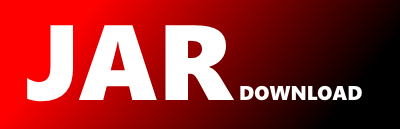
io.ciera.tool.sql.architecture.component.impl.FunctionImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.architecture.component.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.architecture.component.ComponentDefinition;
import io.ciera.tool.sql.architecture.component.ComponentDefinitionSet;
import io.ciera.tool.sql.architecture.component.Function;
import io.ciera.tool.sql.architecture.component.impl.ComponentDefinitionImpl;
import io.ciera.tool.sql.architecture.component.impl.ComponentDefinitionSetImpl;
import io.ciera.tool.sql.architecture.invocable.InvocableObject;
import io.ciera.tool.sql.architecture.invocable.impl.InvocableObjectImpl;
import io.ciera.tool.sql.architecture.type.TypeReference;
public class FunctionImpl extends ModelInstance implements Function {
public static final String KEY_LETTERS = "Function";
public static final Function EMPTY_FUNCTION = new EmptyFunction();
private Sql context;
// constructors
private FunctionImpl( Sql context ) {
this.context = context;
ref_comp_name = "";
ref_comp_package = "";
ref_name = "";
R405_can_execute_synchronously_within_ComponentDefinition_inst = ComponentDefinitionImpl.EMPTY_COMPONENTDEFINITION;
R427_is_a_InvocableObject_inst = InvocableObjectImpl.EMPTY_INVOCABLEOBJECT;
R4561_initializes_ComponentDefinition_set = new ComponentDefinitionSetImpl();
}
private FunctionImpl( Sql context, UniqueId instanceId, String ref_comp_name, String ref_comp_package, String ref_name ) {
super(instanceId);
this.context = context;
this.ref_comp_name = ref_comp_name;
this.ref_comp_package = ref_comp_package;
this.ref_name = ref_name;
R405_can_execute_synchronously_within_ComponentDefinition_inst = ComponentDefinitionImpl.EMPTY_COMPONENTDEFINITION;
R427_is_a_InvocableObject_inst = InvocableObjectImpl.EMPTY_INVOCABLEOBJECT;
R4561_initializes_ComponentDefinition_set = new ComponentDefinitionSetImpl();
}
public static Function create( Sql context ) throws XtumlException {
Function newFunction = new FunctionImpl( context );
if ( context.addInstance( newFunction ) ) {
newFunction.getRunContext().addChange(new InstanceCreatedDelta(newFunction, KEY_LETTERS));
return newFunction;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Function create( Sql context, String ref_comp_name, String ref_comp_package, String ref_name ) throws XtumlException {
return create(context, UniqueId.random(), ref_comp_name, ref_comp_package, ref_name);
}
public static Function create( Sql context, UniqueId instanceId, String ref_comp_name, String ref_comp_package, String ref_name ) throws XtumlException {
Function newFunction = new FunctionImpl( context, instanceId, ref_comp_name, ref_comp_package, ref_name );
if ( context.addInstance( newFunction ) ) {
return newFunction;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String ref_comp_name;
@Override
public void setComp_name(String ref_comp_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_comp_name, this.ref_comp_name)) {
final String oldValue = this.ref_comp_name;
this.ref_comp_name = ref_comp_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_comp_name", oldValue, this.ref_comp_name));
if ( !R4561_initializes_ComponentDefinition().isEmpty() ) R4561_initializes_ComponentDefinition().setName( ref_comp_name );
}
}
@Override
public String getComp_name() throws XtumlException {
checkLiving();
return ref_comp_name;
}
private String ref_comp_package;
@Override
public void setComp_package(String ref_comp_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_comp_package, this.ref_comp_package)) {
final String oldValue = this.ref_comp_package;
this.ref_comp_package = ref_comp_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_comp_package", oldValue, this.ref_comp_package));
if ( !R4561_initializes_ComponentDefinition().isEmpty() ) R4561_initializes_ComponentDefinition().setPackage( ref_comp_package );
}
}
@Override
public String getComp_package() throws XtumlException {
checkLiving();
return ref_comp_package;
}
private String ref_name;
@Override
public String getName() throws XtumlException {
checkLiving();
return ref_name;
}
@Override
public void setName(String ref_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_name, this.ref_name)) {
final String oldValue = this.ref_name;
this.ref_name = ref_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_name", oldValue, this.ref_name));
if ( !R4561_initializes_ComponentDefinition().isEmpty() ) R4561_initializes_ComponentDefinition().setInit_function_name( ref_name );
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getComp_name(), getComp_package(), getName());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public void render() throws XtumlException {
TypeReference type = self().R427_is_a_InvocableObject().R428_return_value_is_typed_by_TypeReference();
String type_name = type.getType_reference_name();
context().T().push_buffer();
InvocableObject invocable = self().R427_is_a_InvocableObject();
String parameter_list = invocable.parameter_list();
String body = invocable.body();
context().T().pop_buffer();
context().T().include( "component/t.function.java", body, parameter_list, self(), type_name );
}
// static operations
// events
// selections
private ComponentDefinition R405_can_execute_synchronously_within_ComponentDefinition_inst;
@Override
public void setR405_can_execute_synchronously_within_ComponentDefinition( ComponentDefinition inst ) {
R405_can_execute_synchronously_within_ComponentDefinition_inst = inst;
}
@Override
public ComponentDefinition R405_can_execute_synchronously_within_ComponentDefinition() throws XtumlException {
return R405_can_execute_synchronously_within_ComponentDefinition_inst;
}
private InvocableObject R427_is_a_InvocableObject_inst;
@Override
public void setR427_is_a_InvocableObject( InvocableObject inst ) {
R427_is_a_InvocableObject_inst = inst;
}
@Override
public InvocableObject R427_is_a_InvocableObject() throws XtumlException {
return R427_is_a_InvocableObject_inst;
}
private ComponentDefinitionSet R4561_initializes_ComponentDefinition_set;
@Override
public void addR4561_initializes_ComponentDefinition( ComponentDefinition inst ) {
R4561_initializes_ComponentDefinition_set.add(inst);
}
@Override
public void removeR4561_initializes_ComponentDefinition( ComponentDefinition inst ) {
R4561_initializes_ComponentDefinition_set.remove(inst);
}
@Override
public ComponentDefinitionSet R4561_initializes_ComponentDefinition() throws XtumlException {
return R4561_initializes_ComponentDefinition_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Function self() {
return this;
}
@Override
public Function oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_FUNCTION;
}
}
class EmptyFunction extends ModelInstance implements Function {
// attributes
public void setComp_name( String ref_comp_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getComp_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setComp_package( String ref_comp_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getComp_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String ref_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
public void render() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public ComponentDefinition R405_can_execute_synchronously_within_ComponentDefinition() {
return ComponentDefinitionImpl.EMPTY_COMPONENTDEFINITION;
}
@Override
public InvocableObject R427_is_a_InvocableObject() {
return InvocableObjectImpl.EMPTY_INVOCABLEOBJECT;
}
@Override
public ComponentDefinitionSet R4561_initializes_ComponentDefinition() {
return (new ComponentDefinitionSetImpl());
}
@Override
public String getKeyLetters() {
return FunctionImpl.KEY_LETTERS;
}
@Override
public Function self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Function oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return FunctionImpl.EMPTY_FUNCTION;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy