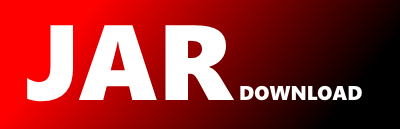
io.camunda.tasklist.store.VariableStore Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Camunda License 1.0. You may not use this file
* except in compliance with the Camunda License 1.0.
*/
package io.camunda.tasklist.store;
import io.camunda.tasklist.entities.*;
import io.camunda.tasklist.views.TaskSearchView;
import io.camunda.webapps.schema.descriptors.tasklist.template.SnapshotTaskVariableTemplate;
import io.camunda.webapps.schema.entities.tasklist.SnapshotTaskVariableEntity;
import io.camunda.webapps.schema.entities.tasklist.TaskEntity;
import io.camunda.webapps.schema.entities.tasklist.TaskState;
import java.util.*;
import java.util.stream.Collectors;
public interface VariableStore {
public List getVariablesByFlowNodeInstanceIds(
List flowNodeInstanceIds, List varNames, final Set fieldNames);
public Map> getTaskVariablesPerTaskId(
final List requests);
Map getTaskVariablesIdsWithIndexByTaskIds(final List taskIds);
public void persistTaskVariables(final Collection finalVariables);
public List getFlowNodeInstances(final List processInstanceIds);
public VariableEntity getRuntimeVariable(final String variableId, Set fieldNames);
public SnapshotTaskVariableEntity getTaskVariable(
final String variableId, Set fieldNames);
public List getProcessInstanceIdsWithMatchingVars(
List varNames, List varValues);
private static Optional getElsFieldByGraphqlField(final String fieldName) {
switch (fieldName) {
case ("id"):
return Optional.of(SnapshotTaskVariableTemplate.ID);
case ("name"):
return Optional.of(SnapshotTaskVariableTemplate.NAME);
case ("value"):
return Optional.of(SnapshotTaskVariableTemplate.FULL_VALUE);
case ("previewValue"):
return Optional.of(SnapshotTaskVariableTemplate.VALUE);
case ("isValueTruncated"):
return Optional.of(SnapshotTaskVariableTemplate.IS_PREVIEW);
default:
return Optional.empty();
}
}
public static Set getElsFieldsByGraphqlFields(final Set fieldNames) {
return fieldNames.stream()
.map((fn) -> getElsFieldByGraphqlField(fn))
.flatMap(Optional::stream)
.collect(Collectors.toSet());
}
static class FlowNodeTree extends HashMap {
public String getParent(String currentFlowNodeInstanceId) {
return super.get(currentFlowNodeInstanceId);
}
public void setParent(String currentFlowNodeInstanceId, String parentFlowNodeInstanceId) {
super.put(currentFlowNodeInstanceId, parentFlowNodeInstanceId);
}
public Set getFlowNodeInstanceIds() {
return super.keySet();
}
}
static class VariableMap extends HashMap {
public void putAll(final VariableMap m) {
for (Entry entry : m.entrySet()) {
// since we build variable map from bottom to top of the flow node tree, we don't overwrite
// the values from lower (inner) scopes with those from upper (outer) scopes
putIfAbsent(entry.getKey(), entry.getValue());
}
}
@Override
@Deprecated
public void putAll(final Map extends String, ? extends VariableEntity> m) {
super.putAll(m);
}
}
public static class GetVariablesRequest {
private String taskId;
private TaskState state;
private String flowNodeInstanceId;
private String processInstanceId;
private List varNames;
private Set fieldNames = new HashSet<>();
public static GetVariablesRequest createFrom(TaskEntity taskEntity, Set fieldNames) {
return new GetVariablesRequest()
.setTaskId(taskEntity.getId())
.setFlowNodeInstanceId(taskEntity.getFlowNodeInstanceId())
.setState(taskEntity.getState())
.setProcessInstanceId(taskEntity.getProcessInstanceId())
.setFieldNames(fieldNames);
}
public static GetVariablesRequest createFrom(TaskEntity taskEntity) {
return new GetVariablesRequest()
.setTaskId(taskEntity.getId())
.setFlowNodeInstanceId(taskEntity.getFlowNodeInstanceId())
.setState(taskEntity.getState())
.setProcessInstanceId(taskEntity.getProcessInstanceId());
}
public static GetVariablesRequest createFrom(
TaskSearchView taskSearchView, List varNames, Set fieldNames) {
return new GetVariablesRequest()
.setTaskId(taskSearchView.getId())
.setFlowNodeInstanceId(taskSearchView.getFlowNodeInstanceId())
.setState(taskSearchView.getState())
.setProcessInstanceId(taskSearchView.getProcessInstanceId())
.setVarNames(varNames)
.setFieldNames(fieldNames);
}
public String getTaskId() {
return taskId;
}
public GetVariablesRequest setTaskId(final String taskId) {
this.taskId = taskId;
return this;
}
public TaskState getState() {
return state;
}
public GetVariablesRequest setState(final TaskState state) {
this.state = state;
return this;
}
public String getFlowNodeInstanceId() {
return flowNodeInstanceId;
}
public GetVariablesRequest setFlowNodeInstanceId(final String flowNodeInstanceId) {
this.flowNodeInstanceId = flowNodeInstanceId;
return this;
}
public String getProcessInstanceId() {
return processInstanceId;
}
public GetVariablesRequest setProcessInstanceId(final String processInstanceId) {
this.processInstanceId = processInstanceId;
return this;
}
public List getVarNames() {
return varNames;
}
public GetVariablesRequest setVarNames(final List varNames) {
this.varNames = varNames;
return this;
}
public Set getFieldNames() {
return fieldNames;
}
public GetVariablesRequest setFieldNames(final Set fieldNames) {
this.fieldNames = fieldNames;
return this;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
final GetVariablesRequest that = (GetVariablesRequest) o;
return Objects.equals(taskId, that.taskId)
&& state == that.state
&& Objects.equals(flowNodeInstanceId, that.flowNodeInstanceId)
&& Objects.equals(processInstanceId, that.processInstanceId)
&& Objects.equals(varNames, that.varNames)
&& Objects.equals(fieldNames, that.fieldNames);
}
@Override
public int hashCode() {
return Objects.hash(
taskId, state, flowNodeInstanceId, processInstanceId, varNames, fieldNames);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy