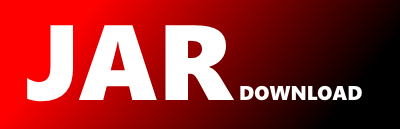
io.ciera.tool.sql.loader.impl.ClassInstanceLoaderImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.loader.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.loader.AttributeLoader;
import io.ciera.tool.sql.loader.ClassInstanceLoader;
import io.ciera.tool.sql.loader.InstanceLoader;
import io.ciera.tool.sql.loader.impl.InstanceLoaderImpl;
import io.ciera.tool.sql.ooaofmarking.Mark;
public class ClassInstanceLoaderImpl extends ModelInstance implements ClassInstanceLoader {
public static final String KEY_LETTERS = "ClassInstanceLoader";
public static final ClassInstanceLoader EMPTY_CLASSINSTANCELOADER = new EmptyClassInstanceLoader();
private Sql context;
// constructors
private ClassInstanceLoaderImpl( Sql context ) {
this.context = context;
ref_loader_name = "";
ref_loader_package = "";
ref_class_name = "";
m_class_package = "";
m_class_key_letters = "";
R3016_is_a_InstanceLoader_inst = InstanceLoaderImpl.EMPTY_INSTANCELOADER;
}
private ClassInstanceLoaderImpl( Sql context, UniqueId instanceId, String ref_loader_name, String ref_loader_package, String ref_class_name, String m_class_package, String m_class_key_letters ) {
super(instanceId);
this.context = context;
this.ref_loader_name = ref_loader_name;
this.ref_loader_package = ref_loader_package;
this.ref_class_name = ref_class_name;
this.m_class_package = m_class_package;
this.m_class_key_letters = m_class_key_letters;
R3016_is_a_InstanceLoader_inst = InstanceLoaderImpl.EMPTY_INSTANCELOADER;
}
public static ClassInstanceLoader create( Sql context ) throws XtumlException {
ClassInstanceLoader newClassInstanceLoader = new ClassInstanceLoaderImpl( context );
if ( context.addInstance( newClassInstanceLoader ) ) {
newClassInstanceLoader.getRunContext().addChange(new InstanceCreatedDelta(newClassInstanceLoader, KEY_LETTERS));
return newClassInstanceLoader;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static ClassInstanceLoader create( Sql context, String ref_loader_name, String ref_loader_package, String ref_class_name, String m_class_package, String m_class_key_letters ) throws XtumlException {
return create(context, UniqueId.random(), ref_loader_name, ref_loader_package, ref_class_name, m_class_package, m_class_key_letters);
}
public static ClassInstanceLoader create( Sql context, UniqueId instanceId, String ref_loader_name, String ref_loader_package, String ref_class_name, String m_class_package, String m_class_key_letters ) throws XtumlException {
ClassInstanceLoader newClassInstanceLoader = new ClassInstanceLoaderImpl( context, instanceId, ref_loader_name, ref_loader_package, ref_class_name, m_class_package, m_class_key_letters );
if ( context.addInstance( newClassInstanceLoader ) ) {
return newClassInstanceLoader;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String ref_loader_name;
@Override
public String getLoader_name() throws XtumlException {
checkLiving();
return ref_loader_name;
}
@Override
public void setLoader_name(String ref_loader_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_loader_name, this.ref_loader_name)) {
final String oldValue = this.ref_loader_name;
this.ref_loader_name = ref_loader_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_loader_name", oldValue, this.ref_loader_name));
}
}
private String ref_loader_package;
@Override
public String getLoader_package() throws XtumlException {
checkLiving();
return ref_loader_package;
}
@Override
public void setLoader_package(String ref_loader_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_loader_package, this.ref_loader_package)) {
final String oldValue = this.ref_loader_package;
this.ref_loader_package = ref_loader_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_loader_package", oldValue, this.ref_loader_package));
}
}
private String ref_class_name;
@Override
public String getClass_name() throws XtumlException {
checkLiving();
return ref_class_name;
}
@Override
public void setClass_name(String ref_class_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_class_name, this.ref_class_name)) {
final String oldValue = this.ref_class_name;
this.ref_class_name = ref_class_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_class_name", oldValue, this.ref_class_name));
}
}
private String m_class_package;
@Override
public String getClass_package() throws XtumlException {
checkLiving();
return m_class_package;
}
@Override
public void setClass_package(String m_class_package) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_class_package, this.m_class_package)) {
final String oldValue = this.m_class_package;
this.m_class_package = m_class_package;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_class_package", oldValue, this.m_class_package));
}
}
private String m_class_key_letters;
@Override
public String getClass_key_letters() throws XtumlException {
checkLiving();
return m_class_key_letters;
}
@Override
public void setClass_key_letters(String m_class_key_letters) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_class_key_letters, this.m_class_key_letters)) {
final String oldValue = this.m_class_key_letters;
this.m_class_key_letters = m_class_key_letters;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_class_key_letters", oldValue, this.m_class_key_letters));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getLoader_name(), getLoader_package(), getClass_name());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public void render() throws XtumlException {
context().T().push_buffer();
AttributeLoader attr_loader = self().R3016_is_a_InstanceLoader().R3006_loads_data_value_using_AttributeLoader().any();
AttributeLoader prev_attr_loader = attr_loader.R3007_follows_AttributeLoader();
while ( !prev_attr_loader.isEmpty() ) {
attr_loader = prev_attr_loader;
prev_attr_loader = attr_loader.R3007_follows_AttributeLoader();
}
String sep = "";
while ( !attr_loader.isEmpty() ) {
context().T().append( sep );
attr_loader.render();
sep = ", ";
attr_loader = attr_loader.R3007_precedes_AttributeLoader();
}
String attribute_loaders = context().T().body();
context().T().clear();
Mark non_persistent_instance_ids_mark = context().Mark_instances().anyWhere(selected -> ( StringUtil.equality(((Mark)selected).getMarkable_name(), "*") && StringUtil.equality(((Mark)selected).getPath(), "*") ) && StringUtil.equality(((Mark)selected).getFeature_name(), "NonPersistentInstanceIds"));
if ( !non_persistent_instance_ids_mark.isEmpty() ) {
attribute_loaders = "UniqueId.random(), " + attribute_loaders;
}
context().T().pop_buffer();
context().T().include( "loader/t.classinstanceloader.java", attribute_loaders, self() );
}
// static operations
// events
// selections
private InstanceLoader R3016_is_a_InstanceLoader_inst;
@Override
public void setR3016_is_a_InstanceLoader( InstanceLoader inst ) {
R3016_is_a_InstanceLoader_inst = inst;
}
@Override
public InstanceLoader R3016_is_a_InstanceLoader() throws XtumlException {
return R3016_is_a_InstanceLoader_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public ClassInstanceLoader self() {
return this;
}
@Override
public ClassInstanceLoader oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_CLASSINSTANCELOADER;
}
}
class EmptyClassInstanceLoader extends ModelInstance implements ClassInstanceLoader {
// attributes
public String getLoader_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLoader_name( String ref_loader_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getLoader_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLoader_package( String ref_loader_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getClass_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setClass_name( String ref_class_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getClass_package() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setClass_package( String m_class_package ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getClass_key_letters() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setClass_key_letters( String m_class_key_letters ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
public void render() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public InstanceLoader R3016_is_a_InstanceLoader() {
return InstanceLoaderImpl.EMPTY_INSTANCELOADER;
}
@Override
public String getKeyLetters() {
return ClassInstanceLoaderImpl.KEY_LETTERS;
}
@Override
public ClassInstanceLoader self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public ClassInstanceLoader oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return ClassInstanceLoaderImpl.EMPTY_CLASSINSTANCELOADER;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy