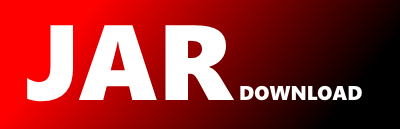
io.camunda.tasklist.generated.api.TaskApi Maven / Gradle / Ivy
/*
* Tasklist REST API
* Tasklist is a ready-to-use API application to rapidly implement business processes alongside user tasks in Zeebe.
*
* The version of the OpenAPI document: v1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.camunda.tasklist.generated.api;
import com.fasterxml.jackson.core.type.TypeReference;
import io.camunda.tasklist.generated.invoker.ApiException;
import io.camunda.tasklist.generated.invoker.ApiClient;
import io.camunda.tasklist.generated.invoker.BaseApi;
import io.camunda.tasklist.generated.invoker.Configuration;
import io.camunda.tasklist.generated.invoker.Pair;
import io.camunda.tasklist.generated.model.Error;
import io.camunda.tasklist.generated.model.SaveVariablesRequest;
import io.camunda.tasklist.generated.model.TaskAssignRequest;
import io.camunda.tasklist.generated.model.TaskCompleteRequest;
import io.camunda.tasklist.generated.model.TaskResponse;
import io.camunda.tasklist.generated.model.TaskSearchRequest;
import io.camunda.tasklist.generated.model.TaskSearchResponse;
import io.camunda.tasklist.generated.model.VariableSearchResponse;
import io.camunda.tasklist.generated.model.VariablesSearchRequest;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-29T10:52:23.981575172Z[Etc/UTC]", comments = "Generator version: 7.8.0")
public class TaskApi extends BaseApi {
public TaskApi() {
super(Configuration.getDefaultApiClient());
}
public TaskApi(ApiClient apiClient) {
super(apiClient);
}
/**
* Assign a task
* Assign a task with `taskId` to `assignee` or the active user. Returns the task.
* @param taskId The ID of the task. (required)
* @param taskAssignRequest When using REST API with JWT authentication token following request body parameters may be used. (optional)
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse assignTask(String taskId, TaskAssignRequest taskAssignRequest) throws ApiException {
return this.assignTask(taskId, taskAssignRequest, Collections.emptyMap());
}
/**
* Assign a task
* Assign a task with `taskId` to `assignee` or the active user. Returns the task.
* @param taskId The ID of the task. (required)
* @param taskAssignRequest When using REST API with JWT authentication token following request body parameters may be used. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse assignTask(String taskId, TaskAssignRequest taskAssignRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = taskAssignRequest;
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException(400, "Missing the required parameter 'taskId' when calling assignTask");
}
// create path and map variables
String localVarPath = "/v1/tasks/{taskId}/assign"
.replaceAll("\\{" + "taskId" + "\\}", apiClient.escapeString(taskId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"PATCH",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Complete a task
* Complete a task with `taskId` and optional `variables`. Returns the task.
* @param taskId The ID of the task. (required)
* @param taskCompleteRequest (optional)
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse completeTask(String taskId, TaskCompleteRequest taskCompleteRequest) throws ApiException {
return this.completeTask(taskId, taskCompleteRequest, Collections.emptyMap());
}
/**
* Complete a task
* Complete a task with `taskId` and optional `variables`. Returns the task.
* @param taskId The ID of the task. (required)
* @param taskCompleteRequest (optional)
* @param additionalHeaders additionalHeaders for this call
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse completeTask(String taskId, TaskCompleteRequest taskCompleteRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = taskCompleteRequest;
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException(400, "Missing the required parameter 'taskId' when calling completeTask");
}
// create path and map variables
String localVarPath = "/v1/tasks/{taskId}/complete"
.replaceAll("\\{" + "taskId" + "\\}", apiClient.escapeString(taskId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"PATCH",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Get a task
* Get one task by id. Returns task or error when task does not exist.
* @param taskId The ID of the task. (required)
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse getTaskById(String taskId) throws ApiException {
return this.getTaskById(taskId, Collections.emptyMap());
}
/**
* Get a task
* Get one task by id. Returns task or error when task does not exist.
* @param taskId The ID of the task. (required)
* @param additionalHeaders additionalHeaders for this call
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse getTaskById(String taskId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException(400, "Missing the required parameter 'taskId' when calling getTaskById");
}
// create path and map variables
String localVarPath = "/v1/tasks/{taskId}"
.replaceAll("\\{" + "taskId" + "\\}", apiClient.escapeString(taskId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Save draft variables
* This operation performs several actions: <br/><ol><li>Validates the task and draft variables.</li><li>Deletes existing draft variables for the task.</li><li>Checks for new draft variables. If a new variable's `name` matches an existing one but the `value` differs, it is saved. In case of duplicate draft variable names, the last variable's value is kept.</li></ol><b>NOTE:</b><ul><li>Invoking this method successively will overwrite all existing draft variables. Only draft variables submitted in the most recent request body will be persisted. Therefore, ensure you include all necessary variables in each request to maintain the intended variable set.</li><li>The UI does not currently display the values for draft variables that are created via this endpoint.</li></ul>
* @param taskId The ID of the task. (required)
* @param saveVariablesRequest (required)
* @throws ApiException if fails to make API call
*/
public void saveDraftTaskVariables(String taskId, SaveVariablesRequest saveVariablesRequest) throws ApiException {
this.saveDraftTaskVariables(taskId, saveVariablesRequest, Collections.emptyMap());
}
/**
* Save draft variables
* This operation performs several actions: <br/><ol><li>Validates the task and draft variables.</li><li>Deletes existing draft variables for the task.</li><li>Checks for new draft variables. If a new variable's `name` matches an existing one but the `value` differs, it is saved. In case of duplicate draft variable names, the last variable's value is kept.</li></ol><b>NOTE:</b><ul><li>Invoking this method successively will overwrite all existing draft variables. Only draft variables submitted in the most recent request body will be persisted. Therefore, ensure you include all necessary variables in each request to maintain the intended variable set.</li><li>The UI does not currently display the values for draft variables that are created via this endpoint.</li></ul>
* @param taskId The ID of the task. (required)
* @param saveVariablesRequest (required)
* @param additionalHeaders additionalHeaders for this call
* @throws ApiException if fails to make API call
*/
public void saveDraftTaskVariables(String taskId, SaveVariablesRequest saveVariablesRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = saveVariablesRequest;
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException(400, "Missing the required parameter 'taskId' when calling saveDraftTaskVariables");
}
// verify the required parameter 'saveVariablesRequest' is set
if (saveVariablesRequest == null) {
throw new ApiException(400, "Missing the required parameter 'saveVariablesRequest' when calling saveDraftTaskVariables");
}
// create path and map variables
String localVarPath = "/v1/tasks/{taskId}/variables"
.replaceAll("\\{" + "taskId" + "\\}", apiClient.escapeString(taskId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"*/*", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
null
);
}
/**
* Search task variables
* This method returns a list of task variables for the specified `taskId` and `variableName`.<br>If the request body is not provided or if the `variableNames` parameter in the request is empty, all variables associated with the task will be returned.
* @param taskId The ID of the task. (required)
* @param variablesSearchRequest (optional)
* @return List<VariableSearchResponse>
* @throws ApiException if fails to make API call
*/
public List searchTaskVariables(String taskId, VariablesSearchRequest variablesSearchRequest) throws ApiException {
return this.searchTaskVariables(taskId, variablesSearchRequest, Collections.emptyMap());
}
/**
* Search task variables
* This method returns a list of task variables for the specified `taskId` and `variableName`.<br>If the request body is not provided or if the `variableNames` parameter in the request is empty, all variables associated with the task will be returned.
* @param taskId The ID of the task. (required)
* @param variablesSearchRequest (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<VariableSearchResponse>
* @throws ApiException if fails to make API call
*/
public List searchTaskVariables(String taskId, VariablesSearchRequest variablesSearchRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = variablesSearchRequest;
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException(400, "Missing the required parameter 'taskId' when calling searchTaskVariables");
}
// create path and map variables
String localVarPath = "/v1/tasks/{taskId}/variables/search"
.replaceAll("\\{" + "taskId" + "\\}", apiClient.escapeString(taskId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Search tasks
* Returns the list of tasks that satisfy search request params.<br><ul><li>If an empty body is provided, all tasks are returned.</li><li>Only one of `[searchAfter, searchAfterOrEqual, searchBefore, searchBeforeOrEqual]` search options must be present in request.</li></ul>
* @param taskSearchRequest (optional)
* @return List<TaskSearchResponse>
* @throws ApiException if fails to make API call
*/
public List searchTasks(TaskSearchRequest taskSearchRequest) throws ApiException {
return this.searchTasks(taskSearchRequest, Collections.emptyMap());
}
/**
* Search tasks
* Returns the list of tasks that satisfy search request params.<br><ul><li>If an empty body is provided, all tasks are returned.</li><li>Only one of `[searchAfter, searchAfterOrEqual, searchBefore, searchBeforeOrEqual]` search options must be present in request.</li></ul>
* @param taskSearchRequest (optional)
* @param additionalHeaders additionalHeaders for this call
* @return List<TaskSearchResponse>
* @throws ApiException if fails to make API call
*/
public List searchTasks(TaskSearchRequest taskSearchRequest, Map additionalHeaders) throws ApiException {
Object localVarPostBody = taskSearchRequest;
// create path and map variables
String localVarPath = "/v1/tasks/search";
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
TypeReference> localVarReturnType = new TypeReference>() {};
return apiClient.invokeAPI(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
/**
* Unassign a task
* Unassign a task with `taskId`. Returns the task.
* @param taskId The ID of the task. (required)
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse unassignTask(String taskId) throws ApiException {
return this.unassignTask(taskId, Collections.emptyMap());
}
/**
* Unassign a task
* Unassign a task with `taskId`. Returns the task.
* @param taskId The ID of the task. (required)
* @param additionalHeaders additionalHeaders for this call
* @return TaskResponse
* @throws ApiException if fails to make API call
*/
public TaskResponse unassignTask(String taskId, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException(400, "Missing the required parameter 'taskId' when calling unassignTask");
}
// create path and map variables
String localVarPath = "/v1/tasks/{taskId}/unassign"
.replaceAll("\\{" + "taskId" + "\\}", apiClient.escapeString(taskId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"PATCH",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
@Override
public T invokeAPI(String url, String method, Object request, TypeReference returnType, Map additionalHeaders) throws ApiException {
String localVarPath = url.replace(apiClient.getBaseURL(), "");
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
return apiClient.invokeAPI(
localVarPath,
method,
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
request,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
returnType
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy