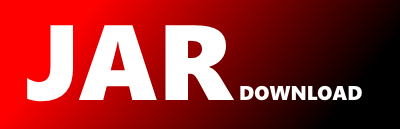
brooklyn.rest.domain.ConfigSummary Maven / Gradle / Ivy
The newest version!
package brooklyn.rest.domain;
import java.net.URI;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import javax.annotation.Nullable;
import org.codehaus.jackson.annotate.JsonProperty;
import org.codehaus.jackson.map.annotate.JsonSerialize;
import org.codehaus.jackson.map.annotate.JsonSerialize.Inclusion;
import brooklyn.config.ConfigKey;
import brooklyn.util.collections.Jsonya;
import com.google.common.base.Function;
import com.google.common.collect.FluentIterable;
import com.google.common.collect.ImmutableMap;
public abstract class ConfigSummary {
private final String name;
private final String type;
@JsonSerialize(include=Inclusion.NON_NULL)
private final Object defaultValue;
@JsonSerialize(include=Inclusion.NON_NULL)
private final String description;
@JsonSerialize
private final boolean reconfigurable;
@JsonSerialize(include=Inclusion.NON_NULL)
private final String label;
@JsonSerialize(include=Inclusion.NON_NULL)
private final Double priority;
@JsonSerialize(include=Inclusion.NON_NULL)
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy