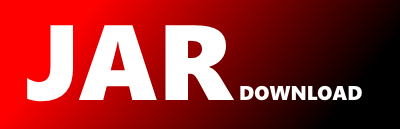
io.ciera.tool.sql.architecture.classes.impl.ModelInstImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.architecture.classes.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.architecture.classes.Attribute;
import io.ciera.tool.sql.architecture.classes.AttributeAccessor;
import io.ciera.tool.sql.architecture.classes.AttributeAccessorSet;
import io.ciera.tool.sql.architecture.classes.AttributeSet;
import io.ciera.tool.sql.architecture.classes.ClassRelationship;
import io.ciera.tool.sql.architecture.classes.ClassRelationshipSet;
import io.ciera.tool.sql.architecture.classes.InstSet;
import io.ciera.tool.sql.architecture.classes.InstanceSelector;
import io.ciera.tool.sql.architecture.classes.InstanceSelectorSet;
import io.ciera.tool.sql.architecture.classes.ModelInst;
import io.ciera.tool.sql.architecture.classes.Operation;
import io.ciera.tool.sql.architecture.classes.OperationSet;
import io.ciera.tool.sql.architecture.classes.Selector;
import io.ciera.tool.sql.architecture.classes.impl.AttributeSetImpl;
import io.ciera.tool.sql.architecture.classes.impl.ClassRelationshipSetImpl;
import io.ciera.tool.sql.architecture.classes.impl.InstSetImpl;
import io.ciera.tool.sql.architecture.classes.impl.InstanceSelectorSetImpl;
import io.ciera.tool.sql.architecture.classes.impl.OperationSetImpl;
import io.ciera.tool.sql.architecture.component.ComponentDefinition;
import io.ciera.tool.sql.architecture.component.InstancePopulationSelector;
import io.ciera.tool.sql.architecture.component.impl.ComponentDefinitionImpl;
import io.ciera.tool.sql.architecture.component.impl.InstancePopulationSelectorImpl;
import io.ciera.tool.sql.architecture.expression.Creation;
import io.ciera.tool.sql.architecture.expression.CreationSet;
import io.ciera.tool.sql.architecture.expression.impl.CreationSetImpl;
import io.ciera.tool.sql.architecture.file.File;
import io.ciera.tool.sql.architecture.file.impl.FileImpl;
import io.ciera.tool.sql.architecture.statemachine.Event;
import io.ciera.tool.sql.architecture.statemachine.EventSet;
import io.ciera.tool.sql.architecture.statemachine.StateMachine;
import io.ciera.tool.sql.architecture.statemachine.impl.StateMachineImpl;
import io.ciera.tool.sql.architecture.type.Type;
import io.ciera.tool.sql.architecture.type.impl.TypeImpl;
import io.ciera.tool.sql.loader.EventInstanceLoader;
import io.ciera.tool.sql.loader.impl.EventInstanceLoaderImpl;
import io.ciera.tool.sql.ooaofooa.subsystem.ModelClass;
import io.ciera.tool.sql.ooaofooa.subsystem.impl.ModelClassImpl;
import java.util.Iterator;
import types.AttributeAccessorType;
import types.ImportType;
import types.Mult;
public class ModelInstImpl extends ModelInstance implements ModelInst {
public static final String KEY_LETTERS = "Class";
public static final ModelInst EMPTY_MODELINST = new EmptyModelInst();
private Sql context;
// constructors
private ModelInstImpl( Sql context ) {
this.context = context;
ref_comp_name = "";
ref_comp_package = "";
ref_name = "";
ref_package = "";
m_extends = "";
m_key_letters = "";
R3017_EventInstanceLoader_inst = EventInstanceLoaderImpl.EMPTY_EVENTINSTANCELOADER;
R401_is_a_File_inst = FileImpl.EMPTY_FILE;
R406_is_type_of_single_element_in_InstSet_inst = InstSetImpl.EMPTY_INSTSET;
R407_is_a_Type_inst = TypeImpl.EMPTY_TYPE;
R408_forms_instance_population_of_ComponentDefinition_inst = ComponentDefinitionImpl.EMPTY_COMPONENTDEFINITION;
R409_ModelClass_inst = ModelClassImpl.EMPTY_MODELCLASS;
R410_data_abstracted_by_Attribute_set = new AttributeSetImpl();
R416_provides_Operation_set = new OperationSetImpl();
R434_participates_in_ClassRelationship_set = new ClassRelationshipSetImpl();
R435_formalizes_ClassRelationship_set = new ClassRelationshipSetImpl();
R442_selects_instances_through_InstanceSelector_set = new InstanceSelectorSetImpl();
R449_extent_accessed_by_InstancePopulationSelector_inst = InstancePopulationSelectorImpl.EMPTY_INSTANCEPOPULATIONSELECTOR;
R4750_behavior_modeled_by_StateMachine_inst = StateMachineImpl.EMPTY_STATEMACHINE;
R780_created_by_Creation_set = new CreationSetImpl();
}
private ModelInstImpl( Sql context, UniqueId instanceId, String ref_comp_name, String ref_comp_package, String ref_name, String ref_package, String m_extends, String m_key_letters ) {
super(instanceId);
this.context = context;
this.ref_comp_name = ref_comp_name;
this.ref_comp_package = ref_comp_package;
this.ref_name = ref_name;
this.ref_package = ref_package;
this.m_extends = m_extends;
this.m_key_letters = m_key_letters;
R3017_EventInstanceLoader_inst = EventInstanceLoaderImpl.EMPTY_EVENTINSTANCELOADER;
R401_is_a_File_inst = FileImpl.EMPTY_FILE;
R406_is_type_of_single_element_in_InstSet_inst = InstSetImpl.EMPTY_INSTSET;
R407_is_a_Type_inst = TypeImpl.EMPTY_TYPE;
R408_forms_instance_population_of_ComponentDefinition_inst = ComponentDefinitionImpl.EMPTY_COMPONENTDEFINITION;
R409_ModelClass_inst = ModelClassImpl.EMPTY_MODELCLASS;
R410_data_abstracted_by_Attribute_set = new AttributeSetImpl();
R416_provides_Operation_set = new OperationSetImpl();
R434_participates_in_ClassRelationship_set = new ClassRelationshipSetImpl();
R435_formalizes_ClassRelationship_set = new ClassRelationshipSetImpl();
R442_selects_instances_through_InstanceSelector_set = new InstanceSelectorSetImpl();
R449_extent_accessed_by_InstancePopulationSelector_inst = InstancePopulationSelectorImpl.EMPTY_INSTANCEPOPULATIONSELECTOR;
R4750_behavior_modeled_by_StateMachine_inst = StateMachineImpl.EMPTY_STATEMACHINE;
R780_created_by_Creation_set = new CreationSetImpl();
}
public static ModelInst create( Sql context ) throws XtumlException {
ModelInst newModelInst = new ModelInstImpl( context );
if ( context.addInstance( newModelInst ) ) {
newModelInst.getRunContext().addChange(new InstanceCreatedDelta(newModelInst, KEY_LETTERS));
return newModelInst;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static ModelInst create( Sql context, String ref_comp_name, String ref_comp_package, String ref_name, String ref_package, String m_extends, String m_key_letters ) throws XtumlException {
return create(context, UniqueId.random(), ref_comp_name, ref_comp_package, ref_name, ref_package, m_extends, m_key_letters);
}
public static ModelInst create( Sql context, UniqueId instanceId, String ref_comp_name, String ref_comp_package, String ref_name, String ref_package, String m_extends, String m_key_letters ) throws XtumlException {
ModelInst newModelInst = new ModelInstImpl( context, instanceId, ref_comp_name, ref_comp_package, ref_name, ref_package, m_extends, m_key_letters );
if ( context.addInstance( newModelInst ) ) {
return newModelInst;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String ref_comp_name;
@Override
public String getComp_name() throws XtumlException {
checkLiving();
return ref_comp_name;
}
@Override
public void setComp_name(String ref_comp_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_comp_name, this.ref_comp_name)) {
final String oldValue = this.ref_comp_name;
this.ref_comp_name = ref_comp_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_comp_name", oldValue, this.ref_comp_name));
if ( !R434_participates_in_ClassRelationship().isEmpty() ) R434_participates_in_ClassRelationship().setComp_name( ref_comp_name );
if ( !R435_formalizes_ClassRelationship().isEmpty() ) R435_formalizes_ClassRelationship().setComp_name( ref_comp_name );
if ( !R780_created_by_Creation().isEmpty() ) R780_created_by_Creation().setComp_name( ref_comp_name );
if ( !R442_selects_instances_through_InstanceSelector().isEmpty() ) R442_selects_instances_through_InstanceSelector().setComp_name( ref_comp_name );
if ( !R410_data_abstracted_by_Attribute().isEmpty() ) R410_data_abstracted_by_Attribute().setComp_name( ref_comp_name );
if ( !R416_provides_Operation().isEmpty() ) R416_provides_Operation().setComp_name( ref_comp_name );
if ( !R449_extent_accessed_by_InstancePopulationSelector().isEmpty() ) R449_extent_accessed_by_InstancePopulationSelector().setComp_name( ref_comp_name );
if ( !R406_is_type_of_single_element_in_InstSet().isEmpty() ) R406_is_type_of_single_element_in_InstSet().setComp_name( ref_comp_name );
if ( !R3017_EventInstanceLoader().isEmpty() ) R3017_EventInstanceLoader().setComp_name( ref_comp_name );
if ( !R4750_behavior_modeled_by_StateMachine().isEmpty() ) R4750_behavior_modeled_by_StateMachine().setComp_name( ref_comp_name );
}
}
private String ref_comp_package;
@Override
public void setComp_package(String ref_comp_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_comp_package, this.ref_comp_package)) {
final String oldValue = this.ref_comp_package;
this.ref_comp_package = ref_comp_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_comp_package", oldValue, this.ref_comp_package));
if ( !R410_data_abstracted_by_Attribute().isEmpty() ) R410_data_abstracted_by_Attribute().setComp_package( ref_comp_package );
if ( !R449_extent_accessed_by_InstancePopulationSelector().isEmpty() ) R449_extent_accessed_by_InstancePopulationSelector().setComp_package( ref_comp_package );
if ( !R416_provides_Operation().isEmpty() ) R416_provides_Operation().setComp_package( ref_comp_package );
if ( !R4750_behavior_modeled_by_StateMachine().isEmpty() ) R4750_behavior_modeled_by_StateMachine().setComp_package( ref_comp_package );
if ( !R435_formalizes_ClassRelationship().isEmpty() ) R435_formalizes_ClassRelationship().setComp_package( ref_comp_package );
if ( !R406_is_type_of_single_element_in_InstSet().isEmpty() ) R406_is_type_of_single_element_in_InstSet().setComp_package( ref_comp_package );
if ( !R442_selects_instances_through_InstanceSelector().isEmpty() ) R442_selects_instances_through_InstanceSelector().setComp_package( ref_comp_package );
if ( !R3017_EventInstanceLoader().isEmpty() ) R3017_EventInstanceLoader().setComp_package( ref_comp_package );
if ( !R780_created_by_Creation().isEmpty() ) R780_created_by_Creation().setComp_package( ref_comp_package );
if ( !R434_participates_in_ClassRelationship().isEmpty() ) R434_participates_in_ClassRelationship().setComp_package( ref_comp_package );
}
}
@Override
public String getComp_package() throws XtumlException {
checkLiving();
return ref_comp_package;
}
private String ref_name;
@Override
public void setName(String ref_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_name, this.ref_name)) {
final String oldValue = this.ref_name;
this.ref_name = ref_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_name", oldValue, this.ref_name));
if ( !R410_data_abstracted_by_Attribute().isEmpty() ) R410_data_abstracted_by_Attribute().setClass_name( ref_name );
if ( !R4750_behavior_modeled_by_StateMachine().isEmpty() ) R4750_behavior_modeled_by_StateMachine().setClass_name( ref_name );
if ( !R780_created_by_Creation().isEmpty() ) R780_created_by_Creation().setClass_name( ref_name );
if ( !R435_formalizes_ClassRelationship().isEmpty() ) R435_formalizes_ClassRelationship().setForm_name( ref_name );
if ( !R434_participates_in_ClassRelationship().isEmpty() ) R434_participates_in_ClassRelationship().setPart_name( ref_name );
if ( !R406_is_type_of_single_element_in_InstSet().isEmpty() ) R406_is_type_of_single_element_in_InstSet().setClass_name( ref_name );
if ( !R449_extent_accessed_by_InstancePopulationSelector().isEmpty() ) R449_extent_accessed_by_InstancePopulationSelector().setClass_name( ref_name );
if ( !R3017_EventInstanceLoader().isEmpty() ) R3017_EventInstanceLoader().setClass_name( ref_name );
if ( !R442_selects_instances_through_InstanceSelector().isEmpty() ) R442_selects_instances_through_InstanceSelector().setClass_name( ref_name );
if ( !R416_provides_Operation().isEmpty() ) R416_provides_Operation().setClass_name( ref_name );
}
}
@Override
public String getName() throws XtumlException {
checkLiving();
return ref_name;
}
private String ref_package;
@Override
public String getPackage() throws XtumlException {
checkLiving();
return ref_package;
}
@Override
public void setPackage(String ref_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_package, this.ref_package)) {
final String oldValue = this.ref_package;
this.ref_package = ref_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_package", oldValue, this.ref_package));
if ( !R780_created_by_Creation().isEmpty() ) R780_created_by_Creation().setClass_package( ref_package );
if ( !R3017_EventInstanceLoader().isEmpty() ) R3017_EventInstanceLoader().setPackage( ref_package );
if ( !R442_selects_instances_through_InstanceSelector().isEmpty() ) R442_selects_instances_through_InstanceSelector().setClass_package( ref_package );
if ( !R435_formalizes_ClassRelationship().isEmpty() ) R435_formalizes_ClassRelationship().setForm_package( ref_package );
if ( !R434_participates_in_ClassRelationship().isEmpty() ) R434_participates_in_ClassRelationship().setPart_package( ref_package );
if ( !R416_provides_Operation().isEmpty() ) R416_provides_Operation().setClass_package( ref_package );
if ( !R410_data_abstracted_by_Attribute().isEmpty() ) R410_data_abstracted_by_Attribute().setClass_package( ref_package );
if ( !R406_is_type_of_single_element_in_InstSet().isEmpty() ) R406_is_type_of_single_element_in_InstSet().setClass_package( ref_package );
if ( !R4750_behavior_modeled_by_StateMachine().isEmpty() ) R4750_behavior_modeled_by_StateMachine().setPackage( ref_package );
if ( !R449_extent_accessed_by_InstancePopulationSelector().isEmpty() ) R449_extent_accessed_by_InstancePopulationSelector().setClass_package( ref_package );
}
}
private String m_extends;
@Override
public void setExtends(String m_extends) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_extends, this.m_extends)) {
final String oldValue = this.m_extends;
this.m_extends = m_extends;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_extends", oldValue, this.m_extends));
}
}
@Override
public String getExtends() throws XtumlException {
checkLiving();
return m_extends;
}
private String m_key_letters;
@Override
public String getKey_letters() throws XtumlException {
checkLiving();
return m_key_letters;
}
@Override
public void setKey_letters(String m_key_letters) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_key_letters, this.m_key_letters)) {
final String oldValue = this.m_key_letters;
this.m_key_letters = m_key_letters;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_key_letters", oldValue, this.m_key_letters));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getComp_name(), getComp_package(), getName(), getPackage());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public void render() throws XtumlException {
File file = self().R401_is_a_File();
String imports = file.getFormattedImports( ImportType.IMPL );
int index = 0;
final int _final2_index = index;
Attribute attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final2_index == ((Attribute)selected).getOrder());
while ( !attribute.isEmpty() ) {
attribute.render_initializer();
index = index + 1;
final int _final5_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final5_index == ((Attribute)selected).getOrder());
}
String attribute_initializers = context().T().body();
context().T().clear();
String attribute_list = "";
String attribute_invocation_list = "";
String attribute_initializers2 = "";
index = 0;
final int _final0_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final0_index == ((Attribute)selected).getOrder());
while ( !attribute.isEmpty() ) {
attribute_list = ( ( ( attribute_list + ", " ) + attribute.getType_reference_name() ) + " " ) + attribute.getName();
attribute_invocation_list = ( attribute_invocation_list + ", " ) + attribute.getName();
attribute_initializers2 = ( ( ( ( attribute_initializers2 + " this." ) + attribute.getName() ) + " = " ) + attribute.getName() ) + ";\n";
index = index + 1;
final int _final7_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final7_index == ((Attribute)selected).getOrder());
}
String id1_attributes = "";
String id2_attributes = "";
String id3_attributes = "";
String sep1 = "";
String sep2 = "";
String sep3 = "";
index = 0;
final int _final1_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final1_index == ((Attribute)selected).getOrder());
while ( !attribute.isEmpty() ) {
attribute.render();
AttributeAccessorSet accessors = attribute.R4510_value_accessed_through_AttributeAccessor();
AttributeAccessor accessor;
for ( Iterator _accessor_iter = accessors.elements().iterator(); _accessor_iter.hasNext(); ) {
accessor = _accessor_iter.next();
accessor.render();
if ( AttributeAccessorType.GETTER.equality(accessor.getAccessor_type()) && attribute.getIdentifier() % 2 == 1 ) {
id1_attributes = ( ( id1_attributes + sep1 ) + accessor.getName() ) + "()";
sep1 = ", ";
}
if ( AttributeAccessorType.GETTER.equality(accessor.getAccessor_type()) && ( attribute.getIdentifier() / 2 ) % 2 == 1 ) {
id2_attributes = ( ( id2_attributes + sep2 ) + accessor.getName() ) + "()";
sep2 = ", ";
}
if ( AttributeAccessorType.GETTER.equality(accessor.getAccessor_type()) && ( attribute.getIdentifier() / 4 ) % 2 == 1 ) {
id3_attributes = ( ( id3_attributes + sep3 ) + accessor.getName() ) + "()";
sep3 = ", ";
}
}
index = index + 1;
final int _final8_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final8_index == ((Attribute)selected).getOrder());
}
String attributes = context().T().body();
context().T().clear();
OperationSet class_ops = ((OperationSet)self().R416_provides_Operation().where(selected -> ((Operation)selected).getIs_class_based()));
if ( !class_ops.isEmpty() ) {
Operation operation;
for ( Iterator _operation_iter = class_ops.elements().iterator(); _operation_iter.hasNext(); ) {
operation = _operation_iter.next();
operation.render();
}
String operations = context().T().body();
context().T().clear();
context().T().include( "class/t.operations.static.java", operations, self() );
}
String static_operations = context().T().body();
context().T().clear();
OperationSet inst_ops = ((OperationSet)self().R416_provides_Operation().where(selected -> !((Operation)selected).getIs_class_based()));
Operation operation;
for ( Iterator _operation_iter = inst_ops.elements().iterator(); _operation_iter.hasNext(); ) {
operation = _operation_iter.next();
operation.render();
}
String operations = context().T().body();
context().T().clear();
String initial_state = "";
String initial_state2 = "";
String state_machine_initializer = "";
String state_machine_initializer2 = "";
String state_machine_decl = "";
StateMachine sm = self().R4750_behavior_modeled_by_StateMachine();
if ( !sm.isEmpty() ) {
initial_state = ", int initialState";
initial_state2 = ", initialState";
state_machine_initializer = ( " statemachine = new " + sm.getName() ) + "(this, context());\n";
state_machine_initializer2 = ( " statemachine = new " + sm.getName() ) + "(this, context(), initialState);\n";
sm.render_decl();
state_machine_decl = context().T().body();
context().T().clear();
}
EventSet evts = sm.R4752_declares_Event();
Event evt;
for ( Iterator _evt_iter = evts.elements().iterator(); _evt_iter.hasNext(); ) {
evt = _evt_iter.next();
evt.render();
}
String state_machine_events = context().T().body();
context().T().clear();
InstanceSelectorSet selects = self().R442_selects_instances_through_InstanceSelector();
String relationship_initializers = "";
InstanceSelector selector;
for ( Iterator _selector_iter = selects.elements().iterator(); _selector_iter.hasNext(); ) {
selector = _selector_iter.next();
selector.render();
Selector sel = selector.R445_is_a_Selector();
if ( sel.getMultiplicity().equality(Mult.MANY) ) {
relationship_initializers = ( ( ( ( relationship_initializers + " " ) + selector.getName() ) + "_set = new " ) + sel.getType_reference_name() ) + "Impl();\n";
}
else {
relationship_initializers = ( ( ( ( ( ( relationship_initializers + " " ) + selector.getName() ) + "_inst = " ) + sel.getType_reference_name() ) + "Impl.EMPTY_" ) + context().T().sub( "u_", sel.getType_reference_name() ) ) + ";\n";
}
}
String selectors = context().T().body();
context().T().clear();
index = 0;
final int _final3_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final3_index == ((Attribute)selected).getOrder());
while ( !attribute.isEmpty() ) {
AttributeAccessorSet accessors = attribute.R4510_value_accessed_through_AttributeAccessor();
AttributeAccessor accessor;
for ( Iterator _accessor_iter = accessors.elements().iterator(); _accessor_iter.hasNext(); ) {
accessor = _accessor_iter.next();
accessor.render_empty();
}
index = index + 1;
final int _final9_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final9_index == ((Attribute)selected).getOrder());
}
String empty_attributes = context().T().body();
context().T().clear();
for ( Iterator _operation_iter = inst_ops.elements().iterator(); _operation_iter.hasNext(); ) {
operation = _operation_iter.next();
operation.render_empty();
}
String empty_operations = context().T().body();
context().T().clear();
for ( Iterator _selector_iter = selects.elements().iterator(); _selector_iter.hasNext(); ) {
selector = _selector_iter.next();
selector.render_empty();
}
String empty_selectors = context().T().body();
context().T().clear();
context().T().include( "class/t.class.impl.java", attribute_initializers, attribute_initializers2, attribute_invocation_list, attribute_list, attributes, empty_attributes, empty_operations, empty_selectors, id1_attributes, id2_attributes, id3_attributes, imports, initial_state, initial_state2, operations, relationship_initializers, selectors, self(), state_machine_decl, state_machine_events, state_machine_initializer, state_machine_initializer2, static_operations );
context().T().emit( ( ( ( file.getPath() + "/impl/" ) + self().getName() ) + "Impl" ) + file.getExtension() );
context().T().clear();
imports = file.getFormattedImports( ImportType.INT );
index = 0;
final int _final4_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final4_index == ((Attribute)selected).getOrder());
while ( !attribute.isEmpty() ) {
AttributeAccessorSet accessors = attribute.R4510_value_accessed_through_AttributeAccessor();
AttributeAccessor accessor;
for ( Iterator _accessor_iter = accessors.elements().iterator(); _accessor_iter.hasNext(); ) {
accessor = _accessor_iter.next();
accessor.render_interface();
}
index = index + 1;
final int _final6_index = index;
attribute = self().R410_data_abstracted_by_Attribute().anyWhere(selected -> _final6_index == ((Attribute)selected).getOrder());
}
attributes = context().T().body();
context().T().clear();
for ( Iterator _operation_iter = inst_ops.elements().iterator(); _operation_iter.hasNext(); ) {
operation = _operation_iter.next();
operation.render_interface();
}
operations = context().T().body();
context().T().clear();
for ( Iterator _selector_iter = selects.elements().iterator(); _selector_iter.hasNext(); ) {
selector = _selector_iter.next();
selector.render_interface();
}
selectors = context().T().body();
context().T().clear();
context().T().include( "class/t.class.java", attributes, imports, operations, selectors, self() );
context().T().emit( ( ( file.getPath() + "/" ) + self().getName() ) + file.getExtension() );
context().T().clear();
}
// static operations
// events
// selections
private EventInstanceLoader R3017_EventInstanceLoader_inst;
@Override
public void setR3017_EventInstanceLoader( EventInstanceLoader inst ) {
R3017_EventInstanceLoader_inst = inst;
}
@Override
public EventInstanceLoader R3017_EventInstanceLoader() throws XtumlException {
return R3017_EventInstanceLoader_inst;
}
private File R401_is_a_File_inst;
@Override
public void setR401_is_a_File( File inst ) {
R401_is_a_File_inst = inst;
}
@Override
public File R401_is_a_File() throws XtumlException {
return R401_is_a_File_inst;
}
private InstSet R406_is_type_of_single_element_in_InstSet_inst;
@Override
public void setR406_is_type_of_single_element_in_InstSet( InstSet inst ) {
R406_is_type_of_single_element_in_InstSet_inst = inst;
}
@Override
public InstSet R406_is_type_of_single_element_in_InstSet() throws XtumlException {
return R406_is_type_of_single_element_in_InstSet_inst;
}
private Type R407_is_a_Type_inst;
@Override
public void setR407_is_a_Type( Type inst ) {
R407_is_a_Type_inst = inst;
}
@Override
public Type R407_is_a_Type() throws XtumlException {
return R407_is_a_Type_inst;
}
private ComponentDefinition R408_forms_instance_population_of_ComponentDefinition_inst;
@Override
public void setR408_forms_instance_population_of_ComponentDefinition( ComponentDefinition inst ) {
R408_forms_instance_population_of_ComponentDefinition_inst = inst;
}
@Override
public ComponentDefinition R408_forms_instance_population_of_ComponentDefinition() throws XtumlException {
return R408_forms_instance_population_of_ComponentDefinition_inst;
}
private ModelClass R409_ModelClass_inst;
@Override
public void setR409_ModelClass( ModelClass inst ) {
R409_ModelClass_inst = inst;
}
@Override
public ModelClass R409_ModelClass() throws XtumlException {
return R409_ModelClass_inst;
}
private AttributeSet R410_data_abstracted_by_Attribute_set;
@Override
public void addR410_data_abstracted_by_Attribute( Attribute inst ) {
R410_data_abstracted_by_Attribute_set.add(inst);
}
@Override
public void removeR410_data_abstracted_by_Attribute( Attribute inst ) {
R410_data_abstracted_by_Attribute_set.remove(inst);
}
@Override
public AttributeSet R410_data_abstracted_by_Attribute() throws XtumlException {
return R410_data_abstracted_by_Attribute_set;
}
private OperationSet R416_provides_Operation_set;
@Override
public void addR416_provides_Operation( Operation inst ) {
R416_provides_Operation_set.add(inst);
}
@Override
public void removeR416_provides_Operation( Operation inst ) {
R416_provides_Operation_set.remove(inst);
}
@Override
public OperationSet R416_provides_Operation() throws XtumlException {
return R416_provides_Operation_set;
}
private ClassRelationshipSet R434_participates_in_ClassRelationship_set;
@Override
public void addR434_participates_in_ClassRelationship( ClassRelationship inst ) {
R434_participates_in_ClassRelationship_set.add(inst);
}
@Override
public void removeR434_participates_in_ClassRelationship( ClassRelationship inst ) {
R434_participates_in_ClassRelationship_set.remove(inst);
}
@Override
public ClassRelationshipSet R434_participates_in_ClassRelationship() throws XtumlException {
return R434_participates_in_ClassRelationship_set;
}
private ClassRelationshipSet R435_formalizes_ClassRelationship_set;
@Override
public void addR435_formalizes_ClassRelationship( ClassRelationship inst ) {
R435_formalizes_ClassRelationship_set.add(inst);
}
@Override
public void removeR435_formalizes_ClassRelationship( ClassRelationship inst ) {
R435_formalizes_ClassRelationship_set.remove(inst);
}
@Override
public ClassRelationshipSet R435_formalizes_ClassRelationship() throws XtumlException {
return R435_formalizes_ClassRelationship_set;
}
private InstanceSelectorSet R442_selects_instances_through_InstanceSelector_set;
@Override
public void addR442_selects_instances_through_InstanceSelector( InstanceSelector inst ) {
R442_selects_instances_through_InstanceSelector_set.add(inst);
}
@Override
public void removeR442_selects_instances_through_InstanceSelector( InstanceSelector inst ) {
R442_selects_instances_through_InstanceSelector_set.remove(inst);
}
@Override
public InstanceSelectorSet R442_selects_instances_through_InstanceSelector() throws XtumlException {
return R442_selects_instances_through_InstanceSelector_set;
}
private InstancePopulationSelector R449_extent_accessed_by_InstancePopulationSelector_inst;
@Override
public void setR449_extent_accessed_by_InstancePopulationSelector( InstancePopulationSelector inst ) {
R449_extent_accessed_by_InstancePopulationSelector_inst = inst;
}
@Override
public InstancePopulationSelector R449_extent_accessed_by_InstancePopulationSelector() throws XtumlException {
return R449_extent_accessed_by_InstancePopulationSelector_inst;
}
private StateMachine R4750_behavior_modeled_by_StateMachine_inst;
@Override
public void setR4750_behavior_modeled_by_StateMachine( StateMachine inst ) {
R4750_behavior_modeled_by_StateMachine_inst = inst;
}
@Override
public StateMachine R4750_behavior_modeled_by_StateMachine() throws XtumlException {
return R4750_behavior_modeled_by_StateMachine_inst;
}
private CreationSet R780_created_by_Creation_set;
@Override
public void addR780_created_by_Creation( Creation inst ) {
R780_created_by_Creation_set.add(inst);
}
@Override
public void removeR780_created_by_Creation( Creation inst ) {
R780_created_by_Creation_set.remove(inst);
}
@Override
public CreationSet R780_created_by_Creation() throws XtumlException {
return R780_created_by_Creation_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public ModelInst self() {
return this;
}
@Override
public ModelInst oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_MODELINST;
}
}
class EmptyModelInst extends ModelInstance implements ModelInst {
// attributes
public String getComp_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setComp_name( String ref_comp_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setComp_package( String ref_comp_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getComp_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String ref_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getPackage() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setPackage( String ref_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setExtends( String m_extends ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getExtends() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getKey_letters() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setKey_letters( String m_key_letters ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
public void render() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public EventInstanceLoader R3017_EventInstanceLoader() {
return EventInstanceLoaderImpl.EMPTY_EVENTINSTANCELOADER;
}
@Override
public File R401_is_a_File() {
return FileImpl.EMPTY_FILE;
}
@Override
public InstSet R406_is_type_of_single_element_in_InstSet() {
return InstSetImpl.EMPTY_INSTSET;
}
@Override
public Type R407_is_a_Type() {
return TypeImpl.EMPTY_TYPE;
}
@Override
public ComponentDefinition R408_forms_instance_population_of_ComponentDefinition() {
return ComponentDefinitionImpl.EMPTY_COMPONENTDEFINITION;
}
@Override
public ModelClass R409_ModelClass() {
return ModelClassImpl.EMPTY_MODELCLASS;
}
@Override
public AttributeSet R410_data_abstracted_by_Attribute() {
return (new AttributeSetImpl());
}
@Override
public OperationSet R416_provides_Operation() {
return (new OperationSetImpl());
}
@Override
public ClassRelationshipSet R434_participates_in_ClassRelationship() {
return (new ClassRelationshipSetImpl());
}
@Override
public ClassRelationshipSet R435_formalizes_ClassRelationship() {
return (new ClassRelationshipSetImpl());
}
@Override
public InstanceSelectorSet R442_selects_instances_through_InstanceSelector() {
return (new InstanceSelectorSetImpl());
}
@Override
public InstancePopulationSelector R449_extent_accessed_by_InstancePopulationSelector() {
return InstancePopulationSelectorImpl.EMPTY_INSTANCEPOPULATIONSELECTOR;
}
@Override
public StateMachine R4750_behavior_modeled_by_StateMachine() {
return StateMachineImpl.EMPTY_STATEMACHINE;
}
@Override
public CreationSet R780_created_by_Creation() {
return (new CreationSetImpl());
}
@Override
public String getKeyLetters() {
return ModelInstImpl.KEY_LETTERS;
}
@Override
public ModelInst self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public ModelInst oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return ModelInstImpl.EMPTY_MODELINST;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy