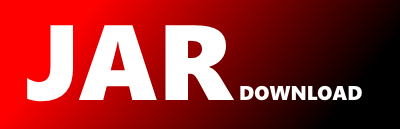
io.ciera.tool.sql.ooaofooa.body.Block Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.body;
import io.ciera.runtime.summit.classes.IModelInstance;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.body.ACT_SMT;
import io.ciera.tool.sql.ooaofooa.body.ACT_SMTSet;
import io.ciera.tool.sql.ooaofooa.body.Body;
import io.ciera.tool.sql.ooaofooa.body.ElseIfStmt;
import io.ciera.tool.sql.ooaofooa.body.ElseStmt;
import io.ciera.tool.sql.ooaofooa.body.ForStmt;
import io.ciera.tool.sql.ooaofooa.body.IfStmt;
import io.ciera.tool.sql.ooaofooa.body.WhileStmt;
import io.ciera.tool.sql.ooaofooa.instance.BlockInStackFrame;
import io.ciera.tool.sql.ooaofooa.instance.BlockInStackFrameSet;
import io.ciera.tool.sql.ooaofooa.value.V_VAR;
import io.ciera.tool.sql.ooaofooa.value.V_VARSet;
import io.ciera.tool.sql.ooaofooa.value.Value;
import io.ciera.tool.sql.ooaofooa.value.ValueSet;
public interface Block extends IModelInstance {
// attributes
public void setBlock_ID( UniqueId m_Block_ID ) throws XtumlException;
public UniqueId getBlock_ID() throws XtumlException;
public boolean getWhereSpecOK() throws XtumlException;
public void setWhereSpecOK( boolean m_WhereSpecOK ) throws XtumlException;
public void setInWhereSpec( boolean m_InWhereSpec ) throws XtumlException;
public boolean getInWhereSpec() throws XtumlException;
public void setSelectedFound( boolean m_SelectedFound ) throws XtumlException;
public boolean getSelectedFound() throws XtumlException;
public String getTempBuffer() throws XtumlException;
public void setTempBuffer( String m_TempBuffer ) throws XtumlException;
public void setSupData1( String m_SupData1 ) throws XtumlException;
public String getSupData1() throws XtumlException;
public void setSupData2( String m_SupData2 ) throws XtumlException;
public String getSupData2() throws XtumlException;
public void setCurrentLine( int m_CurrentLine ) throws XtumlException;
public int getCurrentLine() throws XtumlException;
public int getCurrentCol() throws XtumlException;
public void setCurrentCol( int m_CurrentCol ) throws XtumlException;
public int getCurrentKeyLettersLineNumber() throws XtumlException;
public void setCurrentKeyLettersLineNumber( int m_currentKeyLettersLineNumber ) throws XtumlException;
public int getCurrentKeyLettersColumn() throws XtumlException;
public void setCurrentKeyLettersColumn( int m_currentKeyLettersColumn ) throws XtumlException;
public int getCurrentParameterAssignmentNameLineNumber() throws XtumlException;
public void setCurrentParameterAssignmentNameLineNumber( int m_currentParameterAssignmentNameLineNumber ) throws XtumlException;
public int getCurrentParameterAssignmentNameColumn() throws XtumlException;
public void setCurrentParameterAssignmentNameColumn( int m_currentParameterAssignmentNameColumn ) throws XtumlException;
public void setCurrentAssociationNumberLineNumber( int m_currentAssociationNumberLineNumber ) throws XtumlException;
public int getCurrentAssociationNumberLineNumber() throws XtumlException;
public int getCurrentAssociationNumberColumn() throws XtumlException;
public void setCurrentAssociationNumberColumn( int m_currentAssociationNumberColumn ) throws XtumlException;
public int getCurrentAssociationPhraseLineNumber() throws XtumlException;
public void setCurrentAssociationPhraseLineNumber( int m_currentAssociationPhraseLineNumber ) throws XtumlException;
public void setCurrentAssociationPhraseColumn( int m_currentAssociationPhraseColumn ) throws XtumlException;
public int getCurrentAssociationPhraseColumn() throws XtumlException;
public int getCurrentDataTypeNameLineNumber() throws XtumlException;
public void setCurrentDataTypeNameLineNumber( int m_currentDataTypeNameLineNumber ) throws XtumlException;
public void setCurrentDataTypeNameColumn( int m_currentDataTypeNameColumn ) throws XtumlException;
public int getCurrentDataTypeNameColumn() throws XtumlException;
public void setBlockInStackFrameCreated( boolean m_blockInStackFrameCreated ) throws XtumlException;
public boolean getBlockInStackFrameCreated() throws XtumlException;
public UniqueId getAction_ID() throws XtumlException;
public void setAction_ID( UniqueId ref_Action_ID ) throws XtumlException;
public void setParsed_Action_ID( UniqueId ref_Parsed_Action_ID ) throws XtumlException;
public UniqueId getParsed_Action_ID() throws XtumlException;
// operations
// selections
default public void addR2923_is_executed_within_the_context_of_BlockInStackFrame( BlockInStackFrame inst ) {}
default public void removeR2923_is_executed_within_the_context_of_BlockInStackFrame( BlockInStackFrame inst ) {}
public BlockInStackFrameSet R2923_is_executed_within_the_context_of_BlockInStackFrame() throws XtumlException;
default public void setR601_is_committed_from_Body( Body inst ) {}
public Body R601_is_committed_from_Body() throws XtumlException;
default public void addR602_contained_by_ACT_SMT( ACT_SMT inst ) {}
default public void removeR602_contained_by_ACT_SMT( ACT_SMT inst ) {}
public ACT_SMTSet R602_contained_by_ACT_SMT() throws XtumlException;
default public void setR605_ForStmt( ForStmt inst ) {}
public ForStmt R605_ForStmt() throws XtumlException;
default public void setR606_ElseStmt( ElseStmt inst ) {}
public ElseStmt R606_ElseStmt() throws XtumlException;
default public void setR607_IfStmt( IfStmt inst ) {}
public IfStmt R607_IfStmt() throws XtumlException;
default public void setR608_WhileStmt( WhileStmt inst ) {}
public WhileStmt R608_WhileStmt() throws XtumlException;
default public void setR612_is_parsed_from_Body( Body inst ) {}
public Body R612_is_parsed_from_Body() throws XtumlException;
default public void setR650_is_outer_parse_level_of_Body( Body inst ) {}
public Body R650_is_outer_parse_level_of_Body() throws XtumlException;
default public void setR658_ElseIfStmt( ElseIfStmt inst ) {}
public ElseIfStmt R658_ElseIfStmt() throws XtumlException;
default public void setR666_is_outer_committed_level_of_Body( Body inst ) {}
public Body R666_is_outer_committed_level_of_Body() throws XtumlException;
default public void setR699_is_current_scope_for_Body( Body inst ) {}
public Body R699_is_current_scope_for_Body() throws XtumlException;
default public void addR823_is_scope_for_V_VAR( V_VAR inst ) {}
default public void removeR823_is_scope_for_V_VAR( V_VAR inst ) {}
public V_VARSet R823_is_scope_for_V_VAR() throws XtumlException;
default public void addR826_defines_scope_of_Value( Value inst ) {}
default public void removeR826_defines_scope_of_Value( Value inst ) {}
public ValueSet R826_defines_scope_of_Value() throws XtumlException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy