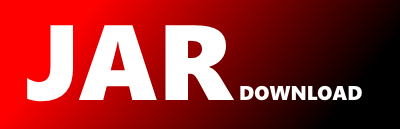
io.ciera.tool.sql.loader.impl.AttributeLoaderImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.loader.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.loader.AttributeLoader;
import io.ciera.tool.sql.loader.BooleanLoader;
import io.ciera.tool.sql.loader.EnumeratorLoader;
import io.ciera.tool.sql.loader.GeneralLoader;
import io.ciera.tool.sql.loader.InstanceLoader;
import io.ciera.tool.sql.loader.IntegerLoader;
import io.ciera.tool.sql.loader.RealLoader;
import io.ciera.tool.sql.loader.StringLoader;
import io.ciera.tool.sql.loader.impl.AttributeLoaderImpl;
import io.ciera.tool.sql.loader.impl.BooleanLoaderImpl;
import io.ciera.tool.sql.loader.impl.EnumeratorLoaderImpl;
import io.ciera.tool.sql.loader.impl.GeneralLoaderImpl;
import io.ciera.tool.sql.loader.impl.InstanceLoaderImpl;
import io.ciera.tool.sql.loader.impl.IntegerLoaderImpl;
import io.ciera.tool.sql.loader.impl.RealLoaderImpl;
import io.ciera.tool.sql.loader.impl.StringLoaderImpl;
public class AttributeLoaderImpl extends ModelInstance implements AttributeLoader {
public static final String KEY_LETTERS = "Z_AttributeLoader";
public static final AttributeLoader EMPTY_ATTRIBUTELOADER = new EmptyAttributeLoader();
private Sql context;
// constructors
private AttributeLoaderImpl( Sql context ) {
this.context = context;
ref_loader_name = "";
ref_loader_package = "";
ref_class_name = "";
m_attr_name = "";
m_index = 0;
ref_prev_attr_name = "";
ref_prev_index = 0;
m_value_index = 0;
R3006_invoked_by_InstanceLoader_inst = InstanceLoaderImpl.EMPTY_INSTANCELOADER;
R3007_follows_AttributeLoader_inst = AttributeLoaderImpl.EMPTY_ATTRIBUTELOADER;
R3007_precedes_AttributeLoader_inst = AttributeLoaderImpl.EMPTY_ATTRIBUTELOADER;
R3008_is_a_BooleanLoader_inst = BooleanLoaderImpl.EMPTY_BOOLEANLOADER;
R3008_is_a_EnumeratorLoader_inst = EnumeratorLoaderImpl.EMPTY_ENUMERATORLOADER;
R3008_is_a_GeneralLoader_inst = GeneralLoaderImpl.EMPTY_GENERALLOADER;
R3008_is_a_IntegerLoader_inst = IntegerLoaderImpl.EMPTY_INTEGERLOADER;
R3008_is_a_RealLoader_inst = RealLoaderImpl.EMPTY_REALLOADER;
R3008_is_a_StringLoader_inst = StringLoaderImpl.EMPTY_STRINGLOADER;
}
private AttributeLoaderImpl( Sql context, UniqueId instanceId, String ref_loader_name, String ref_loader_package, String ref_class_name, String m_attr_name, int m_index, String ref_prev_attr_name, int ref_prev_index, int m_value_index ) {
super(instanceId);
this.context = context;
this.ref_loader_name = ref_loader_name;
this.ref_loader_package = ref_loader_package;
this.ref_class_name = ref_class_name;
this.m_attr_name = m_attr_name;
this.m_index = m_index;
this.ref_prev_attr_name = ref_prev_attr_name;
this.ref_prev_index = ref_prev_index;
this.m_value_index = m_value_index;
R3006_invoked_by_InstanceLoader_inst = InstanceLoaderImpl.EMPTY_INSTANCELOADER;
R3007_follows_AttributeLoader_inst = AttributeLoaderImpl.EMPTY_ATTRIBUTELOADER;
R3007_precedes_AttributeLoader_inst = AttributeLoaderImpl.EMPTY_ATTRIBUTELOADER;
R3008_is_a_BooleanLoader_inst = BooleanLoaderImpl.EMPTY_BOOLEANLOADER;
R3008_is_a_EnumeratorLoader_inst = EnumeratorLoaderImpl.EMPTY_ENUMERATORLOADER;
R3008_is_a_GeneralLoader_inst = GeneralLoaderImpl.EMPTY_GENERALLOADER;
R3008_is_a_IntegerLoader_inst = IntegerLoaderImpl.EMPTY_INTEGERLOADER;
R3008_is_a_RealLoader_inst = RealLoaderImpl.EMPTY_REALLOADER;
R3008_is_a_StringLoader_inst = StringLoaderImpl.EMPTY_STRINGLOADER;
}
public static AttributeLoader create( Sql context ) throws XtumlException {
AttributeLoader newAttributeLoader = new AttributeLoaderImpl( context );
if ( context.addInstance( newAttributeLoader ) ) {
newAttributeLoader.getRunContext().addChange(new InstanceCreatedDelta(newAttributeLoader, KEY_LETTERS));
return newAttributeLoader;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static AttributeLoader create( Sql context, String ref_loader_name, String ref_loader_package, String ref_class_name, String m_attr_name, int m_index, String ref_prev_attr_name, int ref_prev_index, int m_value_index ) throws XtumlException {
return create(context, UniqueId.random(), ref_loader_name, ref_loader_package, ref_class_name, m_attr_name, m_index, ref_prev_attr_name, ref_prev_index, m_value_index);
}
public static AttributeLoader create( Sql context, UniqueId instanceId, String ref_loader_name, String ref_loader_package, String ref_class_name, String m_attr_name, int m_index, String ref_prev_attr_name, int ref_prev_index, int m_value_index ) throws XtumlException {
AttributeLoader newAttributeLoader = new AttributeLoaderImpl( context, instanceId, ref_loader_name, ref_loader_package, ref_class_name, m_attr_name, m_index, ref_prev_attr_name, ref_prev_index, m_value_index );
if ( context.addInstance( newAttributeLoader ) ) {
return newAttributeLoader;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String ref_loader_name;
@Override
public void setLoader_name(String ref_loader_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_loader_name, this.ref_loader_name)) {
final String oldValue = this.ref_loader_name;
this.ref_loader_name = ref_loader_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_loader_name", oldValue, this.ref_loader_name));
if ( !R3008_is_a_GeneralLoader().isEmpty() ) R3008_is_a_GeneralLoader().setLoader_name( ref_loader_name );
if ( !R3008_is_a_IntegerLoader().isEmpty() ) R3008_is_a_IntegerLoader().setLoader_name( ref_loader_name );
if ( !R3008_is_a_RealLoader().isEmpty() ) R3008_is_a_RealLoader().setLoader_name( ref_loader_name );
if ( !R3007_precedes_AttributeLoader().isEmpty() ) R3007_precedes_AttributeLoader().setLoader_name( ref_loader_name );
if ( !R3008_is_a_BooleanLoader().isEmpty() ) R3008_is_a_BooleanLoader().setLoader_name( ref_loader_name );
if ( !R3008_is_a_EnumeratorLoader().isEmpty() ) R3008_is_a_EnumeratorLoader().setLoader_name( ref_loader_name );
if ( !R3008_is_a_StringLoader().isEmpty() ) R3008_is_a_StringLoader().setLoader_name( ref_loader_name );
}
}
@Override
public String getLoader_name() throws XtumlException {
checkLiving();
return ref_loader_name;
}
private String ref_loader_package;
@Override
public void setLoader_package(String ref_loader_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_loader_package, this.ref_loader_package)) {
final String oldValue = this.ref_loader_package;
this.ref_loader_package = ref_loader_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_loader_package", oldValue, this.ref_loader_package));
if ( !R3008_is_a_IntegerLoader().isEmpty() ) R3008_is_a_IntegerLoader().setLoader_package( ref_loader_package );
if ( !R3008_is_a_RealLoader().isEmpty() ) R3008_is_a_RealLoader().setLoader_package( ref_loader_package );
if ( !R3008_is_a_EnumeratorLoader().isEmpty() ) R3008_is_a_EnumeratorLoader().setLoader_package( ref_loader_package );
if ( !R3008_is_a_GeneralLoader().isEmpty() ) R3008_is_a_GeneralLoader().setLoader_package( ref_loader_package );
if ( !R3008_is_a_BooleanLoader().isEmpty() ) R3008_is_a_BooleanLoader().setLoader_package( ref_loader_package );
if ( !R3008_is_a_StringLoader().isEmpty() ) R3008_is_a_StringLoader().setLoader_package( ref_loader_package );
if ( !R3007_precedes_AttributeLoader().isEmpty() ) R3007_precedes_AttributeLoader().setLoader_package( ref_loader_package );
}
}
@Override
public String getLoader_package() throws XtumlException {
checkLiving();
return ref_loader_package;
}
private String ref_class_name;
@Override
public void setClass_name(String ref_class_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_class_name, this.ref_class_name)) {
final String oldValue = this.ref_class_name;
this.ref_class_name = ref_class_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_class_name", oldValue, this.ref_class_name));
if ( !R3008_is_a_EnumeratorLoader().isEmpty() ) R3008_is_a_EnumeratorLoader().setClass_name( ref_class_name );
if ( !R3008_is_a_IntegerLoader().isEmpty() ) R3008_is_a_IntegerLoader().setClass_name( ref_class_name );
if ( !R3008_is_a_RealLoader().isEmpty() ) R3008_is_a_RealLoader().setClass_name( ref_class_name );
if ( !R3008_is_a_BooleanLoader().isEmpty() ) R3008_is_a_BooleanLoader().setClass_name( ref_class_name );
if ( !R3007_precedes_AttributeLoader().isEmpty() ) R3007_precedes_AttributeLoader().setClass_name( ref_class_name );
if ( !R3008_is_a_StringLoader().isEmpty() ) R3008_is_a_StringLoader().setClass_name( ref_class_name );
if ( !R3008_is_a_GeneralLoader().isEmpty() ) R3008_is_a_GeneralLoader().setClass_name( ref_class_name );
}
}
@Override
public String getClass_name() throws XtumlException {
checkLiving();
return ref_class_name;
}
private String m_attr_name;
@Override
public void setAttr_name(String m_attr_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_attr_name, this.m_attr_name)) {
final String oldValue = this.m_attr_name;
this.m_attr_name = m_attr_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_attr_name", oldValue, this.m_attr_name));
if ( !R3008_is_a_GeneralLoader().isEmpty() ) R3008_is_a_GeneralLoader().setAttr_name( m_attr_name );
if ( !R3008_is_a_EnumeratorLoader().isEmpty() ) R3008_is_a_EnumeratorLoader().setAttr_name( m_attr_name );
if ( !R3008_is_a_RealLoader().isEmpty() ) R3008_is_a_RealLoader().setAttr_name( m_attr_name );
if ( !R3008_is_a_IntegerLoader().isEmpty() ) R3008_is_a_IntegerLoader().setAttr_name( m_attr_name );
if ( !R3007_precedes_AttributeLoader().isEmpty() ) R3007_precedes_AttributeLoader().setPrev_attr_name( m_attr_name );
if ( !R3008_is_a_BooleanLoader().isEmpty() ) R3008_is_a_BooleanLoader().setAttr_name( m_attr_name );
if ( !R3008_is_a_StringLoader().isEmpty() ) R3008_is_a_StringLoader().setAttr_name( m_attr_name );
}
}
@Override
public String getAttr_name() throws XtumlException {
checkLiving();
return m_attr_name;
}
private int m_index;
@Override
public int getIndex() throws XtumlException {
checkLiving();
return m_index;
}
@Override
public void setIndex(int m_index) throws XtumlException {
checkLiving();
if (m_index != this.m_index) {
final int oldValue = this.m_index;
this.m_index = m_index;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_index", oldValue, this.m_index));
if ( !R3008_is_a_IntegerLoader().isEmpty() ) R3008_is_a_IntegerLoader().setIndex( m_index );
if ( !R3008_is_a_EnumeratorLoader().isEmpty() ) R3008_is_a_EnumeratorLoader().setIndex( m_index );
if ( !R3008_is_a_StringLoader().isEmpty() ) R3008_is_a_StringLoader().setIndex( m_index );
if ( !R3008_is_a_GeneralLoader().isEmpty() ) R3008_is_a_GeneralLoader().setIndex( m_index );
if ( !R3007_precedes_AttributeLoader().isEmpty() ) R3007_precedes_AttributeLoader().setPrev_index( m_index );
if ( !R3008_is_a_BooleanLoader().isEmpty() ) R3008_is_a_BooleanLoader().setIndex( m_index );
if ( !R3008_is_a_RealLoader().isEmpty() ) R3008_is_a_RealLoader().setIndex( m_index );
}
}
private String ref_prev_attr_name;
@Override
public String getPrev_attr_name() throws XtumlException {
checkLiving();
return ref_prev_attr_name;
}
@Override
public void setPrev_attr_name(String ref_prev_attr_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_prev_attr_name, this.ref_prev_attr_name)) {
final String oldValue = this.ref_prev_attr_name;
this.ref_prev_attr_name = ref_prev_attr_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_prev_attr_name", oldValue, this.ref_prev_attr_name));
}
}
private int ref_prev_index;
@Override
public void setPrev_index(int ref_prev_index) throws XtumlException {
checkLiving();
if (ref_prev_index != this.ref_prev_index) {
final int oldValue = this.ref_prev_index;
this.ref_prev_index = ref_prev_index;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_prev_index", oldValue, this.ref_prev_index));
}
}
@Override
public int getPrev_index() throws XtumlException {
checkLiving();
return ref_prev_index;
}
private int m_value_index;
@Override
public void setValue_index(int m_value_index) throws XtumlException {
checkLiving();
if (m_value_index != this.m_value_index) {
final int oldValue = this.m_value_index;
this.m_value_index = m_value_index;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_value_index", oldValue, this.m_value_index));
}
}
@Override
public int getValue_index() throws XtumlException {
checkLiving();
return m_value_index;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getLoader_name(), getLoader_package(), getClass_name(), getAttr_name(), getIndex());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public void render() throws XtumlException {
BooleanLoader booleanloader = self().R3008_is_a_BooleanLoader();
if ( !booleanloader.isEmpty() ) {
booleanloader.render();
}
else {
EnumeratorLoader enumeratorloader = self().R3008_is_a_EnumeratorLoader();
if ( !enumeratorloader.isEmpty() ) {
enumeratorloader.render();
}
else {
GeneralLoader generalloader = self().R3008_is_a_GeneralLoader();
if ( !generalloader.isEmpty() ) {
generalloader.render();
}
else {
IntegerLoader integerloader = self().R3008_is_a_IntegerLoader();
if ( !integerloader.isEmpty() ) {
integerloader.render();
}
else {
RealLoader realloader = self().R3008_is_a_RealLoader();
if ( !realloader.isEmpty() ) {
realloader.render();
}
else {
StringLoader stringloader = self().R3008_is_a_StringLoader();
if ( !stringloader.isEmpty() ) {
stringloader.render();
}
else {
throw new XtumlException("No subtype selected");
}
}
}
}
}
}
}
// static operations
// events
// selections
private InstanceLoader R3006_invoked_by_InstanceLoader_inst;
@Override
public void setR3006_invoked_by_InstanceLoader( InstanceLoader inst ) {
R3006_invoked_by_InstanceLoader_inst = inst;
}
@Override
public InstanceLoader R3006_invoked_by_InstanceLoader() throws XtumlException {
return R3006_invoked_by_InstanceLoader_inst;
}
private AttributeLoader R3007_follows_AttributeLoader_inst;
@Override
public void setR3007_follows_AttributeLoader( AttributeLoader inst ) {
R3007_follows_AttributeLoader_inst = inst;
}
@Override
public AttributeLoader R3007_follows_AttributeLoader() throws XtumlException {
return R3007_follows_AttributeLoader_inst;
}
private AttributeLoader R3007_precedes_AttributeLoader_inst;
@Override
public void setR3007_precedes_AttributeLoader( AttributeLoader inst ) {
R3007_precedes_AttributeLoader_inst = inst;
}
@Override
public AttributeLoader R3007_precedes_AttributeLoader() throws XtumlException {
return R3007_precedes_AttributeLoader_inst;
}
private BooleanLoader R3008_is_a_BooleanLoader_inst;
@Override
public void setR3008_is_a_BooleanLoader( BooleanLoader inst ) {
R3008_is_a_BooleanLoader_inst = inst;
}
@Override
public BooleanLoader R3008_is_a_BooleanLoader() throws XtumlException {
return R3008_is_a_BooleanLoader_inst;
}
private EnumeratorLoader R3008_is_a_EnumeratorLoader_inst;
@Override
public void setR3008_is_a_EnumeratorLoader( EnumeratorLoader inst ) {
R3008_is_a_EnumeratorLoader_inst = inst;
}
@Override
public EnumeratorLoader R3008_is_a_EnumeratorLoader() throws XtumlException {
return R3008_is_a_EnumeratorLoader_inst;
}
private GeneralLoader R3008_is_a_GeneralLoader_inst;
@Override
public void setR3008_is_a_GeneralLoader( GeneralLoader inst ) {
R3008_is_a_GeneralLoader_inst = inst;
}
@Override
public GeneralLoader R3008_is_a_GeneralLoader() throws XtumlException {
return R3008_is_a_GeneralLoader_inst;
}
private IntegerLoader R3008_is_a_IntegerLoader_inst;
@Override
public void setR3008_is_a_IntegerLoader( IntegerLoader inst ) {
R3008_is_a_IntegerLoader_inst = inst;
}
@Override
public IntegerLoader R3008_is_a_IntegerLoader() throws XtumlException {
return R3008_is_a_IntegerLoader_inst;
}
private RealLoader R3008_is_a_RealLoader_inst;
@Override
public void setR3008_is_a_RealLoader( RealLoader inst ) {
R3008_is_a_RealLoader_inst = inst;
}
@Override
public RealLoader R3008_is_a_RealLoader() throws XtumlException {
return R3008_is_a_RealLoader_inst;
}
private StringLoader R3008_is_a_StringLoader_inst;
@Override
public void setR3008_is_a_StringLoader( StringLoader inst ) {
R3008_is_a_StringLoader_inst = inst;
}
@Override
public StringLoader R3008_is_a_StringLoader() throws XtumlException {
return R3008_is_a_StringLoader_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public AttributeLoader self() {
return this;
}
@Override
public AttributeLoader oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_ATTRIBUTELOADER;
}
}
class EmptyAttributeLoader extends ModelInstance implements AttributeLoader {
// attributes
public void setLoader_name( String ref_loader_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getLoader_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLoader_package( String ref_loader_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getLoader_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setClass_name( String ref_class_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getClass_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAttr_name( String m_attr_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getAttr_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getIndex() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setIndex( int m_index ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getPrev_attr_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setPrev_attr_name( String ref_prev_attr_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setPrev_index( int ref_prev_index ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getPrev_index() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setValue_index( int m_value_index ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getValue_index() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
public void render() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public InstanceLoader R3006_invoked_by_InstanceLoader() {
return InstanceLoaderImpl.EMPTY_INSTANCELOADER;
}
@Override
public AttributeLoader R3007_follows_AttributeLoader() {
return AttributeLoaderImpl.EMPTY_ATTRIBUTELOADER;
}
@Override
public AttributeLoader R3007_precedes_AttributeLoader() {
return AttributeLoaderImpl.EMPTY_ATTRIBUTELOADER;
}
@Override
public BooleanLoader R3008_is_a_BooleanLoader() {
return BooleanLoaderImpl.EMPTY_BOOLEANLOADER;
}
@Override
public EnumeratorLoader R3008_is_a_EnumeratorLoader() {
return EnumeratorLoaderImpl.EMPTY_ENUMERATORLOADER;
}
@Override
public GeneralLoader R3008_is_a_GeneralLoader() {
return GeneralLoaderImpl.EMPTY_GENERALLOADER;
}
@Override
public IntegerLoader R3008_is_a_IntegerLoader() {
return IntegerLoaderImpl.EMPTY_INTEGERLOADER;
}
@Override
public RealLoader R3008_is_a_RealLoader() {
return RealLoaderImpl.EMPTY_REALLOADER;
}
@Override
public StringLoader R3008_is_a_StringLoader() {
return StringLoaderImpl.EMPTY_STRINGLOADER;
}
@Override
public String getKeyLetters() {
return AttributeLoaderImpl.KEY_LETTERS;
}
@Override
public AttributeLoader self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public AttributeLoader oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return AttributeLoaderImpl.EMPTY_ATTRIBUTELOADER;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy