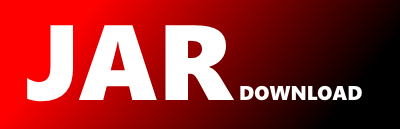
io.camunda.zeebe.model.bpmn.validation.zeebe.EscalationValidator Maven / Gradle / Ivy
/*
* Copyright © 2017 camunda services GmbH ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.camunda.zeebe.model.bpmn.validation.zeebe;
import io.camunda.zeebe.model.bpmn.instance.Escalation;
import io.camunda.zeebe.model.bpmn.instance.EscalationEventDefinition;
import io.camunda.zeebe.model.bpmn.instance.Process;
import io.camunda.zeebe.model.bpmn.instance.ThrowEvent;
import java.util.Collection;
import java.util.List;
import java.util.stream.Collectors;
import org.camunda.bpm.model.xml.impl.ModelInstanceImpl;
import org.camunda.bpm.model.xml.impl.util.ModelUtil;
import org.camunda.bpm.model.xml.instance.DomElement;
import org.camunda.bpm.model.xml.instance.ModelElementInstance;
import org.camunda.bpm.model.xml.validation.ModelElementValidator;
import org.camunda.bpm.model.xml.validation.ValidationResultCollector;
public class EscalationValidator implements ModelElementValidator {
@Override
public Class getElementType() {
return Escalation.class;
}
@Override
public void validate(
final Escalation element, final ValidationResultCollector validationResultCollector) {
if (isReferredByThrowEvent(element)) {
validateEscalationCode(element, validationResultCollector);
}
}
private void validateEscalationCode(
final Escalation element, final ValidationResultCollector validationResultCollector) {
if (element.getEscalationCode() == null || element.getEscalationCode().isEmpty()) {
validationResultCollector.addError(0, "EscalationCode must be present and not empty");
}
}
private boolean isReferredByThrowEvent(final Escalation element) {
final Collection throwEvents = getAllElementsByType(element, ThrowEvent.class);
return throwEvents.stream()
.flatMap(i -> i.getEventDefinitions().stream())
.anyMatch(
e ->
e instanceof EscalationEventDefinition
&& ((EscalationEventDefinition) e).getEscalation() == element);
}
private Collection getAllElementsByType(
final Escalation element, final Class type) {
return element.getParentElement().getChildElementsByType(Process.class).stream()
.flatMap(p -> getAllElementsByTypeRecursive(p, type).stream())
.collect(Collectors.toList());
}
private Collection getAllElementsByTypeRecursive(
final ModelElementInstance element, final Class type) {
// look for immediate children
final Collection result = element.getChildElementsByType(type);
// look for children in subtree
final List childDomElements = element.getDomElement().getChildElements();
final Collection childModelElements =
ModelUtil.getModelElementCollection(
childDomElements, (ModelInstanceImpl) element.getModelInstance());
result.addAll(
childModelElements.stream()
.flatMap(child -> getAllElementsByTypeRecursive(child, type).stream())
.collect(Collectors.toList()));
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy