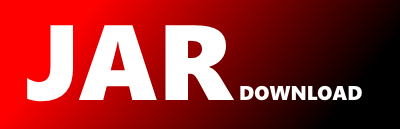
io.camunda.tasklist.generated.api.FormApi Maven / Gradle / Ivy
The newest version!
/*
* Tasklist REST API
* Tasklist is a ready-to-use API application to rapidly implement business processes alongside user tasks in Zeebe.
*
* The version of the OpenAPI document: v1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.camunda.tasklist.generated.api;
import com.fasterxml.jackson.core.type.TypeReference;
import io.camunda.tasklist.generated.invoker.ApiException;
import io.camunda.tasklist.generated.invoker.ApiClient;
import io.camunda.tasklist.generated.invoker.BaseApi;
import io.camunda.tasklist.generated.invoker.Configuration;
import io.camunda.tasklist.generated.invoker.Pair;
import io.camunda.tasklist.generated.model.Error;
import io.camunda.tasklist.generated.model.FormResponse;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-10T08:38:14.250454645Z[Etc/UTC]", comments = "Generator version: 7.8.0")
public class FormApi extends BaseApi {
public FormApi() {
super(Configuration.getDefaultApiClient());
}
public FormApi(ApiClient apiClient) {
super(apiClient);
}
/**
* Get a form
* Get the form details by `formId` and `processDefinitionKey` required query param. The `version` query param is optional and is used only for deployed forms (if empty, it retrieves the highest version).
* @param formId The ID of the form. (required)
* @param processDefinitionKey Reference to the process definition. (required)
* @param version The version of the form. Valid only for deployed forms. (optional)
* @return FormResponse
* @throws ApiException if fails to make API call
*/
public FormResponse getForm(String formId, String processDefinitionKey, Long version) throws ApiException {
return this.getForm(formId, processDefinitionKey, version, Collections.emptyMap());
}
/**
* Get a form
* Get the form details by `formId` and `processDefinitionKey` required query param. The `version` query param is optional and is used only for deployed forms (if empty, it retrieves the highest version).
* @param formId The ID of the form. (required)
* @param processDefinitionKey Reference to the process definition. (required)
* @param version The version of the form. Valid only for deployed forms. (optional)
* @param additionalHeaders additionalHeaders for this call
* @return FormResponse
* @throws ApiException if fails to make API call
*/
public FormResponse getForm(String formId, String processDefinitionKey, Long version, Map additionalHeaders) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'formId' is set
if (formId == null) {
throw new ApiException(400, "Missing the required parameter 'formId' when calling getForm");
}
// verify the required parameter 'processDefinitionKey' is set
if (processDefinitionKey == null) {
throw new ApiException(400, "Missing the required parameter 'processDefinitionKey' when calling getForm");
}
// create path and map variables
String localVarPath = "/v1/forms/{formId}"
.replaceAll("\\{" + "formId" + "\\}", apiClient.escapeString(formId.toString()));
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
String localVarQueryParameterBaseName;
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPair("processDefinitionKey", processDefinitionKey));
localVarQueryParams.addAll(apiClient.parameterToPair("version", version));
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
TypeReference localVarReturnType = new TypeReference() {};
return apiClient.invokeAPI(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType
);
}
@Override
public T invokeAPI(String url, String method, Object request, TypeReference returnType, Map additionalHeaders) throws ApiException {
String localVarPath = url.replace(apiClient.getBaseURL(), "");
StringJoiner localVarQueryStringJoiner = new StringJoiner("&");
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarHeaderParams.putAll(additionalHeaders);
final String[] localVarAccepts = {
"application/json", "application/problem+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "cookie", "bearer-key" };
return apiClient.invokeAPI(
localVarPath,
method,
localVarQueryParams,
localVarCollectionQueryParams,
localVarQueryStringJoiner.toString(),
request,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
returnType
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy