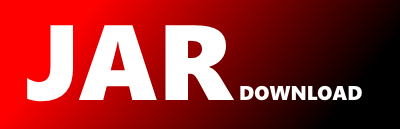
io.ciera.tool.sql.architecture.invocable.impl.InvocableObjectImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.architecture.invocable.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.architecture.classes.AttributeDerivation;
import io.ciera.tool.sql.architecture.classes.Operation;
import io.ciera.tool.sql.architecture.classes.impl.AttributeDerivationImpl;
import io.ciera.tool.sql.architecture.classes.impl.OperationImpl;
import io.ciera.tool.sql.architecture.component.Function;
import io.ciera.tool.sql.architecture.component.UtilityFunction;
import io.ciera.tool.sql.architecture.component.impl.FunctionImpl;
import io.ciera.tool.sql.architecture.component.impl.UtilityFunctionImpl;
import io.ciera.tool.sql.architecture.expression.Invocation;
import io.ciera.tool.sql.architecture.expression.InvocationSet;
import io.ciera.tool.sql.architecture.expression.impl.InvocationSetImpl;
import io.ciera.tool.sql.architecture.interfaces.PortMessage;
import io.ciera.tool.sql.architecture.interfaces.impl.PortMessageImpl;
import io.ciera.tool.sql.architecture.invocable.CodeBlock;
import io.ciera.tool.sql.architecture.invocable.CodeBlockSet;
import io.ciera.tool.sql.architecture.invocable.FormalParameter;
import io.ciera.tool.sql.architecture.invocable.FormalParameterSet;
import io.ciera.tool.sql.architecture.invocable.GenericInvocable;
import io.ciera.tool.sql.architecture.invocable.InvocableObject;
import io.ciera.tool.sql.architecture.invocable.impl.CodeBlockSetImpl;
import io.ciera.tool.sql.architecture.invocable.impl.FormalParameterImpl;
import io.ciera.tool.sql.architecture.invocable.impl.FormalParameterSetImpl;
import io.ciera.tool.sql.architecture.invocable.impl.GenericInvocableImpl;
import io.ciera.tool.sql.architecture.statemachine.Event;
import io.ciera.tool.sql.architecture.statemachine.State;
import io.ciera.tool.sql.architecture.statemachine.StateTransition;
import io.ciera.tool.sql.architecture.statemachine.impl.EventImpl;
import io.ciera.tool.sql.architecture.statemachine.impl.StateImpl;
import io.ciera.tool.sql.architecture.statemachine.impl.StateTransitionImpl;
import io.ciera.tool.sql.architecture.type.TypeReference;
import io.ciera.tool.sql.architecture.type.impl.TypeReferenceImpl;
import io.ciera.tool.sql.ooaofooa.body.Body;
import io.ciera.tool.sql.ooaofooa.body.impl.BodyImpl;
public class InvocableObjectImpl extends ModelInstance implements InvocableObject {
public static final String KEY_LETTERS = "InvocableObject";
public static final InvocableObject EMPTY_INVOCABLEOBJECT = new EmptyInvocableObject();
private Sql context;
// constructors
private InvocableObjectImpl( Sql context ) {
this.context = context;
m_parent_name = "";
m_parent_package = "";
m_name = "";
ref_type_name = "";
ref_type_package = "";
ref_type_reference_name = "";
m_oal = "";
R4000_has_CodeBlock_set = new CodeBlockSetImpl();
R427_is_a_AttributeDerivation_inst = AttributeDerivationImpl.EMPTY_ATTRIBUTEDERIVATION;
R427_is_a_Event_inst = EventImpl.EMPTY_EVENT;
R427_is_a_Function_inst = FunctionImpl.EMPTY_FUNCTION;
R427_is_a_GenericInvocable_inst = GenericInvocableImpl.EMPTY_GENERICINVOCABLE;
R427_is_a_Operation_inst = OperationImpl.EMPTY_OPERATION;
R427_is_a_PortMessage_inst = PortMessageImpl.EMPTY_PORTMESSAGE;
R427_is_a_State_inst = StateImpl.EMPTY_STATE;
R427_is_a_StateTransition_inst = StateTransitionImpl.EMPTY_STATETRANSITION;
R427_is_a_UtilityFunction_inst = UtilityFunctionImpl.EMPTY_UTILITYFUNCTION;
R428_return_value_is_typed_by_TypeReference_inst = TypeReferenceImpl.EMPTY_TYPEREFERENCE;
R429_declares_signature_with_FormalParameter_set = new FormalParameterSetImpl();
R432_Body_inst = BodyImpl.EMPTY_BODY;
R792_invoked_through_Invocation_set = new InvocationSetImpl();
}
private InvocableObjectImpl( Sql context, UniqueId instanceId, String m_parent_name, String m_parent_package, String m_name, String ref_type_name, String ref_type_package, String ref_type_reference_name, String m_oal ) {
super(instanceId);
this.context = context;
this.m_parent_name = m_parent_name;
this.m_parent_package = m_parent_package;
this.m_name = m_name;
this.ref_type_name = ref_type_name;
this.ref_type_package = ref_type_package;
this.ref_type_reference_name = ref_type_reference_name;
this.m_oal = m_oal;
R4000_has_CodeBlock_set = new CodeBlockSetImpl();
R427_is_a_AttributeDerivation_inst = AttributeDerivationImpl.EMPTY_ATTRIBUTEDERIVATION;
R427_is_a_Event_inst = EventImpl.EMPTY_EVENT;
R427_is_a_Function_inst = FunctionImpl.EMPTY_FUNCTION;
R427_is_a_GenericInvocable_inst = GenericInvocableImpl.EMPTY_GENERICINVOCABLE;
R427_is_a_Operation_inst = OperationImpl.EMPTY_OPERATION;
R427_is_a_PortMessage_inst = PortMessageImpl.EMPTY_PORTMESSAGE;
R427_is_a_State_inst = StateImpl.EMPTY_STATE;
R427_is_a_StateTransition_inst = StateTransitionImpl.EMPTY_STATETRANSITION;
R427_is_a_UtilityFunction_inst = UtilityFunctionImpl.EMPTY_UTILITYFUNCTION;
R428_return_value_is_typed_by_TypeReference_inst = TypeReferenceImpl.EMPTY_TYPEREFERENCE;
R429_declares_signature_with_FormalParameter_set = new FormalParameterSetImpl();
R432_Body_inst = BodyImpl.EMPTY_BODY;
R792_invoked_through_Invocation_set = new InvocationSetImpl();
}
public static InvocableObject create( Sql context ) throws XtumlException {
InvocableObject newInvocableObject = new InvocableObjectImpl( context );
if ( context.addInstance( newInvocableObject ) ) {
newInvocableObject.getRunContext().addChange(new InstanceCreatedDelta(newInvocableObject, KEY_LETTERS));
return newInvocableObject;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static InvocableObject create( Sql context, String m_parent_name, String m_parent_package, String m_name, String ref_type_name, String ref_type_package, String ref_type_reference_name, String m_oal ) throws XtumlException {
return create(context, UniqueId.random(), m_parent_name, m_parent_package, m_name, ref_type_name, ref_type_package, ref_type_reference_name, m_oal);
}
public static InvocableObject create( Sql context, UniqueId instanceId, String m_parent_name, String m_parent_package, String m_name, String ref_type_name, String ref_type_package, String ref_type_reference_name, String m_oal ) throws XtumlException {
InvocableObject newInvocableObject = new InvocableObjectImpl( context, instanceId, m_parent_name, m_parent_package, m_name, ref_type_name, ref_type_package, ref_type_reference_name, m_oal );
if ( context.addInstance( newInvocableObject ) ) {
return newInvocableObject;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String m_parent_name;
@Override
public String getParent_name() throws XtumlException {
checkLiving();
return m_parent_name;
}
@Override
public void setParent_name(String m_parent_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_parent_name, this.m_parent_name)) {
final String oldValue = this.m_parent_name;
this.m_parent_name = m_parent_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_parent_name", oldValue, this.m_parent_name));
if ( !R427_is_a_GenericInvocable().isEmpty() ) R427_is_a_GenericInvocable().setParent_name( m_parent_name );
if ( !R4000_has_CodeBlock().isEmpty() ) R4000_has_CodeBlock().setParent_name( m_parent_name );
if ( !R427_is_a_Event().isEmpty() ) R427_is_a_Event().setSm_name( m_parent_name );
if ( !R429_declares_signature_with_FormalParameter().isEmpty() ) R429_declares_signature_with_FormalParameter().setParent_name( m_parent_name );
if ( !R427_is_a_Function().isEmpty() ) R427_is_a_Function().setComp_name( m_parent_name );
if ( !R427_is_a_AttributeDerivation().isEmpty() ) R427_is_a_AttributeDerivation().setClass_name( m_parent_name );
if ( !R792_invoked_through_Invocation().isEmpty() ) R792_invoked_through_Invocation().setInvoked_parent_name( m_parent_name );
if ( !R427_is_a_State().isEmpty() ) R427_is_a_State().setSm_name( m_parent_name );
if ( !R427_is_a_UtilityFunction().isEmpty() ) R427_is_a_UtilityFunction().setUtility_name( m_parent_name );
if ( !R427_is_a_StateTransition().isEmpty() ) R427_is_a_StateTransition().setSm_name( m_parent_name );
if ( !R427_is_a_PortMessage().isEmpty() ) R427_is_a_PortMessage().setPort_name( m_parent_name );
if ( !R427_is_a_Operation().isEmpty() ) R427_is_a_Operation().setClass_name( m_parent_name );
}
}
private String m_parent_package;
@Override
public String getParent_package() throws XtumlException {
checkLiving();
return m_parent_package;
}
@Override
public void setParent_package(String m_parent_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_parent_package, this.m_parent_package)) {
final String oldValue = this.m_parent_package;
this.m_parent_package = m_parent_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_parent_package", oldValue, this.m_parent_package));
if ( !R429_declares_signature_with_FormalParameter().isEmpty() ) R429_declares_signature_with_FormalParameter().setParent_package( m_parent_package );
if ( !R427_is_a_State().isEmpty() ) R427_is_a_State().setSm_package( m_parent_package );
if ( !R427_is_a_Event().isEmpty() ) R427_is_a_Event().setSm_package( m_parent_package );
if ( !R4000_has_CodeBlock().isEmpty() ) R4000_has_CodeBlock().setParent_package( m_parent_package );
if ( !R792_invoked_through_Invocation().isEmpty() ) R792_invoked_through_Invocation().setInvoked_parent_package( m_parent_package );
if ( !R427_is_a_Function().isEmpty() ) R427_is_a_Function().setComp_package( m_parent_package );
if ( !R427_is_a_Operation().isEmpty() ) R427_is_a_Operation().setClass_package( m_parent_package );
if ( !R427_is_a_UtilityFunction().isEmpty() ) R427_is_a_UtilityFunction().setUtility_package( m_parent_package );
if ( !R427_is_a_PortMessage().isEmpty() ) R427_is_a_PortMessage().setPort_package( m_parent_package );
if ( !R427_is_a_StateTransition().isEmpty() ) R427_is_a_StateTransition().setSm_package( m_parent_package );
if ( !R427_is_a_GenericInvocable().isEmpty() ) R427_is_a_GenericInvocable().setParent_package( m_parent_package );
if ( !R427_is_a_AttributeDerivation().isEmpty() ) R427_is_a_AttributeDerivation().setClass_package( m_parent_package );
}
}
private String m_name;
@Override
public String getName() throws XtumlException {
checkLiving();
return m_name;
}
@Override
public void setName(String m_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_name, this.m_name)) {
final String oldValue = this.m_name;
this.m_name = m_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_name", oldValue, this.m_name));
if ( !R427_is_a_Operation().isEmpty() ) R427_is_a_Operation().setName( m_name );
if ( !R4000_has_CodeBlock().isEmpty() ) R4000_has_CodeBlock().setBody_name( m_name );
if ( !R427_is_a_PortMessage().isEmpty() ) R427_is_a_PortMessage().setMsg_name( m_name );
if ( !R427_is_a_GenericInvocable().isEmpty() ) R427_is_a_GenericInvocable().setName( m_name );
if ( !R427_is_a_UtilityFunction().isEmpty() ) R427_is_a_UtilityFunction().setName( m_name );
if ( !R427_is_a_Function().isEmpty() ) R427_is_a_Function().setName( m_name );
if ( !R427_is_a_Event().isEmpty() ) R427_is_a_Event().setName( m_name );
if ( !R427_is_a_AttributeDerivation().isEmpty() ) R427_is_a_AttributeDerivation().setAttribute_name( m_name );
if ( !R792_invoked_through_Invocation().isEmpty() ) R792_invoked_through_Invocation().setInvoked_name( m_name );
if ( !R429_declares_signature_with_FormalParameter().isEmpty() ) R429_declares_signature_with_FormalParameter().setInvocable_name( m_name );
if ( !R427_is_a_State().isEmpty() ) R427_is_a_State().setName( m_name );
if ( !R427_is_a_StateTransition().isEmpty() ) R427_is_a_StateTransition().setName( m_name );
}
}
private String ref_type_name;
@Override
public String getType_name() throws XtumlException {
checkLiving();
return ref_type_name;
}
@Override
public void setType_name(String ref_type_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_type_name, this.ref_type_name)) {
final String oldValue = this.ref_type_name;
this.ref_type_name = ref_type_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_type_name", oldValue, this.ref_type_name));
}
}
private String ref_type_package;
@Override
public void setType_package(String ref_type_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_type_package, this.ref_type_package)) {
final String oldValue = this.ref_type_package;
this.ref_type_package = ref_type_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_type_package", oldValue, this.ref_type_package));
}
}
@Override
public String getType_package() throws XtumlException {
checkLiving();
return ref_type_package;
}
private String ref_type_reference_name;
@Override
public void setType_reference_name(String ref_type_reference_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_type_reference_name, this.ref_type_reference_name)) {
final String oldValue = this.ref_type_reference_name;
this.ref_type_reference_name = ref_type_reference_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_type_reference_name", oldValue, this.ref_type_reference_name));
}
}
@Override
public String getType_reference_name() throws XtumlException {
checkLiving();
return ref_type_reference_name;
}
private String m_oal;
@Override
public String getOal() throws XtumlException {
checkLiving();
return m_oal;
}
@Override
public void setOal(String m_oal) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_oal, this.m_oal)) {
final String oldValue = this.m_oal;
this.m_oal = m_oal;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_oal", oldValue, this.m_oal));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getParent_name(), getParent_package(), getName());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public String body() throws XtumlException {
String body = "{}";
CodeBlock code_block = self().R4000_has_CodeBlock().anyWhere(selected -> ((CodeBlock)selected).getTop_level());
if ( !code_block.isEmpty() ) {
code_block.render();
body = context().T().body();
context().T().clear();
}
return body;
}
@Override
public String get_signature() throws XtumlException {
TypeReference return_type = self().R428_return_value_is_typed_by_TypeReference();
String signature = return_type.getType_reference_name() + "(";
FormalParameter parm = self().R429_declares_signature_with_FormalParameter().any();
FormalParameter prev_parm = parm.R404_follows_FormalParameter();
while ( !prev_parm.isEmpty() ) {
parm = prev_parm;
prev_parm = parm.R404_follows_FormalParameter();
}
String sep = "";
while ( !parm.isEmpty() ) {
TypeReference parm_type = parm.R431_is_typed_by_TypeReference();
signature = ( signature + sep ) + parm_type.getType_reference_name();
sep = ",";
parm = parm.R404_precedes_FormalParameter();
}
signature = signature + ")";
return signature;
}
@Override
public String modifiers() throws XtumlException {
return "";
}
@Override
public String parameter_list() throws XtumlException {
String parameter_list = "";
FormalParameter parm = FormalParameterImpl.EMPTY_FORMALPARAMETER;
FormalParameter prev_parm = self().R429_declares_signature_with_FormalParameter().any();
while ( !prev_parm.isEmpty() ) {
parm = prev_parm;
prev_parm = parm.R404_follows_FormalParameter();
}
String separator = " ";
while ( !parm.isEmpty() ) {
TypeReference parm_type = parm.R431_is_typed_by_TypeReference();
parameter_list = ( ( ( ( parameter_list + separator ) + "final " ) + parm_type.getType_reference_name() ) + " " ) + parm.getName();
separator = ", ";
parm = parm.R404_precedes_FormalParameter();
if ( parm.isEmpty() ) {
parameter_list = parameter_list + " ";
}
}
return parameter_list;
}
// static operations
// events
// selections
private CodeBlockSet R4000_has_CodeBlock_set;
@Override
public void addR4000_has_CodeBlock( CodeBlock inst ) {
R4000_has_CodeBlock_set.add(inst);
}
@Override
public void removeR4000_has_CodeBlock( CodeBlock inst ) {
R4000_has_CodeBlock_set.remove(inst);
}
@Override
public CodeBlockSet R4000_has_CodeBlock() throws XtumlException {
return R4000_has_CodeBlock_set;
}
private AttributeDerivation R427_is_a_AttributeDerivation_inst;
@Override
public void setR427_is_a_AttributeDerivation( AttributeDerivation inst ) {
R427_is_a_AttributeDerivation_inst = inst;
}
@Override
public AttributeDerivation R427_is_a_AttributeDerivation() throws XtumlException {
return R427_is_a_AttributeDerivation_inst;
}
private Event R427_is_a_Event_inst;
@Override
public void setR427_is_a_Event( Event inst ) {
R427_is_a_Event_inst = inst;
}
@Override
public Event R427_is_a_Event() throws XtumlException {
return R427_is_a_Event_inst;
}
private Function R427_is_a_Function_inst;
@Override
public void setR427_is_a_Function( Function inst ) {
R427_is_a_Function_inst = inst;
}
@Override
public Function R427_is_a_Function() throws XtumlException {
return R427_is_a_Function_inst;
}
private GenericInvocable R427_is_a_GenericInvocable_inst;
@Override
public void setR427_is_a_GenericInvocable( GenericInvocable inst ) {
R427_is_a_GenericInvocable_inst = inst;
}
@Override
public GenericInvocable R427_is_a_GenericInvocable() throws XtumlException {
return R427_is_a_GenericInvocable_inst;
}
private Operation R427_is_a_Operation_inst;
@Override
public void setR427_is_a_Operation( Operation inst ) {
R427_is_a_Operation_inst = inst;
}
@Override
public Operation R427_is_a_Operation() throws XtumlException {
return R427_is_a_Operation_inst;
}
private PortMessage R427_is_a_PortMessage_inst;
@Override
public void setR427_is_a_PortMessage( PortMessage inst ) {
R427_is_a_PortMessage_inst = inst;
}
@Override
public PortMessage R427_is_a_PortMessage() throws XtumlException {
return R427_is_a_PortMessage_inst;
}
private State R427_is_a_State_inst;
@Override
public void setR427_is_a_State( State inst ) {
R427_is_a_State_inst = inst;
}
@Override
public State R427_is_a_State() throws XtumlException {
return R427_is_a_State_inst;
}
private StateTransition R427_is_a_StateTransition_inst;
@Override
public void setR427_is_a_StateTransition( StateTransition inst ) {
R427_is_a_StateTransition_inst = inst;
}
@Override
public StateTransition R427_is_a_StateTransition() throws XtumlException {
return R427_is_a_StateTransition_inst;
}
private UtilityFunction R427_is_a_UtilityFunction_inst;
@Override
public void setR427_is_a_UtilityFunction( UtilityFunction inst ) {
R427_is_a_UtilityFunction_inst = inst;
}
@Override
public UtilityFunction R427_is_a_UtilityFunction() throws XtumlException {
return R427_is_a_UtilityFunction_inst;
}
private TypeReference R428_return_value_is_typed_by_TypeReference_inst;
@Override
public void setR428_return_value_is_typed_by_TypeReference( TypeReference inst ) {
R428_return_value_is_typed_by_TypeReference_inst = inst;
}
@Override
public TypeReference R428_return_value_is_typed_by_TypeReference() throws XtumlException {
return R428_return_value_is_typed_by_TypeReference_inst;
}
private FormalParameterSet R429_declares_signature_with_FormalParameter_set;
@Override
public void addR429_declares_signature_with_FormalParameter( FormalParameter inst ) {
R429_declares_signature_with_FormalParameter_set.add(inst);
}
@Override
public void removeR429_declares_signature_with_FormalParameter( FormalParameter inst ) {
R429_declares_signature_with_FormalParameter_set.remove(inst);
}
@Override
public FormalParameterSet R429_declares_signature_with_FormalParameter() throws XtumlException {
return R429_declares_signature_with_FormalParameter_set;
}
private Body R432_Body_inst;
@Override
public void setR432_Body( Body inst ) {
R432_Body_inst = inst;
}
@Override
public Body R432_Body() throws XtumlException {
return R432_Body_inst;
}
private InvocationSet R792_invoked_through_Invocation_set;
@Override
public void addR792_invoked_through_Invocation( Invocation inst ) {
R792_invoked_through_Invocation_set.add(inst);
}
@Override
public void removeR792_invoked_through_Invocation( Invocation inst ) {
R792_invoked_through_Invocation_set.remove(inst);
}
@Override
public InvocationSet R792_invoked_through_Invocation() throws XtumlException {
return R792_invoked_through_Invocation_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public InvocableObject self() {
return this;
}
@Override
public InvocableObject oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_INVOCABLEOBJECT;
}
}
class EmptyInvocableObject extends ModelInstance implements InvocableObject {
// attributes
public String getParent_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setParent_name( String m_parent_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getParent_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setParent_package( String m_parent_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getType_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setType_name( String ref_type_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setType_package( String ref_type_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getType_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setType_reference_name( String ref_type_reference_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getType_reference_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getOal() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setOal( String m_oal ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
public String body() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
public String get_signature() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
public String modifiers() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
public String parameter_list() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public CodeBlockSet R4000_has_CodeBlock() {
return (new CodeBlockSetImpl());
}
@Override
public AttributeDerivation R427_is_a_AttributeDerivation() {
return AttributeDerivationImpl.EMPTY_ATTRIBUTEDERIVATION;
}
@Override
public Event R427_is_a_Event() {
return EventImpl.EMPTY_EVENT;
}
@Override
public Function R427_is_a_Function() {
return FunctionImpl.EMPTY_FUNCTION;
}
@Override
public GenericInvocable R427_is_a_GenericInvocable() {
return GenericInvocableImpl.EMPTY_GENERICINVOCABLE;
}
@Override
public Operation R427_is_a_Operation() {
return OperationImpl.EMPTY_OPERATION;
}
@Override
public PortMessage R427_is_a_PortMessage() {
return PortMessageImpl.EMPTY_PORTMESSAGE;
}
@Override
public State R427_is_a_State() {
return StateImpl.EMPTY_STATE;
}
@Override
public StateTransition R427_is_a_StateTransition() {
return StateTransitionImpl.EMPTY_STATETRANSITION;
}
@Override
public UtilityFunction R427_is_a_UtilityFunction() {
return UtilityFunctionImpl.EMPTY_UTILITYFUNCTION;
}
@Override
public TypeReference R428_return_value_is_typed_by_TypeReference() {
return TypeReferenceImpl.EMPTY_TYPEREFERENCE;
}
@Override
public FormalParameterSet R429_declares_signature_with_FormalParameter() {
return (new FormalParameterSetImpl());
}
@Override
public Body R432_Body() {
return BodyImpl.EMPTY_BODY;
}
@Override
public InvocationSet R792_invoked_through_Invocation() {
return (new InvocationSetImpl());
}
@Override
public String getKeyLetters() {
return InvocableObjectImpl.KEY_LETTERS;
}
@Override
public InvocableObject self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public InvocableObject oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return InvocableObjectImpl.EMPTY_INVOCABLEOBJECT;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy