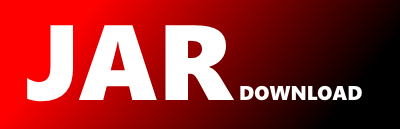
io.camunda.tasklist.generated.model.TaskResponse Maven / Gradle / Ivy
The newest version!
/*
* Tasklist REST API
* Tasklist is a ready-to-use API application to rapidly implement business processes alongside user tasks in Zeebe.
*
* The version of the OpenAPI document: v1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.camunda.tasklist.generated.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.StringJoiner;
/**
* TaskResponse
*/
@JsonPropertyOrder({
TaskResponse.JSON_PROPERTY_ID,
TaskResponse.JSON_PROPERTY_NAME,
TaskResponse.JSON_PROPERTY_TASK_DEFINITION_ID,
TaskResponse.JSON_PROPERTY_PROCESS_NAME,
TaskResponse.JSON_PROPERTY_CREATION_DATE,
TaskResponse.JSON_PROPERTY_COMPLETION_DATE,
TaskResponse.JSON_PROPERTY_ASSIGNEE,
TaskResponse.JSON_PROPERTY_TASK_STATE,
TaskResponse.JSON_PROPERTY_FORM_KEY,
TaskResponse.JSON_PROPERTY_FORM_ID,
TaskResponse.JSON_PROPERTY_FORM_VERSION,
TaskResponse.JSON_PROPERTY_IS_FORM_EMBEDDED,
TaskResponse.JSON_PROPERTY_PROCESS_DEFINITION_KEY,
TaskResponse.JSON_PROPERTY_PROCESS_INSTANCE_KEY,
TaskResponse.JSON_PROPERTY_TENANT_ID,
TaskResponse.JSON_PROPERTY_DUE_DATE,
TaskResponse.JSON_PROPERTY_FOLLOW_UP_DATE,
TaskResponse.JSON_PROPERTY_CANDIDATE_GROUPS,
TaskResponse.JSON_PROPERTY_CANDIDATE_USERS,
TaskResponse.JSON_PROPERTY_IMPLEMENTATION,
TaskResponse.JSON_PROPERTY_PRIORITY
})
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-10T08:38:14.250454645Z[Etc/UTC]", comments = "Generator version: 7.8.0")
public class TaskResponse {
public static final String JSON_PROPERTY_ID = "id";
private String id;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_TASK_DEFINITION_ID = "taskDefinitionId";
private String taskDefinitionId;
public static final String JSON_PROPERTY_PROCESS_NAME = "processName";
private String processName;
public static final String JSON_PROPERTY_CREATION_DATE = "creationDate";
private String creationDate;
public static final String JSON_PROPERTY_COMPLETION_DATE = "completionDate";
private String completionDate;
public static final String JSON_PROPERTY_ASSIGNEE = "assignee";
private String assignee;
/**
* The state of the task.
*/
public enum TaskStateEnum {
CREATED("CREATED"),
COMPLETED("COMPLETED"),
CANCELED("CANCELED"),
FAILED("FAILED");
private String value;
TaskStateEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TaskStateEnum fromValue(String value) {
for (TaskStateEnum b : TaskStateEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_TASK_STATE = "taskState";
private TaskStateEnum taskState;
public static final String JSON_PROPERTY_FORM_KEY = "formKey";
private String formKey;
public static final String JSON_PROPERTY_FORM_ID = "formId";
private String formId;
public static final String JSON_PROPERTY_FORM_VERSION = "formVersion";
private Long formVersion;
public static final String JSON_PROPERTY_IS_FORM_EMBEDDED = "isFormEmbedded";
private Boolean isFormEmbedded;
public static final String JSON_PROPERTY_PROCESS_DEFINITION_KEY = "processDefinitionKey";
private String processDefinitionKey;
public static final String JSON_PROPERTY_PROCESS_INSTANCE_KEY = "processInstanceKey";
private String processInstanceKey;
public static final String JSON_PROPERTY_TENANT_ID = "tenantId";
private String tenantId;
public static final String JSON_PROPERTY_DUE_DATE = "dueDate";
private OffsetDateTime dueDate;
public static final String JSON_PROPERTY_FOLLOW_UP_DATE = "followUpDate";
private OffsetDateTime followUpDate;
public static final String JSON_PROPERTY_CANDIDATE_GROUPS = "candidateGroups";
private List candidateGroups;
public static final String JSON_PROPERTY_CANDIDATE_USERS = "candidateUsers";
private List candidateUsers;
/**
* Gets or Sets implementation
*/
public enum ImplementationEnum {
JOB_WORKER("JOB_WORKER"),
ZEEBE_USER_TASK("ZEEBE_USER_TASK");
private String value;
ImplementationEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ImplementationEnum fromValue(String value) {
for (ImplementationEnum b : ImplementationEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_IMPLEMENTATION = "implementation";
private ImplementationEnum implementation;
public static final String JSON_PROPERTY_PRIORITY = "priority";
private Integer priority;
public TaskResponse() {
}
/**
* Constructor with only readonly parameters
*/
@JsonCreator
public TaskResponse(
@JsonProperty(JSON_PROPERTY_TASK_STATE) TaskStateEnum taskState
) {
this();
this.taskState = taskState;
}
public TaskResponse id(String id) {
this.id = id;
return this;
}
/**
* The unique identifier of the task.
* @return id
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getId() {
return id;
}
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setId(String id) {
this.id = id;
}
public TaskResponse name(String name) {
this.name = name;
return this;
}
/**
* The name of the task.
* @return name
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public TaskResponse taskDefinitionId(String taskDefinitionId) {
this.taskDefinitionId = taskDefinitionId;
return this;
}
/**
* User Task ID from the BPMN definition.
* @return taskDefinitionId
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TASK_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTaskDefinitionId() {
return taskDefinitionId;
}
@JsonProperty(JSON_PROPERTY_TASK_DEFINITION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTaskDefinitionId(String taskDefinitionId) {
this.taskDefinitionId = taskDefinitionId;
}
public TaskResponse processName(String processName) {
this.processName = processName;
return this;
}
/**
* The name of the process.
* @return processName
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PROCESS_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getProcessName() {
return processName;
}
@JsonProperty(JSON_PROPERTY_PROCESS_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProcessName(String processName) {
this.processName = processName;
}
public TaskResponse creationDate(String creationDate) {
this.creationDate = creationDate;
return this;
}
/**
* When was the task created (renamed equivalent of `Task.creationTime` field).
* @return creationDate
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CREATION_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCreationDate() {
return creationDate;
}
@JsonProperty(JSON_PROPERTY_CREATION_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCreationDate(String creationDate) {
this.creationDate = creationDate;
}
public TaskResponse completionDate(String completionDate) {
this.completionDate = completionDate;
return this;
}
/**
* When was the task completed (renamed equivalent of `Task.completionTime` field).
* @return completionDate
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COMPLETION_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCompletionDate() {
return completionDate;
}
@JsonProperty(JSON_PROPERTY_COMPLETION_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCompletionDate(String completionDate) {
this.completionDate = completionDate;
}
public TaskResponse assignee(String assignee) {
this.assignee = assignee;
return this;
}
/**
* The username/id of who is assigned to the task.
* @return assignee
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ASSIGNEE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getAssignee() {
return assignee;
}
@JsonProperty(JSON_PROPERTY_ASSIGNEE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAssignee(String assignee) {
this.assignee = assignee;
}
/**
* The state of the task.
* @return taskState
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TASK_STATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TaskStateEnum getTaskState() {
return taskState;
}
public TaskResponse formKey(String formKey) {
this.formKey = formKey;
return this;
}
/**
* Reference to the task form.
* @return formKey
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FORM_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFormKey() {
return formKey;
}
@JsonProperty(JSON_PROPERTY_FORM_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFormKey(String formKey) {
this.formKey = formKey;
}
public TaskResponse formId(String formId) {
this.formId = formId;
return this;
}
/**
* Reference to the ID of a deployed form. If the form is not deployed, this property is null.
* @return formId
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FORM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFormId() {
return formId;
}
@JsonProperty(JSON_PROPERTY_FORM_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFormId(String formId) {
this.formId = formId;
}
public TaskResponse formVersion(Long formVersion) {
this.formVersion = formVersion;
return this;
}
/**
* Reference to the version of a deployed form. If the form is not deployed, this property is null.
* @return formVersion
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FORM_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getFormVersion() {
return formVersion;
}
@JsonProperty(JSON_PROPERTY_FORM_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFormVersion(Long formVersion) {
this.formVersion = formVersion;
}
public TaskResponse isFormEmbedded(Boolean isFormEmbedded) {
this.isFormEmbedded = isFormEmbedded;
return this;
}
/**
* Is the form embedded for this task? If there is no form, this property is null.
* @return isFormEmbedded
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IS_FORM_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsFormEmbedded() {
return isFormEmbedded;
}
@JsonProperty(JSON_PROPERTY_IS_FORM_EMBEDDED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsFormEmbedded(Boolean isFormEmbedded) {
this.isFormEmbedded = isFormEmbedded;
}
public TaskResponse processDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
return this;
}
/**
* Reference to process definition (renamed equivalent of `Task.processDefinitionId` field).
* @return processDefinitionKey
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getProcessDefinitionKey() {
return processDefinitionKey;
}
@JsonProperty(JSON_PROPERTY_PROCESS_DEFINITION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProcessDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
}
public TaskResponse processInstanceKey(String processInstanceKey) {
this.processInstanceKey = processInstanceKey;
return this;
}
/**
* Reference to process instance id (renamed equivalent of `Task.processInstanceId` field).
* @return processInstanceKey
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getProcessInstanceKey() {
return processInstanceKey;
}
@JsonProperty(JSON_PROPERTY_PROCESS_INSTANCE_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProcessInstanceKey(String processInstanceKey) {
this.processInstanceKey = processInstanceKey;
}
public TaskResponse tenantId(String tenantId) {
this.tenantId = tenantId;
return this;
}
/**
* The tenant ID associated with the task.
* @return tenantId
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TENANT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTenantId() {
return tenantId;
}
@JsonProperty(JSON_PROPERTY_TENANT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTenantId(String tenantId) {
this.tenantId = tenantId;
}
public TaskResponse dueDate(OffsetDateTime dueDate) {
this.dueDate = dueDate;
return this;
}
/**
* The due date for the task.
* @return dueDate
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DUE_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getDueDate() {
return dueDate;
}
@JsonProperty(JSON_PROPERTY_DUE_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDueDate(OffsetDateTime dueDate) {
this.dueDate = dueDate;
}
public TaskResponse followUpDate(OffsetDateTime followUpDate) {
this.followUpDate = followUpDate;
return this;
}
/**
* The follow-up date for the task.
* @return followUpDate
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_FOLLOW_UP_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getFollowUpDate() {
return followUpDate;
}
@JsonProperty(JSON_PROPERTY_FOLLOW_UP_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFollowUpDate(OffsetDateTime followUpDate) {
this.followUpDate = followUpDate;
}
public TaskResponse candidateGroups(List candidateGroups) {
this.candidateGroups = candidateGroups;
return this;
}
public TaskResponse addCandidateGroupsItem(String candidateGroupsItem) {
if (this.candidateGroups == null) {
this.candidateGroups = new ArrayList<>();
}
this.candidateGroups.add(candidateGroupsItem);
return this;
}
/**
* The candidate groups for the task.
* @return candidateGroups
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CANDIDATE_GROUPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCandidateGroups() {
return candidateGroups;
}
@JsonProperty(JSON_PROPERTY_CANDIDATE_GROUPS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCandidateGroups(List candidateGroups) {
this.candidateGroups = candidateGroups;
}
public TaskResponse candidateUsers(List candidateUsers) {
this.candidateUsers = candidateUsers;
return this;
}
public TaskResponse addCandidateUsersItem(String candidateUsersItem) {
if (this.candidateUsers == null) {
this.candidateUsers = new ArrayList<>();
}
this.candidateUsers.add(candidateUsersItem);
return this;
}
/**
* The candidate users for the task.
* @return candidateUsers
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CANDIDATE_USERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCandidateUsers() {
return candidateUsers;
}
@JsonProperty(JSON_PROPERTY_CANDIDATE_USERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCandidateUsers(List candidateUsers) {
this.candidateUsers = candidateUsers;
}
public TaskResponse implementation(ImplementationEnum implementation) {
this.implementation = implementation;
return this;
}
/**
* Get implementation
* @return implementation
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IMPLEMENTATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ImplementationEnum getImplementation() {
return implementation;
}
@JsonProperty(JSON_PROPERTY_IMPLEMENTATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setImplementation(ImplementationEnum implementation) {
this.implementation = implementation;
}
public TaskResponse priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* The assigned priority of the task. Only for Zeebe User Tasks.
* @return priority
*/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PRIORITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getPriority() {
return priority;
}
@JsonProperty(JSON_PROPERTY_PRIORITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPriority(Integer priority) {
this.priority = priority;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskResponse taskResponse = (TaskResponse) o;
return Objects.equals(this.id, taskResponse.id) &&
Objects.equals(this.name, taskResponse.name) &&
Objects.equals(this.taskDefinitionId, taskResponse.taskDefinitionId) &&
Objects.equals(this.processName, taskResponse.processName) &&
Objects.equals(this.creationDate, taskResponse.creationDate) &&
Objects.equals(this.completionDate, taskResponse.completionDate) &&
Objects.equals(this.assignee, taskResponse.assignee) &&
Objects.equals(this.taskState, taskResponse.taskState) &&
Objects.equals(this.formKey, taskResponse.formKey) &&
Objects.equals(this.formId, taskResponse.formId) &&
Objects.equals(this.formVersion, taskResponse.formVersion) &&
Objects.equals(this.isFormEmbedded, taskResponse.isFormEmbedded) &&
Objects.equals(this.processDefinitionKey, taskResponse.processDefinitionKey) &&
Objects.equals(this.processInstanceKey, taskResponse.processInstanceKey) &&
Objects.equals(this.tenantId, taskResponse.tenantId) &&
Objects.equals(this.dueDate, taskResponse.dueDate) &&
Objects.equals(this.followUpDate, taskResponse.followUpDate) &&
Objects.equals(this.candidateGroups, taskResponse.candidateGroups) &&
Objects.equals(this.candidateUsers, taskResponse.candidateUsers) &&
Objects.equals(this.implementation, taskResponse.implementation) &&
Objects.equals(this.priority, taskResponse.priority);
}
@Override
public int hashCode() {
return Objects.hash(id, name, taskDefinitionId, processName, creationDate, completionDate, assignee, taskState, formKey, formId, formVersion, isFormEmbedded, processDefinitionKey, processInstanceKey, tenantId, dueDate, followUpDate, candidateGroups, candidateUsers, implementation, priority);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskResponse {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" taskDefinitionId: ").append(toIndentedString(taskDefinitionId)).append("\n");
sb.append(" processName: ").append(toIndentedString(processName)).append("\n");
sb.append(" creationDate: ").append(toIndentedString(creationDate)).append("\n");
sb.append(" completionDate: ").append(toIndentedString(completionDate)).append("\n");
sb.append(" assignee: ").append(toIndentedString(assignee)).append("\n");
sb.append(" taskState: ").append(toIndentedString(taskState)).append("\n");
sb.append(" formKey: ").append(toIndentedString(formKey)).append("\n");
sb.append(" formId: ").append(toIndentedString(formId)).append("\n");
sb.append(" formVersion: ").append(toIndentedString(formVersion)).append("\n");
sb.append(" isFormEmbedded: ").append(toIndentedString(isFormEmbedded)).append("\n");
sb.append(" processDefinitionKey: ").append(toIndentedString(processDefinitionKey)).append("\n");
sb.append(" processInstanceKey: ").append(toIndentedString(processInstanceKey)).append("\n");
sb.append(" tenantId: ").append(toIndentedString(tenantId)).append("\n");
sb.append(" dueDate: ").append(toIndentedString(dueDate)).append("\n");
sb.append(" followUpDate: ").append(toIndentedString(followUpDate)).append("\n");
sb.append(" candidateGroups: ").append(toIndentedString(candidateGroups)).append("\n");
sb.append(" candidateUsers: ").append(toIndentedString(candidateUsers)).append("\n");
sb.append(" implementation: ").append(toIndentedString(implementation)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
/**
* Convert the instance into URL query string.
*
* @return URL query string
*/
public String toUrlQueryString() {
return toUrlQueryString(null);
}
/**
* Convert the instance into URL query string.
*
* @param prefix prefix of the query string
* @return URL query string
*/
public String toUrlQueryString(String prefix) {
String suffix = "";
String containerSuffix = "";
String containerPrefix = "";
if (prefix == null) {
// style=form, explode=true, e.g. /pet?name=cat&type=manx
prefix = "";
} else {
// deepObject style e.g. /pet?id[name]=cat&id[type]=manx
prefix = prefix + "[";
suffix = "]";
containerSuffix = "]";
containerPrefix = "[";
}
StringJoiner joiner = new StringJoiner("&");
// add `id` to the URL query string
if (getId() != null) {
try {
joiner.add(String.format("%sid%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `name` to the URL query string
if (getName() != null) {
try {
joiner.add(String.format("%sname%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getName()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `taskDefinitionId` to the URL query string
if (getTaskDefinitionId() != null) {
try {
joiner.add(String.format("%staskDefinitionId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTaskDefinitionId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processName` to the URL query string
if (getProcessName() != null) {
try {
joiner.add(String.format("%sprocessName%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessName()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `creationDate` to the URL query string
if (getCreationDate() != null) {
try {
joiner.add(String.format("%screationDate%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCreationDate()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `completionDate` to the URL query string
if (getCompletionDate() != null) {
try {
joiner.add(String.format("%scompletionDate%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getCompletionDate()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `assignee` to the URL query string
if (getAssignee() != null) {
try {
joiner.add(String.format("%sassignee%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getAssignee()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `taskState` to the URL query string
if (getTaskState() != null) {
try {
joiner.add(String.format("%staskState%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTaskState()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `formKey` to the URL query string
if (getFormKey() != null) {
try {
joiner.add(String.format("%sformKey%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getFormKey()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `formId` to the URL query string
if (getFormId() != null) {
try {
joiner.add(String.format("%sformId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getFormId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `formVersion` to the URL query string
if (getFormVersion() != null) {
try {
joiner.add(String.format("%sformVersion%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getFormVersion()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `isFormEmbedded` to the URL query string
if (getIsFormEmbedded() != null) {
try {
joiner.add(String.format("%sisFormEmbedded%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getIsFormEmbedded()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processDefinitionKey` to the URL query string
if (getProcessDefinitionKey() != null) {
try {
joiner.add(String.format("%sprocessDefinitionKey%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessDefinitionKey()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `processInstanceKey` to the URL query string
if (getProcessInstanceKey() != null) {
try {
joiner.add(String.format("%sprocessInstanceKey%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getProcessInstanceKey()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `tenantId` to the URL query string
if (getTenantId() != null) {
try {
joiner.add(String.format("%stenantId%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getTenantId()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `dueDate` to the URL query string
if (getDueDate() != null) {
try {
joiner.add(String.format("%sdueDate%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getDueDate()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `followUpDate` to the URL query string
if (getFollowUpDate() != null) {
try {
joiner.add(String.format("%sfollowUpDate%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getFollowUpDate()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `candidateGroups` to the URL query string
if (getCandidateGroups() != null) {
for (int i = 0; i < getCandidateGroups().size(); i++) {
try {
joiner.add(String.format("%scandidateGroups%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getCandidateGroups().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `candidateUsers` to the URL query string
if (getCandidateUsers() != null) {
for (int i = 0; i < getCandidateUsers().size(); i++) {
try {
joiner.add(String.format("%scandidateUsers%s%s=%s", prefix, suffix,
"".equals(suffix) ? "" : String.format("%s%d%s", containerPrefix, i, containerSuffix),
URLEncoder.encode(String.valueOf(getCandidateUsers().get(i)), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
}
// add `implementation` to the URL query string
if (getImplementation() != null) {
try {
joiner.add(String.format("%simplementation%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getImplementation()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
// add `priority` to the URL query string
if (getPriority() != null) {
try {
joiner.add(String.format("%spriority%s=%s", prefix, suffix, URLEncoder.encode(String.valueOf(getPriority()), "UTF-8").replaceAll("\\+", "%20")));
} catch (UnsupportedEncodingException e) {
// Should never happen, UTF-8 is always supported
throw new RuntimeException(e);
}
}
return joiner.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy