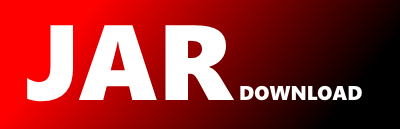
io.cequence.pineconescala.service.PineconeIndexService.scala Maven / Gradle / Ivy
package io.cequence.pineconescala.service
import io.cequence.pineconescala.domain.response._
import io.cequence.pineconescala.domain.settings.IndexSettings
import io.cequence.wsclient.service.CloseableService
import scala.concurrent.Future
/**
* Central service to access all Pinecone vector operations/endpoints as defined at the API ref. page
*
* The following services are supported:
*
* - '''Collection Operations''': listCollections, createCollection, describeCollection, and
* deleteCollection
*
* - '''Index Operations''': listIndexes, creatIndex, describeIndex, deleteIndex, and
* configureIndex
*
* @since Apr
* 2023
*/
trait PineconeIndexService[S <: IndexSettings]
extends CloseableService
with PineconeServiceConsts {
/**
* Get a description of a collection.
*
* @param collectionName
* The name of the collection
* @return
* Configuration information and deployment status of the collection (if found)
* @see
* Pinecone Doc
*/
def describeCollection(
collectionName: String
): Future[Option[CollectionInfo]]
/**
* This operation deletes an existing collection.
*
* @param collectionName
* The name of the collection
* @return
* Whether the collection was deleted successfully or not found.
* @see
* Pinecone Doc
*/
def deleteCollection(
collectionName: String
): Future[DeleteResponse]
/**
* This operation returns a list of your Pinecone indexes.
*
* @return
* List of indexes associated with the account (API key)
* @see
* Pinecone Doc
*/
def listIndexes: Future[Seq[String]]
/**
* This operation creates a Pinecone index. You can use it to specify the measure of
* similarity, the dimension of vectors to be stored in the index, the numbers of replicas to
* use, and more.
*
* @param name
* The name of the index to be created. The maximum length is 45 characters.
* @param dimension
* The dimensions of the vectors to be inserted in the index
* @param settings
* The settings for the index
* @return
* Whether the index was created successfully or not.
* @see
* Pinecone Doc
*/
def createIndex(
name: String,
dimension: Int,
settings: S
): Future[CreateResponse]
/**
* Get a description of an index.
*
* @param indexName
* The name of the index
* @return
* Configuration information and deployment status of the index (if found)
* @see
* Pinecone Doc
*/
def describeIndex(
indexName: String
): Future[Option[IndexInfo]]
/**
* This operation deletes an existing index.
*
* @param indexName
* The name of the index
* @return
* Whether the index was deleted successfully or not found.
* @see
* Pinecone Doc
*/
def deleteIndex(
indexName: String
): Future[DeleteResponse]
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy