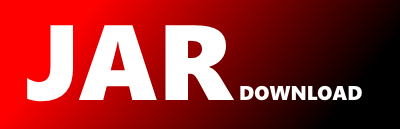
io.camunda.exporter.utils.SearchEngineClientUtils Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Camunda License 1.0. You may not use this file
* except in compliance with the Camunda License 1.0.
*/
package io.camunda.exporter.utils;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.camunda.exporter.config.ExporterConfiguration.IndexSettings;
import io.camunda.webapps.schema.descriptors.IndexDescriptor;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
import org.apache.commons.io.IOUtils;
public final class SearchEngineClientUtils {
private static final ObjectMapper MAPPER = new ObjectMapper();
private SearchEngineClientUtils() {}
public static InputStream appendToFileSchemaSettings(
final InputStream file, final IndexSettings settingsToAppend) throws IOException {
final var map = MAPPER.readValue(file, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy