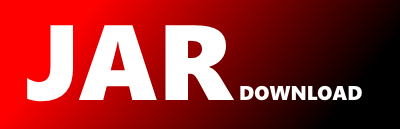
io.camunda.exporter.rdbms.UserExportHandler Maven / Gradle / Ivy
The newest version!
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Camunda License 1.0. You may not use this file
* except in compliance with the Camunda License 1.0.
*/
package io.camunda.exporter.rdbms;
import io.camunda.db.rdbms.write.domain.UserDbModel;
import io.camunda.db.rdbms.write.service.UserWriter;
import io.camunda.zeebe.protocol.record.Record;
import io.camunda.zeebe.protocol.record.ValueType;
import io.camunda.zeebe.protocol.record.intent.UserIntent;
import io.camunda.zeebe.protocol.record.value.UserRecordValue;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserExportHandler implements RdbmsExportHandler {
private static final Logger LOG = LoggerFactory.getLogger(UserExportHandler.class);
private final UserWriter userWriter;
public UserExportHandler(final UserWriter userWriter) {
this.userWriter = userWriter;
}
@Override
public boolean canExport(final Record record) {
// do not react on UserIntent.DELETED to keep historic data
return record.getValueType() == ValueType.USER
&& (record.getIntent() == UserIntent.CREATED
|| record.getIntent() == UserIntent.UPDATED
|| record.getIntent() == UserIntent.DELETED);
}
@Override
public void export(final Record record) {
final UserRecordValue value = record.getValue();
if (record.getIntent() == UserIntent.CREATED) {
userWriter.create(map(value));
} else if (record.getIntent() == UserIntent.UPDATED) {
userWriter.update(map(value));
} else if (record.getIntent() == UserIntent.DELETED) {
userWriter.delete(value.getUserKey());
} else {
LOG.warn("Unexpected intent {} for user record", record.getIntent());
}
}
private UserDbModel map(final UserRecordValue decision) {
return new UserDbModel.Builder()
.userKey(decision.getUserKey())
.username(decision.getUsername())
.name(decision.getName())
.email(decision.getEmail())
.password(decision.getPassword())
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy