getHostGeoInfo()==null) {
if (cachedHostGeoInfo==null)
cachedHostGeoInfo = HostGeoInfo.fromLocation(this);
setHostGeoInfo(cachedHostGeoInfo);
}
if (initialCount > getMachines().size()) {
provisionMore(initialCount - getMachines().size());
}
}
public static InetAddress getLocalhostInetAddress() {
return BrooklynNetworkUtils.getLocalhostInetAddress();
}
@Override
public InetAddress getAddress() {
return address;
}
@Override
public boolean canProvisionMore() {
return canProvisionMore;
}
@Override
protected void provisionMore(int size, Map,?> flags) {
for (int i=0; i flags2 = MutableMap.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy