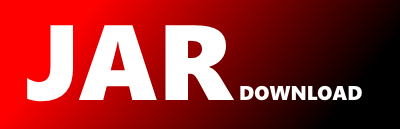
io.camunda.exporter.schema.IndexMapping Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Camunda License 1.0. You may not use this file
* except in compliance with the Camunda License 1.0.
*/
package io.camunda.exporter.schema;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.camunda.webapps.schema.descriptors.IndexDescriptor;
import java.io.IOException;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
public record IndexMapping(
String indexName,
String dynamic,
Set properties,
Map metaProperties) {
public Map toMap() {
return properties.stream()
.collect(
Collectors.toMap(IndexMappingProperty::name, IndexMappingProperty::typeDefinition));
}
public boolean isDynamic() {
return Boolean.parseBoolean(dynamic);
}
@SuppressWarnings("unchecked")
public static IndexMapping from(
final IndexDescriptor indexDescriptor, final ObjectMapper mapper) {
try (final var mappingsStream =
SchemaManager.class.getResourceAsStream(indexDescriptor.getMappingsClasspathFilename())) {
final var nestedType = new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy