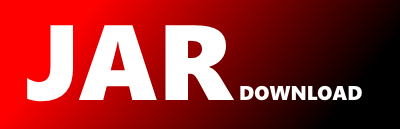
io.camunda.exporter.handlers.TenantCreateUpdateHandler Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Camunda License 1.0. You may not use this file
* except in compliance with the Camunda License 1.0.
*/
package io.camunda.exporter.handlers;
import io.camunda.exporter.exceptions.PersistenceException;
import io.camunda.exporter.store.BatchRequest;
import io.camunda.webapps.schema.entities.usermanagement.TenantEntity;
import io.camunda.zeebe.protocol.record.Record;
import io.camunda.zeebe.protocol.record.ValueType;
import io.camunda.zeebe.protocol.record.intent.Intent;
import io.camunda.zeebe.protocol.record.intent.TenantIntent;
import io.camunda.zeebe.protocol.record.value.TenantRecordValue;
import java.util.List;
import java.util.Set;
public class TenantCreateUpdateHandler implements ExportHandler {
private static final Set SUPPORTED_INTENTS =
Set.of(TenantIntent.CREATED, TenantIntent.UPDATED);
private final String indexName;
public TenantCreateUpdateHandler(final String indexName) {
this.indexName = indexName;
}
@Override
public ValueType getHandledValueType() {
return ValueType.TENANT;
}
@Override
public Class getEntityType() {
return TenantEntity.class;
}
@Override
public boolean handlesRecord(final Record record) {
return getHandledValueType().equals(record.getValueType())
&& SUPPORTED_INTENTS.contains(record.getIntent());
}
@Override
public List generateIds(final Record record) {
return List.of(String.valueOf(record.getKey()));
}
@Override
public TenantEntity createNewEntity(final String id) {
return new TenantEntity().setId(id);
}
@Override
public void updateEntity(final Record record, final TenantEntity entity) {
final TenantRecordValue value = record.getValue();
entity.setKey(value.getTenantKey()).setTenantId(value.getTenantId()).setName(value.getName());
}
@Override
public void flush(final TenantEntity entity, final BatchRequest batchRequest)
throws PersistenceException {
batchRequest.add(indexName, entity);
}
@Override
public String getIndexName() {
return indexName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy