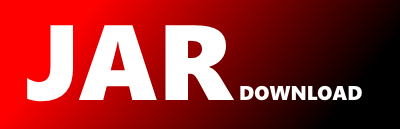
io.camunda.util.CollectionUtil Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Camunda License 1.0. You may not use this file
* except in compliance with the Camunda License 1.0.
*/
package io.camunda.util;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
public final class CollectionUtil {
private CollectionUtil() {}
public static List withoutNull(final A[] items) {
if (items != null) {
return withoutNull(Arrays.asList(items));
}
return null;
}
public static List withoutNull(final Collection items) {
if (items != null) {
return items.stream().filter(Objects::nonNull).collect(Collectors.toList());
}
return null;
}
/**
* Adds given values to a list.
*
* This method can handle null-values gracefully.
*
*
This means, if either one of the given list is empty, and empty ArrayList will be used
* instead.
*
*
Example:
*
*
* addValuesToList(null, List.of(1)) -> List(1) * addValuesToList(List.of(1), null) ->
* List(1) * addValuesToList(null, null) -> List() * addValuesToList(List.of(1), List.of(2)) ->
* List(1, 2)
*
* @param list where the values should be added to
* @param values the values which should be added
* @return the given list (when not empty) otherwise a new list containing the values
* @param the list value type
*/
public static List addValuesToList(final List list, final List values) {
final List result = Objects.requireNonNullElse(list, new ArrayList<>());
result.addAll(Objects.requireNonNullElse(values, new ArrayList<>()));
return result;
}
/**
* Collects a given values (array) as list, and handles potential null or empty values.
*
* @param values the values that needs to be collected
* @return an appropriate list containing the values
* @param the type of the values
*/
public static List collectValuesAsList(final T... values) {
if (values == null) {
return List.of();
}
return Arrays.stream(values).toList();
}
public static List collectValues(final T value, final T... values) {
final List collectedValues = new ArrayList<>();
collectedValues.add(value);
if (values != null && values.length > 0) {
collectedValues.addAll(Arrays.asList(values));
}
return collectedValues;
}
}