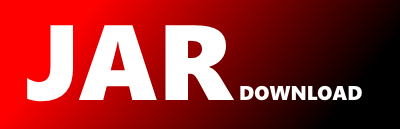
io.camunda.service.ApiServices Maven / Gradle / Ivy
The newest version!
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Camunda License 1.0. You may not use this file
* except in compliance with the Camunda License 1.0.
*/
package io.camunda.service;
import io.camunda.security.auth.Authentication;
import io.camunda.security.configuration.SecurityConfiguration;
import io.camunda.service.exception.CamundaBrokerException;
import io.camunda.zeebe.broker.client.api.BrokerClient;
import io.camunda.zeebe.broker.client.api.dto.BrokerRequest;
import io.camunda.zeebe.broker.client.api.dto.BrokerResponse;
import io.camunda.zeebe.msgpack.value.DocumentValue;
import io.camunda.zeebe.protocol.impl.encoding.MsgPackConverter;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import org.agrona.DirectBuffer;
import org.agrona.concurrent.UnsafeBuffer;
public abstract class ApiServices> {
protected final BrokerClient brokerClient;
protected final SecurityConfiguration securityConfiguration;
protected final Authentication authentication;
protected ApiServices(
final BrokerClient brokerClient,
final SecurityConfiguration securityConfiguration,
final Authentication authentication) {
this.brokerClient = brokerClient;
this.securityConfiguration = securityConfiguration;
this.authentication = authentication;
}
public abstract T withAuthentication(final Authentication authentication);
public T withAuthentication(final Function fn) {
return withAuthentication(fn.apply(new Authentication.Builder()).build());
}
protected CompletableFuture sendBrokerRequest(final BrokerRequest brokerRequest) {
return sendBrokerRequestWithFullResponse(brokerRequest).thenApply(BrokerResponse::getResponse);
}
protected CompletableFuture> sendBrokerRequestWithFullResponse(
final BrokerRequest brokerRequest) {
brokerRequest.setAuthorization(authentication.token());
return brokerClient
.sendRequest(brokerRequest)
.handleAsync(
(response, error) -> {
if (error != null) {
throw new CamundaBrokerException(error);
}
if (response.isError()) {
throw new CamundaBrokerException(response.getError());
}
if (response.isRejection()) {
throw new CamundaBrokerException(response.getRejection());
}
return response;
});
}
protected DirectBuffer getDocumentOrEmpty(final Map value) {
return value == null || value.isEmpty()
? DocumentValue.EMPTY_DOCUMENT
: new UnsafeBuffer(MsgPackConverter.convertToMsgPack(value));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy