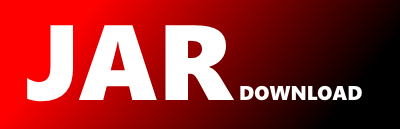
brooklyn.util.task.TaskBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-core Show documentation
Show all versions of brooklyn-core Show documentation
Entity implementation classes, events, and other core elements
package brooklyn.util.task;
import java.util.ArrayList;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import java.util.concurrent.Callable;
import brooklyn.management.Task;
import brooklyn.management.TaskFactory;
import brooklyn.management.TaskQueueingContext;
import brooklyn.util.JavaGroovyEquivalents;
import brooklyn.util.collections.MutableMap;
/** Convenience for creating tasks; note that DynamicSequentialTask is the default */
public class TaskBuilder {
String name = null;
Callable body = null;
List> children = new ArrayList>();
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy