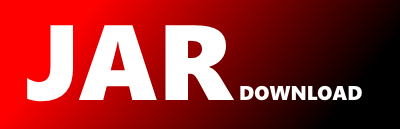
io.ciera.tool.sql.ooaofmarking.impl.MarkImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofmarking.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.ActionHome;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofmarking.Feature;
import io.ciera.tool.sql.ooaofmarking.Mark;
import io.ciera.tool.sql.ooaofmarking.MarkSet;
import io.ciera.tool.sql.ooaofmarking.MarkableElementType;
import io.ciera.tool.sql.ooaofmarking.impl.FeatureImpl;
import io.ciera.tool.sql.ooaofmarking.impl.MarkImpl;
import io.ciera.tool.sql.ooaofmarking.impl.MarkableElementTypeImpl;
import java.util.Iterator;
public class MarkImpl extends ModelInstance implements Mark {
public static final String KEY_LETTERS = "Mark";
public static final Mark EMPTY_MARK = new EmptyMark();
private Sql context;
// constructors
private MarkImpl( Sql context ) {
this.context = context;
ref_markable_name = "";
ref_feature_name = "";
m_path = "";
m_value = "";
R2821_is_marked_by_Feature_inst = FeatureImpl.EMPTY_FEATURE;
R2821_marks_MarkableElementType_inst = MarkableElementTypeImpl.EMPTY_MARKABLEELEMENTTYPE;
R2823_precedes_Mark_inst = MarkImpl.EMPTY_MARK;
R2823_succeeds_Mark_inst = MarkImpl.EMPTY_MARK;
}
private MarkImpl( Sql context, UniqueId instanceId, String ref_markable_name, String ref_feature_name, String m_path, String m_value ) {
super(instanceId);
this.context = context;
this.ref_markable_name = ref_markable_name;
this.ref_feature_name = ref_feature_name;
this.m_path = m_path;
this.m_value = m_value;
R2821_is_marked_by_Feature_inst = FeatureImpl.EMPTY_FEATURE;
R2821_marks_MarkableElementType_inst = MarkableElementTypeImpl.EMPTY_MARKABLEELEMENTTYPE;
R2823_precedes_Mark_inst = MarkImpl.EMPTY_MARK;
R2823_succeeds_Mark_inst = MarkImpl.EMPTY_MARK;
}
public static Mark create( Sql context ) throws XtumlException {
Mark newMark = new MarkImpl( context );
if ( context.addInstance( newMark ) ) {
newMark.getRunContext().addChange(new InstanceCreatedDelta(newMark, KEY_LETTERS));
return newMark;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Mark create( Sql context, String ref_markable_name, String ref_feature_name, String m_path, String m_value ) throws XtumlException {
return create(context, UniqueId.random(), ref_markable_name, ref_feature_name, m_path, m_value);
}
public static Mark create( Sql context, UniqueId instanceId, String ref_markable_name, String ref_feature_name, String m_path, String m_value ) throws XtumlException {
Mark newMark = new MarkImpl( context, instanceId, ref_markable_name, ref_feature_name, m_path, m_value );
if ( context.addInstance( newMark ) ) {
return newMark;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private String ref_markable_name;
@Override
public String getMarkable_name() throws XtumlException {
checkLiving();
return ref_markable_name;
}
@Override
public void setMarkable_name(String ref_markable_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_markable_name, this.ref_markable_name)) {
final String oldValue = this.ref_markable_name;
this.ref_markable_name = ref_markable_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_markable_name", oldValue, this.ref_markable_name));
}
}
private String ref_feature_name;
@Override
public void setFeature_name(String ref_feature_name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(ref_feature_name, this.ref_feature_name)) {
final String oldValue = this.ref_feature_name;
this.ref_feature_name = ref_feature_name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_feature_name", oldValue, this.ref_feature_name));
}
}
@Override
public String getFeature_name() throws XtumlException {
checkLiving();
return ref_feature_name;
}
private String m_path;
@Override
public String getPath() throws XtumlException {
checkLiving();
return m_path;
}
@Override
public void setPath(String m_path) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_path, this.m_path)) {
final String oldValue = this.m_path;
this.m_path = m_path;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_path", oldValue, this.m_path));
}
}
private String m_value;
@Override
public void setValue(String m_value) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_value, this.m_value)) {
final String oldValue = this.m_value;
this.m_value = m_value;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_value", oldValue, this.m_value));
}
}
@Override
public String getValue() throws XtumlException {
checkLiving();
return m_value;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getMarkable_name(), getFeature_name(), getPath());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
@Override
public void dispose() throws XtumlException {
MarkableElementType markable = self().R2821_marks_MarkableElementType();
Feature feature = self().R2821_is_marked_by_Feature();
context().unrelate_R2821_Mark_marks_MarkableElementType( self(), markable );
context().unrelate_R2821_Mark_is_marked_by_Feature( self(), feature );
Mark previous_mark = self().R2823_succeeds_Mark();
if ( !previous_mark.isEmpty() ) {
context().unrelate_R2823_Mark_precedes_Mark( self(), previous_mark );
}
Mark following_mark = self().R2823_precedes_Mark();
if ( !following_mark.isEmpty() ) {
context().unrelate_R2823_Mark_precedes_Mark( following_mark, self() );
}
self().delete();
}
// static operations
public static class CLASS extends ActionHome {
public CLASS( Sql context ) {
super( context );
}
public void load( final String p_filename, final String[] p_values ) throws XtumlException {
boolean done = false;
while ( !done ) {
int count = context().CSV().readline( p_filename, p_values );
if ( count > 0 ) {
if ( count > 1 ) {
String s = p_values[3];
if ( count > 4 ) {
s = ( s + "," ) + p_values[4];
if ( count > 5 ) {
s = ( s + "," ) + p_values[5];
if ( count > 6 ) {
s = ( s + "," ) + p_values[6];
if ( count > 7 ) {
s = ( s + "," ) + p_values[7];
}
}
}
}
new MarkImpl.CLASS(context()).populate( p_values[2], p_values[1], p_values[0], s );
}
}
else {
done = true;
}
}
}
public void persist( final String p_filename, final String[] p_values ) throws XtumlException {
String newfilename = p_filename;
MarkSet marks = context().Mark_instances();
Mark mark;
for ( Iterator _mark_iter = marks.elements().iterator(); _mark_iter.hasNext(); ) {
mark = _mark_iter.next();
p_values[0] = mark.getPath();
p_values[1] = mark.getFeature_name();
p_values[2] = mark.getMarkable_name();
p_values[3] = mark.getValue();
p_values[4] = "";
context().CSV().writeline( newfilename, p_values );
}
}
public Mark populate( final String p_markable_name, final String p_feature_name, final String p_path, final String p_value ) throws XtumlException {
Mark mark = MarkImpl.create( context() );
mark.setPath(p_path);
mark.setValue(p_value);
Feature feature = context().Feature_instances().anyWhere(selected -> StringUtil.equality(((Feature)selected).getName(), p_feature_name));
MarkableElementType markable = feature.R2822_is_available_for_MarkableElementType().anyWhere(selected -> StringUtil.equality(((MarkableElementType)selected).getName(), p_markable_name));
if ( markable.isEmpty() ) {
markable = feature.R2822_is_available_for_MarkableElementType().any();
}
if ( !feature.isEmpty() && !markable.isEmpty() ) {
context().relate_R2821_Mark_is_marked_by_Feature( mark, feature );
context().relate_R2821_Mark_marks_MarkableElementType( mark, markable );
}
return mark;
}
}
// events
// selections
private Feature R2821_is_marked_by_Feature_inst;
@Override
public void setR2821_is_marked_by_Feature( Feature inst ) {
R2821_is_marked_by_Feature_inst = inst;
}
@Override
public Feature R2821_is_marked_by_Feature() throws XtumlException {
return R2821_is_marked_by_Feature_inst;
}
private MarkableElementType R2821_marks_MarkableElementType_inst;
@Override
public void setR2821_marks_MarkableElementType( MarkableElementType inst ) {
R2821_marks_MarkableElementType_inst = inst;
}
@Override
public MarkableElementType R2821_marks_MarkableElementType() throws XtumlException {
return R2821_marks_MarkableElementType_inst;
}
private Mark R2823_precedes_Mark_inst;
@Override
public void setR2823_precedes_Mark( Mark inst ) {
R2823_precedes_Mark_inst = inst;
}
@Override
public Mark R2823_precedes_Mark() throws XtumlException {
return R2823_precedes_Mark_inst;
}
private Mark R2823_succeeds_Mark_inst;
@Override
public void setR2823_succeeds_Mark( Mark inst ) {
R2823_succeeds_Mark_inst = inst;
}
@Override
public Mark R2823_succeeds_Mark() throws XtumlException {
return R2823_succeeds_Mark_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Mark self() {
return this;
}
@Override
public Mark oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_MARK;
}
}
class EmptyMark extends ModelInstance implements Mark {
// attributes
public String getMarkable_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setMarkable_name( String ref_markable_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setFeature_name( String ref_feature_name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getFeature_name() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getPath() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setPath( String m_path ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setValue( String m_value ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getValue() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
public void dispose() throws XtumlException {
throw new EmptyInstanceException( "Cannot invoke operation on empty instance." );
}
// selections
@Override
public Feature R2821_is_marked_by_Feature() {
return FeatureImpl.EMPTY_FEATURE;
}
@Override
public MarkableElementType R2821_marks_MarkableElementType() {
return MarkableElementTypeImpl.EMPTY_MARKABLEELEMENTTYPE;
}
@Override
public Mark R2823_precedes_Mark() {
return MarkImpl.EMPTY_MARK;
}
@Override
public Mark R2823_succeeds_Mark() {
return MarkImpl.EMPTY_MARK;
}
@Override
public String getKeyLetters() {
return MarkImpl.KEY_LETTERS;
}
@Override
public Mark self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Mark oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return MarkImpl.EMPTY_MARK;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy