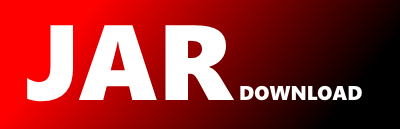
io.cdap.wrangler.api.Arguments Maven / Gradle / Ivy
/*
* Copyright © 2017-2019 Cask Data, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package io.cdap.wrangler.api;
import com.google.gson.JsonElement;
import io.cdap.wrangler.api.parser.Token;
import io.cdap.wrangler.api.parser.TokenType;
/**
* This class {@code Arguments} represents the wrapped tokens that
* are tokenized and parsed arguments provided to the {@code Executor}.
*
* This class Arguments
includes methods for retrieving
* the value of the token provided the name for the token, number of
* tokens, support for checking if the named argument exists, type of
* token as specified by TokenType
and helper method for
* constructing JsonElement
object.
*
* @see io.cdap.wrangler.api.parser.UsageDefinition
*/
public interface Arguments {
/**
* This method returns the token {@code value} based on the {@code name}
* specified in the argument. This method will attempt to convert the token
* into the expected return type T
.
*
* If the name
doesn't exist in this object, then this
* method is expected to return null
*
* @param name of the token to be retrieved.
* @param type the token need to casted to.
* @return object that extends Token
.
*/
T value(String name);
/**
* Returns the number of tokens that are mapped to arguments.
*
* The optional arguments specified during the UsageDefinition
* are not included in the size if they are not present in the tokens parsed.
*
* @return number of tokens parsed, excluding optional tokens if not present.
*/
int size();
/**
* This method checks if there exists a token named name
registered
* with this object.
*
* The name
is expected to the same as specified in the UsageDefinition
.
* There are two reason why the name
might not exists in this object :
*
*
* - When an token is defined to be optional, the user might not have specified the
* token, hence the token would not exist in the argument.
* - User has specified invalid
name
.
*
*
* @param name associated with the token.
* @return true if argument with name name
exists, false otherwise.
*/
boolean contains(String name);
/**
* Each token is defined as one of the types defined in the class {@link TokenType}.
* When the directive is parsed into token, the type of the token is passed through.
*
* @param name associated with the token.
* @return TokenType
associated with argument name
, else null.
*/
TokenType type(String name);
/**
* Returns the source line number these arguments were parsed from.
*
* @return the source line number.
*/
int line();
/**
* Returns the source column number these arguments were parsed from.
* It takes the start position of the directive as the column number.
*
* @return the start of the column number for the start of the directive
* these arguments contain.
*/
int column();
/**
* This method returns the original source line of the directive as specified
* the user. It returns the String
representation of the directive.
*
* @return String
object representing the original directive
* as specified by the user.
*/
String source();
/**
* Returns JsonElement
representation of this object.
*
* @return an instance of JsonElement
object representing all the
* named tokens held within this object.
*/
JsonElement toJson();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy