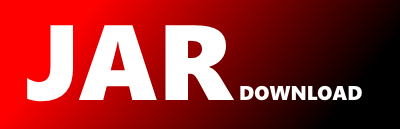
io.cequence.pineconescala.service.PineconeInferenceService.scala Maven / Gradle / Ivy
package io.cequence.pineconescala.service
import io.cequence.pineconescala.domain.response.{EvaluateResponse, GenerateEmbeddingsResponse, RerankResponse}
import io.cequence.pineconescala.domain.settings.{GenerateEmbeddingsSettings, RerankSettings}
import io.cequence.wsclient.service.CloseableService
import scala.concurrent.Future
/**
* Pinecone inference operations as defined at the API ref. page
*
* The following services are supported:
*
* - createEmbeddings
* - rerank
* - evaluate
*
* @since May
* 2024
*/
trait PineconeInferenceService extends CloseableService with PineconeServiceConsts {
/**
* Uses the specified model to generate embeddings for the input sequence.
*
* @param inputs
* Input sequence for which to generate embeddings.
* @param settings
* @return
* list of embeddings inside an envelope
*
* @see
* Pinecone
* Doc
*/
// TODO: rename to embedData to be consistent with the API
def createEmbeddings(
inputs: Seq[String],
settings: GenerateEmbeddingsSettings = DefaultSettings.GenerateEmbeddings
): Future[GenerateEmbeddingsResponse]
/**
* Using a reranker to rerank a list of items for a query.
*
* @param query
* The query to rerank documents against (required)
* @param documents
* The documents to rerank (required)
* @param settings
* @return
*
* @see
* Pinecone
* Doc
*/
def rerank(
query: String,
documents: Seq[Map[String, Any]],
settings: RerankSettings = DefaultSettings.Rerank
): Future[RerankResponse]
/**
* Evaluate an answer
*
* The metrics_alignment endpoint evaluates the correctness, completeness, and alignment of a
* generated answer with respect to a question and a ground truth answer. The correctness and
* completeness are evaluated based on the precision and recall of the generated answer with
* respect to the ground truth answer facts. Alignment is the harmonic mean of correctness
* and completeness.
*
* Note: Originally in the Pinecone API this function is part of Assistant API.
*
* @param question
* The question for which the answer was generated.
* @param answer
* The generated answer.
* @param groundTruthAnswer
* The ground truth answer to the question.
* @return
*/
def evaluate(
question: String,
answer: String,
groundTruthAnswer: String
): Future[EvaluateResponse]
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy