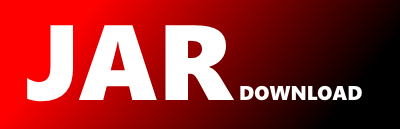
brooklyn.management.internal.QueueingSubscriptionManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.management.internal;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import brooklyn.entity.Entity;
import brooklyn.event.Sensor;
import brooklyn.event.SensorEvent;
import brooklyn.management.SubscriptionHandle;
import com.google.common.base.Objects;
@SuppressWarnings("rawtypes")
public class QueueingSubscriptionManager extends AbstractSubscriptionManager {
static class QueuedSubscription {
Map flags;
Subscription s;
}
public AbstractSubscriptionManager delegate = null;
public boolean useDelegateForSubscribing = false;
public boolean useDelegateForPublishing = false;
List queuedSubscriptions = new ArrayList();
List queuedSensorEvents = new ArrayList();
@Override
protected synchronized SubscriptionHandle subscribe(Map flags, Subscription s) {
if (useDelegateForSubscribing)
return delegate.subscribe(flags, s);
QueuedSubscription qs = new QueuedSubscription();
qs.flags = flags;
s.subscriber = getSubscriber(flags, s);
qs.s = s;
queuedSubscriptions.add(qs);
return s;
}
@Override
public synchronized void publish(SensorEvent event) {
if (useDelegateForPublishing) {
delegate.publish(event);
return;
}
queuedSensorEvents.add(event);
}
public void setDelegate(AbstractSubscriptionManager delegate) {
this.delegate = delegate;
}
@SuppressWarnings("unchecked")
public synchronized void startDelegatingForSubscribing() {
assert delegate!=null;
for (QueuedSubscription s: queuedSubscriptions) {
delegate.subscribe(s.flags, s.s);
}
queuedSubscriptions.clear();
useDelegateForSubscribing = true;
}
@SuppressWarnings("unchecked")
public synchronized void startDelegatingForPublishing() {
assert delegate!=null;
for (SensorEvent evt: queuedSensorEvents) {
delegate.publish(evt);
}
queuedSensorEvents.clear();
useDelegateForPublishing = true;
}
public synchronized void stopDelegatingForSubscribing() {
useDelegateForSubscribing = false;
}
public synchronized void stopDelegatingForPublishing() {
useDelegateForPublishing = false;
}
@Override
public synchronized boolean unsubscribe(SubscriptionHandle subscriptionId) {
if (useDelegateForSubscribing)
return delegate.unsubscribe(subscriptionId);
Iterator qi = queuedSubscriptions.iterator();
while (qi.hasNext()) {
QueuedSubscription q = qi.next();
if (Objects.equal(subscriptionId, q.s)) {
qi.remove();
return true;
}
}
return false;
}
@SuppressWarnings("unchecked")
@Override
public synchronized Set getSubscriptionsForSubscriber(Object subscriber) {
if (useDelegateForSubscribing)
return delegate.getSubscriptionsForSubscriber(subscriber);
Set result = new LinkedHashSet();
for (QueuedSubscription q: queuedSubscriptions) {
if (Objects.equal(subscriber, getSubscriber(q.flags, q.s))) result.add(q.s);
}
return result;
}
@Override
public synchronized Set getSubscriptionsForEntitySensor(Entity source, Sensor> sensor) {
if (useDelegateForSubscribing)
return delegate.getSubscriptionsForEntitySensor(source, sensor);
Set result = new LinkedHashSet();
for (QueuedSubscription q: queuedSubscriptions) {
if ((q.s.sensor==null || Objects.equal(q.s.sensor, sensor)) &&
(q.s.producer==null || Objects.equal(q.s.producer, sensor)))
result.add(q.s);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy