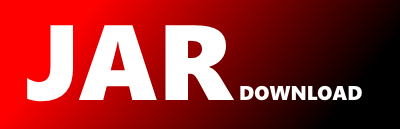
brooklyn.location.cloud.AbstractAvailabilityZoneExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.location.cloud;
import static com.google.common.base.Preconditions.checkNotNull;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.atomic.AtomicReference;
import brooklyn.location.Location;
import brooklyn.management.ManagementContext;
import com.google.common.annotations.Beta;
import com.google.common.base.Predicate;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
@Beta
public abstract class AbstractAvailabilityZoneExtension implements AvailabilityZoneExtension {
protected final ManagementContext managementContext;
protected final AtomicReference> subLocations = new AtomicReference>();
private final Object mutex = new Object();
public AbstractAvailabilityZoneExtension(ManagementContext managementContext) {
this.managementContext = checkNotNull(managementContext, "managementContext");
}
@Override
public List getSubLocations(int max) {
List all = getAllSubLocations();
return all.subList(0, Math.min(max, all.size()));
}
@Override
public List getSubLocationsByName(Predicate super String> namePredicate, int max) {
List result = Lists.newArrayList();
List all = getAllSubLocations();
for (Location loc : all) {
if (isNameMatch(loc, namePredicate)) {
result.add(loc);
}
}
return Collections.unmodifiableList(result);
}
protected List getAllSubLocations() {
synchronized (mutex) {
if (subLocations.get() == null) {
List result = doGetAllSubLocations();
subLocations.set(ImmutableList.copyOf(result));
}
}
return subLocations.get();
}
/**
* Note called while holding mutex, so do not call code that will result in deadlock!
* TODO: bad pattern, as this will likely call alien code (such as asking cloud provider?!)
*/
protected abstract List doGetAllSubLocations();
protected abstract boolean isNameMatch(Location loc, Predicate super String> namePredicate);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy