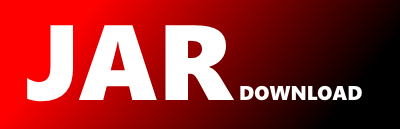
io.ciera.tool.sql.architecture.expression.ExpressionSet Maven / Gradle / Ivy
package io.ciera.tool.sql.architecture.expression;
import io.ciera.runtime.summit.classes.IInstanceSet;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.tool.sql.architecture.expression.ActualParameterSet;
import io.ciera.tool.sql.architecture.expression.AnySet;
import io.ciera.tool.sql.architecture.expression.ArrayElementReferenceSet;
import io.ciera.tool.sql.architecture.expression.ArrayLengthAccessSet;
import io.ciera.tool.sql.architecture.expression.AttributeAccessSet;
import io.ciera.tool.sql.architecture.expression.BinaryOperationSet;
import io.ciera.tool.sql.architecture.expression.ConstantReferenceSet;
import io.ciera.tool.sql.architecture.expression.CreationSet;
import io.ciera.tool.sql.architecture.expression.EnumeratorReferenceSet;
import io.ciera.tool.sql.architecture.expression.EventCreationSet;
import io.ciera.tool.sql.architecture.expression.InvocationSet;
import io.ciera.tool.sql.architecture.expression.LiteralSet;
import io.ciera.tool.sql.architecture.expression.MemberReferenceSet;
import io.ciera.tool.sql.architecture.expression.NamedReferenceSet;
import io.ciera.tool.sql.architecture.expression.ParameterReferenceSet;
import io.ciera.tool.sql.architecture.expression.ParenthesizedExpressionSet;
import io.ciera.tool.sql.architecture.expression.PromotionSet;
import io.ciera.tool.sql.architecture.expression.SelectRelatedSet;
import io.ciera.tool.sql.architecture.expression.SelectSet;
import io.ciera.tool.sql.architecture.expression.SelectedSet;
import io.ciera.tool.sql.architecture.expression.UnaryOperationSet;
import io.ciera.tool.sql.architecture.expression.VariableReferenceSet;
import io.ciera.tool.sql.architecture.expression.WhereSet;
import io.ciera.tool.sql.architecture.statement.DeleteSmtSet;
import io.ciera.tool.sql.architecture.statement.ExpressionAsStatementSet;
import io.ciera.tool.sql.architecture.statement.ForSmtSet;
import io.ciera.tool.sql.architecture.statement.GenerateSet;
import io.ciera.tool.sql.architecture.statement.IfSmtSet;
import io.ciera.tool.sql.architecture.statement.RelateSmtSet;
import io.ciera.tool.sql.architecture.statement.ReturnSmtSet;
import io.ciera.tool.sql.architecture.statement.StatementSet;
import io.ciera.tool.sql.architecture.statement.UnrelateSmtSet;
import io.ciera.tool.sql.architecture.statement.WhileSmtSet;
import io.ciera.tool.sql.architecture.type.TypeReferenceSet;
public interface ExpressionSet extends IInstanceSet {
// attributes
public void setBody_name( String ref_body_name ) throws XtumlException;
public void setParent_package( String ref_parent_package ) throws XtumlException;
public void setParent_name( String ref_parent_name ) throws XtumlException;
public void setType_reference_name( String ref_type_reference_name ) throws XtumlException;
public void setType_package( String ref_type_package ) throws XtumlException;
public void setStatement_number( String ref_statement_number ) throws XtumlException;
public void setBlock_number( String ref_block_number ) throws XtumlException;
public void setType_name( String ref_type_name ) throws XtumlException;
public void setExpression_number( String m_expression_number ) throws XtumlException;
// selections
public ArrayElementReferenceSet R3900_is_root_for_ArrayElementReference() throws XtumlException;
public ArrayElementReferenceSet R3901_is_index_for_ArrayElementReference() throws XtumlException;
public EventCreationSet R3902_EventCreation() throws XtumlException;
public EventCreationSet R3903_EventCreation() throws XtumlException;
public PromotionSet R3907_promoted_by_Promotion() throws XtumlException;
public DeleteSmtSet R462_is_deleted_by_DeleteSmt() throws XtumlException;
public IfSmtSet R471_determines_execution_of_blocks_for_IfSmt() throws XtumlException;
public WhileSmtSet R472_determines_execution_of_block_for_WhileSmt() throws XtumlException;
public ReturnSmtSet R473_defines_return_value_for_ReturnSmt() throws XtumlException;
public ExpressionAsStatementSet R476_executed_by_ExpressionAsStatement() throws XtumlException;
public ForSmtSet R478_is_iterated_by_ForSmt() throws XtumlException;
public RelateSmtSet R479_related_to_participant_by_RelateSmt() throws XtumlException;
public RelateSmtSet R480_related_to_formalizer_by_RelateSmt() throws XtumlException;
public UnrelateSmtSet R482_unrelated_from_formalizer_by_UnrelateSmt() throws XtumlException;
public UnrelateSmtSet R483_unrelated_from_participant_by_UnrelateSmt() throws XtumlException;
public GenerateSet R486_Generate() throws XtumlException;
public StatementSet R775_expressed_within_Statement() throws XtumlException;
public AnySet R776_is_a_Any() throws XtumlException;
public ArrayElementReferenceSet R776_is_a_ArrayElementReference() throws XtumlException;
public ArrayLengthAccessSet R776_is_a_ArrayLengthAccess() throws XtumlException;
public AttributeAccessSet R776_is_a_AttributeAccess() throws XtumlException;
public BinaryOperationSet R776_is_a_BinaryOperation() throws XtumlException;
public ConstantReferenceSet R776_is_a_ConstantReference() throws XtumlException;
public CreationSet R776_is_a_Creation() throws XtumlException;
public EnumeratorReferenceSet R776_is_a_EnumeratorReference() throws XtumlException;
public EventCreationSet R776_is_a_EventCreation() throws XtumlException;
public InvocationSet R776_is_a_Invocation() throws XtumlException;
public LiteralSet R776_is_a_Literal() throws XtumlException;
public MemberReferenceSet R776_is_a_MemberReference() throws XtumlException;
public NamedReferenceSet R776_is_a_NamedReference() throws XtumlException;
public ParameterReferenceSet R776_is_a_ParameterReference() throws XtumlException;
public ParenthesizedExpressionSet R776_is_a_ParenthesizedExpression() throws XtumlException;
public PromotionSet R776_is_a_Promotion() throws XtumlException;
public SelectSet R776_is_a_Select() throws XtumlException;
public SelectedSet R776_is_a_Selected() throws XtumlException;
public UnaryOperationSet R776_is_a_UnaryOperation() throws XtumlException;
public VariableReferenceSet R776_is_a_VariableReference() throws XtumlException;
public WhereSet R776_is_a_Where() throws XtumlException;
public UnaryOperationSet R777_is_single_operand_for_UnaryOperation() throws XtumlException;
public BinaryOperationSet R778_is_right_operand_for_BinaryOperation() throws XtumlException;
public BinaryOperationSet R779_is_left_operand_for_BinaryOperation() throws XtumlException;
public AnySet R783_is_basis_for_Any() throws XtumlException;
public ParenthesizedExpressionSet R784_is_wrapped_in_parentheses_by_ParenthesizedExpression() throws XtumlException;
public AttributeAccessSet R785_is_used_as_basis_for_AttributeAccess() throws XtumlException;
public MemberReferenceSet R787_is_used_as_basis_for_MemberReference() throws XtumlException;
public WhereSet R790_filtered_by_Where() throws XtumlException;
public SelectRelatedSet R791_is_basis_for_SelectRelated() throws XtumlException;
public ActualParameterSet R794_defines_value_for_ActualParameter() throws XtumlException;
public TypeReferenceSet R795_expresses_value_of_TypeReference() throws XtumlException;
public WhereSet R796_defines_condtion_for_Where() throws XtumlException;
public InvocationSet R798_is_used_as_basis_for_Invocation() throws XtumlException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy