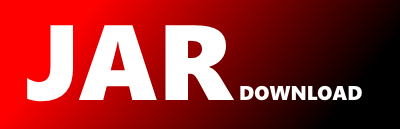
io.ciera.tool.sql.architecture.interfaces.impl.IfaceImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.architecture.interfaces.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.architecture.file.File;
import io.ciera.tool.sql.architecture.file.impl.FileImpl;
import io.ciera.tool.sql.architecture.interfaces.Iface;
import io.ciera.tool.sql.architecture.interfaces.Message;
import io.ciera.tool.sql.architecture.interfaces.MessageSet;
import io.ciera.tool.sql.architecture.interfaces.Port;
import io.ciera.tool.sql.architecture.interfaces.PortSet;
import io.ciera.tool.sql.architecture.interfaces.impl.MessageSetImpl;
import io.ciera.tool.sql.architecture.interfaces.impl.PortSetImpl;
import io.ciera.tool.sql.ooaofooa.component.C_I;
import io.ciera.tool.sql.ooaofooa.component.impl.C_IImpl;
import java.util.Iterator;
import types.ImportType;
public class IfaceImpl extends ModelInstance implements Iface {
public static final String KEY_LETTERS = "Interface";
public static final Iface EMPTY_IFACE = new EmptyIface();
private Sql context;
// constructors
private IfaceImpl( Sql context ) {
this.context = context;
ref_name = "";
ref_package = "";
R401_is_a_File_inst = FileImpl.EMPTY_FILE;
R418_is_implemented_by_Port_set = new PortSetImpl();
R419_defines_communication_through_Message_set = new MessageSetImpl();
R421_C_I_inst = C_IImpl.EMPTY_C_I;
}
private IfaceImpl( Sql context, UniqueId instanceId, String ref_name, String ref_package ) {
super(instanceId);
this.context = context;
this.ref_name = ref_name;
this.ref_package = ref_package;
R401_is_a_File_inst = FileImpl.EMPTY_FILE;
R418_is_implemented_by_Port_set = new PortSetImpl();
R419_defines_communication_through_Message_set = new MessageSetImpl();
R421_C_I_inst = C_IImpl.EMPTY_C_I;
}
public static Iface create( Sql context ) throws XtumlException {
Iface newIface = new IfaceImpl( context );
if ( context.addInstance( newIface ) ) {
newIface.getRunContext().addChange(new InstanceCreatedDelta(newIface, KEY_LETTERS));
return newIface;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Iface create( Sql context, String ref_name, String ref_package ) throws XtumlException {
return create(context, UniqueId.random(), ref_name, ref_package);
}
public static Iface create( Sql context, UniqueId instanceId, String ref_name, String ref_package ) throws XtumlException {
Iface newIface = new IfaceImpl( context, instanceId, ref_name, ref_package );
if ( context.addInstance( newIface ) ) {
return newIface;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String ref_name;
@Override
public void setName(String ref_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_name, this.ref_name)) {
final String oldValue = this.ref_name;
this.ref_name = ref_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_name", oldValue, this.ref_name));
if ( !R419_defines_communication_through_Message().isEmpty() ) R419_defines_communication_through_Message().setIface_name( ref_name );
if ( !R418_is_implemented_by_Port().isEmpty() ) R418_is_implemented_by_Port().setIface_name( ref_name );
}
}
@Override
public String getName() throws XtumlException {
checkLiving();
return ref_name;
}
private String ref_package;
@Override
public void setPackage(String ref_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_package, this.ref_package)) {
final String oldValue = this.ref_package;
this.ref_package = ref_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_package", oldValue, this.ref_package));
if ( !R418_is_implemented_by_Port().isEmpty() ) R418_is_implemented_by_Port().setIface_package( ref_package );
if ( !R419_defines_communication_through_Message().isEmpty() ) R419_defines_communication_through_Message().setIface_package( ref_package );
}
}
@Override
public String getPackage() throws XtumlException {
checkLiving();
return ref_package;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getName(), getPackage());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public void render() throws XtumlException {
File file = self().R401_is_a_File();
String imports = file.getFormattedImports( ImportType.IMPL );
MessageSet to_provider_msgs = ((MessageSet)self().R419_defines_communication_through_Message().where(selected -> ((Message)selected).getTo_provider()));
Message msg;
for ( Iterator _msg_iter = to_provider_msgs.elements().iterator(); _msg_iter.hasNext(); ) {
msg = _msg_iter.next();
msg.render();
}
String to_provider_message_block = context().T().body();
context().T().clear();
MessageSet from_provider_msgs = ((MessageSet)self().R419_defines_communication_through_Message().where(selected -> !((Message)selected).getTo_provider()));
for ( Iterator _msg_iter = from_provider_msgs.elements().iterator(); _msg_iter.hasNext(); ) {
msg = _msg_iter.next();
msg.render();
}
String from_provider_message_block = context().T().body();
context().T().clear();
context().T().include( "interface/t.interface.java", from_provider_message_block, imports, self(), to_provider_message_block );
context().T().emit( ( ( file.getPath() + "/" ) + self().getName() ) + file.getExtension() );
context().T().clear();
}
// static operations
// events
// selections
private File R401_is_a_File_inst;
@Override
public void setR401_is_a_File( File inst ) {
R401_is_a_File_inst = inst;
}
@Override
public File R401_is_a_File() throws XtumlException {
return R401_is_a_File_inst;
}
private PortSet R418_is_implemented_by_Port_set;
@Override
public void addR418_is_implemented_by_Port( Port inst ) {
R418_is_implemented_by_Port_set.add(inst);
}
@Override
public void removeR418_is_implemented_by_Port( Port inst ) {
R418_is_implemented_by_Port_set.remove(inst);
}
@Override
public PortSet R418_is_implemented_by_Port() throws XtumlException {
return R418_is_implemented_by_Port_set;
}
private MessageSet R419_defines_communication_through_Message_set;
@Override
public void addR419_defines_communication_through_Message( Message inst ) {
R419_defines_communication_through_Message_set.add(inst);
}
@Override
public void removeR419_defines_communication_through_Message( Message inst ) {
R419_defines_communication_through_Message_set.remove(inst);
}
@Override
public MessageSet R419_defines_communication_through_Message() throws XtumlException {
return R419_defines_communication_through_Message_set;
}
private C_I R421_C_I_inst;
@Override
public void setR421_C_I( C_I inst ) {
R421_C_I_inst = inst;
}
@Override
public C_I R421_C_I() throws XtumlException {
return R421_C_I_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Iface self() {
return this;
}
@Override
public Iface oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_IFACE;
}
}
class EmptyIface extends ModelInstance implements Iface {
// attributes
public void setName( String ref_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setPackage( String ref_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getPackage() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
public void render() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public File R401_is_a_File() {
return FileImpl.EMPTY_FILE;
}
@Override
public PortSet R418_is_implemented_by_Port() {
return (new PortSetImpl());
}
@Override
public MessageSet R419_defines_communication_through_Message() {
return (new MessageSetImpl());
}
@Override
public C_I R421_C_I() {
return C_IImpl.EMPTY_C_I;
}
@Override
public String getKeyLetters() {
return IfaceImpl.KEY_LETTERS;
}
@Override
public Iface self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Iface oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return IfaceImpl.EMPTY_IFACE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy