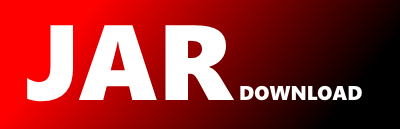
io.camunda.connector.slack.utils.SlackRequestDeserializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connector-slack Show documentation
Show all versions of connector-slack Show documentation
Camunda Cloud Slack Connector
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH
* under one or more contributor license agreements. Licensed under a proprietary license.
* See the License.txt file for more information. You may not use this file
* except in compliance with the proprietary license.
*/
package io.camunda.connector.slack.utils;
import com.google.gson.*;
import com.google.gson.reflect.TypeToken;
import io.camunda.connector.slack.SlackRequest;
import io.camunda.connector.slack.SlackRequestData;
import java.lang.reflect.Type;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
public class SlackRequestDeserializer
implements JsonDeserializer> {
private String typeElementName;
private Gson gson;
private Map> typeRegistry;
public SlackRequestDeserializer(String typeElementName) {
this.typeElementName = typeElementName;
this.gson = new Gson();
this.typeRegistry = new HashMap<>();
}
public SlackRequestDeserializer registerType(
String typeName, Class extends SlackRequestData> requestType) {
typeRegistry.put(typeName, requestType);
return this;
}
@Override
public SlackRequest extends SlackRequestData> deserialize(
JsonElement jsonElement, Type type, JsonDeserializationContext jsonDeserializationContext)
throws JsonParseException {
return getTypeElementValue(jsonElement)
.map(typeRegistry::get)
.map(SlackRequestDeserializer::getTypeToken)
.map(typeToken -> getSlackRequest(jsonElement, typeToken))
.orElse(null);
}
private Optional getTypeElementValue(JsonElement jsonElement) {
JsonObject asJsonObject = jsonElement.getAsJsonObject();
JsonElement element = asJsonObject.get(typeElementName);
return Optional.ofNullable(element).map(JsonElement::getAsString);
}
private static TypeToken> getTypeToken(
Class extends SlackRequestData> requestDataClass) {
return (TypeToken>)
TypeToken.getParameterized(SlackRequest.class, requestDataClass);
}
private SlackRequest extends SlackRequestData> getSlackRequest(
JsonElement jsonElement, TypeToken> typeToken) {
return gson.fromJson(jsonElement, typeToken.getType());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy