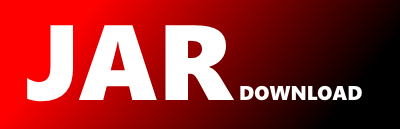
io.camunda.tasklist.generated.model.TaskAssignRequest Maven / Gradle / Ivy
/*
* Tasklist REST API
* Tasklist is a ready-to-use API application to rapidly implement business processes alongside user tasks in Zeebe.
*
* The version of the OpenAPI document: v1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.camunda.tasklist.generated.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* Request params used to assign the task to assignee or current user.
*/
@JsonPropertyOrder({
TaskAssignRequest.JSON_PROPERTY_ASSIGNEE,
TaskAssignRequest.JSON_PROPERTY_ALLOW_OVERRIDE_ASSIGNMENT
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-28T19:05:36.804206600Z[Etc/UTC]", comments = "Generator version: 7.8.0")
public class TaskAssignRequest {
public static final String JSON_PROPERTY_ASSIGNEE = "assignee";
private String assignee;
public static final String JSON_PROPERTY_ALLOW_OVERRIDE_ASSIGNMENT = "allowOverrideAssignment";
private Boolean allowOverrideAssignment = true;
public TaskAssignRequest() {
}
public TaskAssignRequest assignee(String assignee) {
this.assignee = assignee;
return this;
}
/**
* When using a JWT token, the assignee parameter is NOT optional when called directly from the API. The system will not be able to detect the assignee from the JWT token, therefore the assignee parameter needs to be explicitly passed in this instance.
* @return assignee
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ASSIGNEE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAssignee() {
return assignee;
}
@JsonProperty(JSON_PROPERTY_ASSIGNEE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAssignee(String assignee) {
this.assignee = assignee;
}
public TaskAssignRequest allowOverrideAssignment(Boolean allowOverrideAssignment) {
this.allowOverrideAssignment = allowOverrideAssignment;
return this;
}
/**
* When `true` the task that is already assigned may be assigned again. Otherwise the task must be first unassigned and only then assigned again.
* @return allowOverrideAssignment
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ALLOW_OVERRIDE_ASSIGNMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAllowOverrideAssignment() {
return allowOverrideAssignment;
}
@JsonProperty(JSON_PROPERTY_ALLOW_OVERRIDE_ASSIGNMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAllowOverrideAssignment(Boolean allowOverrideAssignment) {
this.allowOverrideAssignment = allowOverrideAssignment;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskAssignRequest taskAssignRequest = (TaskAssignRequest) o;
return Objects.equals(this.assignee, taskAssignRequest.assignee) &&
Objects.equals(this.allowOverrideAssignment, taskAssignRequest.allowOverrideAssignment);
}
@Override
public int hashCode() {
return Objects.hash(assignee, allowOverrideAssignment);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskAssignRequest {\n");
sb.append(" assignee: ").append(toIndentedString(assignee)).append("\n");
sb.append(" allowOverrideAssignment: ").append(toIndentedString(allowOverrideAssignment)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `assignee` to the URL query string
if (getAssignee() != null) {
try {
joiner.add(String.format("%sassignee%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAssignee()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `allowOverrideAssignment` to the URL query string
if (getAllowOverrideAssignment() != null) {
try {
joiner.add(String.format("%sallowOverrideAssignment%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAllowOverrideAssignment()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy