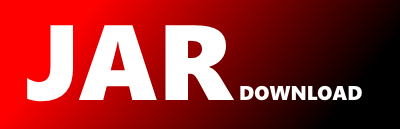
io.ciera.tool.sql.architecture.expression.impl.BinaryOperationImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.architecture.expression.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.architecture.classes.AttributeAccessor;
import io.ciera.tool.sql.architecture.expression.AttributeAccess;
import io.ciera.tool.sql.architecture.expression.BinaryOperation;
import io.ciera.tool.sql.architecture.expression.Expression;
import io.ciera.tool.sql.architecture.expression.impl.ExpressionImpl;
import types.AttributeAccessorType;
public class BinaryOperationImpl extends ModelInstance implements BinaryOperation {
public static final String KEY_LETTERS = "BinaryOperation";
public static final BinaryOperation EMPTY_BINARYOPERATION = new EmptyBinaryOperation();
private Sql context;
// constructors
private BinaryOperationImpl( Sql context ) {
this.context = context;
ref_parent_name = "";
ref_parent_package = "";
ref_body_name = "";
ref_block_number = "";
ref_statement_number = "";
ref_expression_number = "";
ref_left_expression_number = "";
ref_right_expression_number = "";
m_operator = "";
m_invocation = false;
m_util = false;
m_cast = "";
R776_is_a_Expression_inst = ExpressionImpl.EMPTY_EXPRESSION;
R778_has_right_Expression_inst = ExpressionImpl.EMPTY_EXPRESSION;
R779_has_left_Expression_inst = ExpressionImpl.EMPTY_EXPRESSION;
}
private BinaryOperationImpl( Sql context, UniqueId instanceId, String ref_parent_name, String ref_parent_package, String ref_body_name, String ref_block_number, String ref_statement_number, String ref_expression_number, String ref_left_expression_number, String ref_right_expression_number, String m_operator, boolean m_invocation, boolean m_util, String m_cast ) {
super(instanceId);
this.context = context;
this.ref_parent_name = ref_parent_name;
this.ref_parent_package = ref_parent_package;
this.ref_body_name = ref_body_name;
this.ref_block_number = ref_block_number;
this.ref_statement_number = ref_statement_number;
this.ref_expression_number = ref_expression_number;
this.ref_left_expression_number = ref_left_expression_number;
this.ref_right_expression_number = ref_right_expression_number;
this.m_operator = m_operator;
this.m_invocation = m_invocation;
this.m_util = m_util;
this.m_cast = m_cast;
R776_is_a_Expression_inst = ExpressionImpl.EMPTY_EXPRESSION;
R778_has_right_Expression_inst = ExpressionImpl.EMPTY_EXPRESSION;
R779_has_left_Expression_inst = ExpressionImpl.EMPTY_EXPRESSION;
}
public static BinaryOperation create( Sql context ) throws XtumlException {
BinaryOperation newBinaryOperation = new BinaryOperationImpl( context );
if ( context.addInstance( newBinaryOperation ) ) {
newBinaryOperation.getRunContext().addChange(new InstanceCreatedDelta(newBinaryOperation, KEY_LETTERS));
return newBinaryOperation;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static BinaryOperation create( Sql context, String ref_parent_name, String ref_parent_package, String ref_body_name, String ref_block_number, String ref_statement_number, String ref_expression_number, String ref_left_expression_number, String ref_right_expression_number, String m_operator, boolean m_invocation, boolean m_util, String m_cast ) throws XtumlException {
return create(context, UniqueId.random(), ref_parent_name, ref_parent_package, ref_body_name, ref_block_number, ref_statement_number, ref_expression_number, ref_left_expression_number, ref_right_expression_number, m_operator, m_invocation, m_util, m_cast);
}
public static BinaryOperation create( Sql context, UniqueId instanceId, String ref_parent_name, String ref_parent_package, String ref_body_name, String ref_block_number, String ref_statement_number, String ref_expression_number, String ref_left_expression_number, String ref_right_expression_number, String m_operator, boolean m_invocation, boolean m_util, String m_cast ) throws XtumlException {
BinaryOperation newBinaryOperation = new BinaryOperationImpl( context, instanceId, ref_parent_name, ref_parent_package, ref_body_name, ref_block_number, ref_statement_number, ref_expression_number, ref_left_expression_number, ref_right_expression_number, m_operator, m_invocation, m_util, m_cast );
if ( context.addInstance( newBinaryOperation ) ) {
return newBinaryOperation;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String ref_parent_name;
@Override
public String getParent_name() throws XtumlException {
checkLiving();
return ref_parent_name;
}
@Override
public void setParent_name(String ref_parent_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_parent_name, this.ref_parent_name)) {
final String oldValue = this.ref_parent_name;
this.ref_parent_name = ref_parent_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_parent_name", oldValue, this.ref_parent_name));
}
}
private String ref_parent_package;
@Override
public String getParent_package() throws XtumlException {
checkLiving();
return ref_parent_package;
}
@Override
public void setParent_package(String ref_parent_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_parent_package, this.ref_parent_package)) {
final String oldValue = this.ref_parent_package;
this.ref_parent_package = ref_parent_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_parent_package", oldValue, this.ref_parent_package));
}
}
private String ref_body_name;
@Override
public void setBody_name(String ref_body_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_body_name, this.ref_body_name)) {
final String oldValue = this.ref_body_name;
this.ref_body_name = ref_body_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_body_name", oldValue, this.ref_body_name));
}
}
@Override
public String getBody_name() throws XtumlException {
checkLiving();
return ref_body_name;
}
private String ref_block_number;
@Override
public void setBlock_number(String ref_block_number) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_block_number, this.ref_block_number)) {
final String oldValue = this.ref_block_number;
this.ref_block_number = ref_block_number;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_block_number", oldValue, this.ref_block_number));
}
}
@Override
public String getBlock_number() throws XtumlException {
checkLiving();
return ref_block_number;
}
private String ref_statement_number;
@Override
public void setStatement_number(String ref_statement_number) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_statement_number, this.ref_statement_number)) {
final String oldValue = this.ref_statement_number;
this.ref_statement_number = ref_statement_number;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_statement_number", oldValue, this.ref_statement_number));
}
}
@Override
public String getStatement_number() throws XtumlException {
checkLiving();
return ref_statement_number;
}
private String ref_expression_number;
@Override
public void setExpression_number(String ref_expression_number) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_expression_number, this.ref_expression_number)) {
final String oldValue = this.ref_expression_number;
this.ref_expression_number = ref_expression_number;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_expression_number", oldValue, this.ref_expression_number));
}
}
@Override
public String getExpression_number() throws XtumlException {
checkLiving();
return ref_expression_number;
}
private String ref_left_expression_number;
@Override
public void setLeft_expression_number(String ref_left_expression_number) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_left_expression_number, this.ref_left_expression_number)) {
final String oldValue = this.ref_left_expression_number;
this.ref_left_expression_number = ref_left_expression_number;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_left_expression_number", oldValue, this.ref_left_expression_number));
}
}
@Override
public String getLeft_expression_number() throws XtumlException {
checkLiving();
return ref_left_expression_number;
}
private String ref_right_expression_number;
@Override
public void setRight_expression_number(String ref_right_expression_number) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_right_expression_number, this.ref_right_expression_number)) {
final String oldValue = this.ref_right_expression_number;
this.ref_right_expression_number = ref_right_expression_number;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_right_expression_number", oldValue, this.ref_right_expression_number));
}
}
@Override
public String getRight_expression_number() throws XtumlException {
checkLiving();
return ref_right_expression_number;
}
private String m_operator;
@Override
public void setOperator(String m_operator) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_operator, this.m_operator)) {
final String oldValue = this.m_operator;
this.m_operator = m_operator;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_operator", oldValue, this.m_operator));
}
}
@Override
public String getOperator() throws XtumlException {
checkLiving();
return m_operator;
}
private boolean m_invocation;
@Override
public void setInvocation(boolean m_invocation) throws XtumlException {
checkLiving();
if (m_invocation != this.m_invocation) {
final boolean oldValue = this.m_invocation;
this.m_invocation = m_invocation;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_invocation", oldValue, this.m_invocation));
}
}
@Override
public boolean getInvocation() throws XtumlException {
checkLiving();
return m_invocation;
}
private boolean m_util;
@Override
public void setUtil(boolean m_util) throws XtumlException {
checkLiving();
if (m_util != this.m_util) {
final boolean oldValue = this.m_util;
this.m_util = m_util;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_util", oldValue, this.m_util));
}
}
@Override
public boolean getUtil() throws XtumlException {
checkLiving();
return m_util;
}
private String m_cast;
@Override
public String getCast() throws XtumlException {
checkLiving();
return m_cast;
}
@Override
public void setCast(String m_cast) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_cast, this.m_cast)) {
final String oldValue = this.m_cast;
this.m_cast = m_cast;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_cast", oldValue, this.m_cast));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getParent_name(), getParent_package(), getBody_name(), getBlock_number(), getStatement_number(), getExpression_number());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public void render() throws XtumlException {
context().T().push_buffer();
Expression left_operand = self().R779_has_left_Expression();
String left_operand_body = "";
String operator = self().getOperator();
if ( StringUtil.equality("=", self().getOperator()) ) {
AttributeAccess attr_access = left_operand.R776_is_a_AttributeAccess();
if ( !attr_access.isEmpty() ) {
AttributeAccessor accessor = attr_access.R788_refers_to_Attribute().R4510_value_accessed_through_AttributeAccessor().anyWhere(selected -> ((AttributeAccessor)selected).getAccessor_type().equality(AttributeAccessorType.SETTER));
operator = accessor.getName();
self().setInvocation(true);
left_operand = attr_access.R785_refers_to_attribute_of_instance_expressed_by_Expression();
}
left_operand.render();
left_operand_body = context().T().body();
context().T().clear();
}
else {
left_operand.render();
left_operand_body = context().T().body();
context().T().clear();
}
Expression right_operand = self().R778_has_right_Expression();
right_operand.render();
String right_operand_body = context().T().body();
context().T().pop_buffer();
context().T().include( "expression/t.binaryoperation.java", left_operand_body, operator, right_operand_body, self() );
}
// static operations
// events
// selections
private Expression R776_is_a_Expression_inst;
@Override
public void setR776_is_a_Expression( Expression inst ) {
R776_is_a_Expression_inst = inst;
}
@Override
public Expression R776_is_a_Expression() throws XtumlException {
return R776_is_a_Expression_inst;
}
private Expression R778_has_right_Expression_inst;
@Override
public void setR778_has_right_Expression( Expression inst ) {
R778_has_right_Expression_inst = inst;
}
@Override
public Expression R778_has_right_Expression() throws XtumlException {
return R778_has_right_Expression_inst;
}
private Expression R779_has_left_Expression_inst;
@Override
public void setR779_has_left_Expression( Expression inst ) {
R779_has_left_Expression_inst = inst;
}
@Override
public Expression R779_has_left_Expression() throws XtumlException {
return R779_has_left_Expression_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public BinaryOperation self() {
return this;
}
@Override
public BinaryOperation oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_BINARYOPERATION;
}
}
class EmptyBinaryOperation extends ModelInstance implements BinaryOperation {
// attributes
public String getParent_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setParent_name( String ref_parent_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getParent_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setParent_package( String ref_parent_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setBody_name( String ref_body_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getBody_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setBlock_number( String ref_block_number ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getBlock_number() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setStatement_number( String ref_statement_number ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getStatement_number() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setExpression_number( String ref_expression_number ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getExpression_number() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLeft_expression_number( String ref_left_expression_number ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getLeft_expression_number() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRight_expression_number( String ref_right_expression_number ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getRight_expression_number() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setOperator( String m_operator ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getOperator() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setInvocation( boolean m_invocation ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getInvocation() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setUtil( boolean m_util ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getUtil() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getCast() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCast( String m_cast ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
public void render() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public Expression R776_is_a_Expression() {
return ExpressionImpl.EMPTY_EXPRESSION;
}
@Override
public Expression R778_has_right_Expression() {
return ExpressionImpl.EMPTY_EXPRESSION;
}
@Override
public Expression R779_has_left_Expression() {
return ExpressionImpl.EMPTY_EXPRESSION;
}
@Override
public String getKeyLetters() {
return BinaryOperationImpl.KEY_LETTERS;
}
@Override
public BinaryOperation self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public BinaryOperation oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return BinaryOperationImpl.EMPTY_BINARYOPERATION;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy