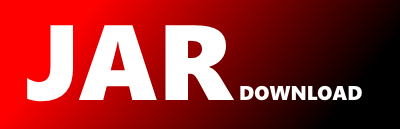
io.ciera.tool.sql.ooaofooa.association.impl.ClassAsSimpleParticipantImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.association.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.association.ClassAsSimpleParticipant;
import io.ciera.tool.sql.ooaofooa.association.ReferredToClassInAssoc;
import io.ciera.tool.sql.ooaofooa.association.SimpleAssociation;
import io.ciera.tool.sql.ooaofooa.association.impl.ReferredToClassInAssocImpl;
import io.ciera.tool.sql.ooaofooa.association.impl.SimpleAssociationImpl;
public class ClassAsSimpleParticipantImpl extends ModelInstance implements ClassAsSimpleParticipant {
public static final String KEY_LETTERS = "R_PART";
public static final ClassAsSimpleParticipant EMPTY_CLASSASSIMPLEPARTICIPANT = new EmptyClassAsSimpleParticipant();
private Sql context;
// constructors
private ClassAsSimpleParticipantImpl( Sql context ) {
this.context = context;
ref_Obj_ID = UniqueId.random();
ref_Rel_ID = UniqueId.random();
ref_OIR_ID = UniqueId.random();
m_Mult = 0;
m_Cond = 0;
m_Txt_Phrs = "";
R204_is_a_ReferredToClassInAssoc_inst = ReferredToClassInAssocImpl.EMPTY_REFERREDTOCLASSINASSOC;
R207_is_related_to_formalizer_via_SimpleAssociation_inst = SimpleAssociationImpl.EMPTY_SIMPLEASSOCIATION;
}
private ClassAsSimpleParticipantImpl( Sql context, UniqueId instanceId, UniqueId ref_Obj_ID, UniqueId ref_Rel_ID, UniqueId ref_OIR_ID, int m_Mult, int m_Cond, String m_Txt_Phrs ) {
super(instanceId);
this.context = context;
this.ref_Obj_ID = ref_Obj_ID;
this.ref_Rel_ID = ref_Rel_ID;
this.ref_OIR_ID = ref_OIR_ID;
this.m_Mult = m_Mult;
this.m_Cond = m_Cond;
this.m_Txt_Phrs = m_Txt_Phrs;
R204_is_a_ReferredToClassInAssoc_inst = ReferredToClassInAssocImpl.EMPTY_REFERREDTOCLASSINASSOC;
R207_is_related_to_formalizer_via_SimpleAssociation_inst = SimpleAssociationImpl.EMPTY_SIMPLEASSOCIATION;
}
public static ClassAsSimpleParticipant create( Sql context ) throws XtumlException {
ClassAsSimpleParticipant newClassAsSimpleParticipant = new ClassAsSimpleParticipantImpl( context );
if ( context.addInstance( newClassAsSimpleParticipant ) ) {
newClassAsSimpleParticipant.getRunContext().addChange(new InstanceCreatedDelta(newClassAsSimpleParticipant, KEY_LETTERS));
return newClassAsSimpleParticipant;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static ClassAsSimpleParticipant create( Sql context, UniqueId ref_Obj_ID, UniqueId ref_Rel_ID, UniqueId ref_OIR_ID, int m_Mult, int m_Cond, String m_Txt_Phrs ) throws XtumlException {
return create(context, UniqueId.random(), ref_Obj_ID, ref_Rel_ID, ref_OIR_ID, m_Mult, m_Cond, m_Txt_Phrs);
}
public static ClassAsSimpleParticipant create( Sql context, UniqueId instanceId, UniqueId ref_Obj_ID, UniqueId ref_Rel_ID, UniqueId ref_OIR_ID, int m_Mult, int m_Cond, String m_Txt_Phrs ) throws XtumlException {
ClassAsSimpleParticipant newClassAsSimpleParticipant = new ClassAsSimpleParticipantImpl( context, instanceId, ref_Obj_ID, ref_Rel_ID, ref_OIR_ID, m_Mult, m_Cond, m_Txt_Phrs );
if ( context.addInstance( newClassAsSimpleParticipant ) ) {
return newClassAsSimpleParticipant;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Obj_ID;
@Override
public void setObj_ID(UniqueId ref_Obj_ID) throws XtumlException {
checkLiving();
if (ref_Obj_ID.inequality( this.ref_Obj_ID)) {
final UniqueId oldValue = this.ref_Obj_ID;
this.ref_Obj_ID = ref_Obj_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Obj_ID", oldValue, this.ref_Obj_ID));
}
}
@Override
public UniqueId getObj_ID() throws XtumlException {
checkLiving();
return ref_Obj_ID;
}
private UniqueId ref_Rel_ID;
@Override
public UniqueId getRel_ID() throws XtumlException {
checkLiving();
return ref_Rel_ID;
}
@Override
public void setRel_ID(UniqueId ref_Rel_ID) throws XtumlException {
checkLiving();
if (ref_Rel_ID.inequality( this.ref_Rel_ID)) {
final UniqueId oldValue = this.ref_Rel_ID;
this.ref_Rel_ID = ref_Rel_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Rel_ID", oldValue, this.ref_Rel_ID));
}
}
private UniqueId ref_OIR_ID;
@Override
public UniqueId getOIR_ID() throws XtumlException {
checkLiving();
return ref_OIR_ID;
}
@Override
public void setOIR_ID(UniqueId ref_OIR_ID) throws XtumlException {
checkLiving();
if (ref_OIR_ID.inequality( this.ref_OIR_ID)) {
final UniqueId oldValue = this.ref_OIR_ID;
this.ref_OIR_ID = ref_OIR_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_OIR_ID", oldValue, this.ref_OIR_ID));
}
}
private int m_Mult;
@Override
public void setMult(int m_Mult) throws XtumlException {
checkLiving();
if (m_Mult != this.m_Mult) {
final int oldValue = this.m_Mult;
this.m_Mult = m_Mult;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Mult", oldValue, this.m_Mult));
}
}
@Override
public int getMult() throws XtumlException {
checkLiving();
return m_Mult;
}
private int m_Cond;
@Override
public int getCond() throws XtumlException {
checkLiving();
return m_Cond;
}
@Override
public void setCond(int m_Cond) throws XtumlException {
checkLiving();
if (m_Cond != this.m_Cond) {
final int oldValue = this.m_Cond;
this.m_Cond = m_Cond;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Cond", oldValue, this.m_Cond));
}
}
private String m_Txt_Phrs;
@Override
public void setTxt_Phrs(String m_Txt_Phrs) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Txt_Phrs, this.m_Txt_Phrs)) {
final String oldValue = this.m_Txt_Phrs;
this.m_Txt_Phrs = m_Txt_Phrs;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Txt_Phrs", oldValue, this.m_Txt_Phrs));
}
}
@Override
public String getTxt_Phrs() throws XtumlException {
checkLiving();
return m_Txt_Phrs;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getObj_ID(), getRel_ID(), getOIR_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private ReferredToClassInAssoc R204_is_a_ReferredToClassInAssoc_inst;
@Override
public void setR204_is_a_ReferredToClassInAssoc( ReferredToClassInAssoc inst ) {
R204_is_a_ReferredToClassInAssoc_inst = inst;
}
@Override
public ReferredToClassInAssoc R204_is_a_ReferredToClassInAssoc() throws XtumlException {
return R204_is_a_ReferredToClassInAssoc_inst;
}
private SimpleAssociation R207_is_related_to_formalizer_via_SimpleAssociation_inst;
@Override
public void setR207_is_related_to_formalizer_via_SimpleAssociation( SimpleAssociation inst ) {
R207_is_related_to_formalizer_via_SimpleAssociation_inst = inst;
}
@Override
public SimpleAssociation R207_is_related_to_formalizer_via_SimpleAssociation() throws XtumlException {
return R207_is_related_to_formalizer_via_SimpleAssociation_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public ClassAsSimpleParticipant self() {
return this;
}
@Override
public ClassAsSimpleParticipant oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_CLASSASSIMPLEPARTICIPANT;
}
}
class EmptyClassAsSimpleParticipant extends ModelInstance implements ClassAsSimpleParticipant {
// attributes
public void setObj_ID( UniqueId ref_Obj_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getObj_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getRel_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRel_ID( UniqueId ref_Rel_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getOIR_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setOIR_ID( UniqueId ref_OIR_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setMult( int m_Mult ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getMult() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getCond() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCond( int m_Cond ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setTxt_Phrs( String m_Txt_Phrs ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getTxt_Phrs() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public ReferredToClassInAssoc R204_is_a_ReferredToClassInAssoc() {
return ReferredToClassInAssocImpl.EMPTY_REFERREDTOCLASSINASSOC;
}
@Override
public SimpleAssociation R207_is_related_to_formalizer_via_SimpleAssociation() {
return SimpleAssociationImpl.EMPTY_SIMPLEASSOCIATION;
}
@Override
public String getKeyLetters() {
return ClassAsSimpleParticipantImpl.KEY_LETTERS;
}
@Override
public ClassAsSimpleParticipant self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public ClassAsSimpleParticipant oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return ClassAsSimpleParticipantImpl.EMPTY_CLASSASSIMPLEPARTICIPANT;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy