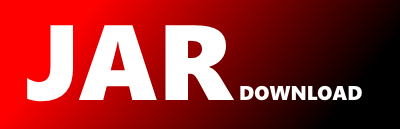
io.carml.engine.sourceresolver.FileResolver Maven / Gradle / Ivy
package io.carml.engine.sourceresolver;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Optional;
import lombok.AccessLevel;
import lombok.AllArgsConstructor;
@AllArgsConstructor(access = AccessLevel.PRIVATE)
public class FileResolver implements SourceResolver {
private final Path basePath;
public static FileResolver of(Path basePath) {
return new FileResolver(basePath);
}
@Override
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy