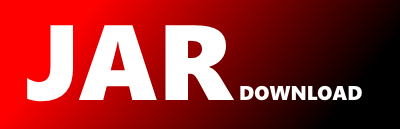
io.cettia.asity.http.AbstractServerHttpExchange Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.cettia.asity.http;
import io.cettia.asity.action.Action;
import io.cettia.asity.action.Actions;
import io.cettia.asity.action.SimpleActions;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.ByteArrayOutputStream;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import java.util.Iterator;
import java.util.List;
/**
* Abstract base class for {@link ServerHttpExchange}.
*
* @author Donghwan Kim
*/
public abstract class AbstractServerHttpExchange implements ServerHttpExchange {
protected final Actions endActions = new SimpleActions<>(new Actions.Options().once(true)
.memory(true));
protected final Actions errorActions = new SimpleActions<>();
protected final Actions closeActions = new SimpleActions<>(new Actions.Options().once
(true).memory(true));
private final Logger logger = LoggerFactory.getLogger(AbstractServerHttpExchange.class);
private final Actions
© 2015 - 2025 Weber Informatics LLC | Privacy Policy