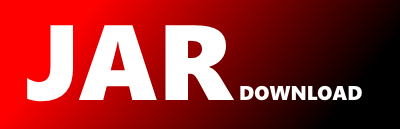
io.ciera.tool.sql.ooaofooa.body.impl.BlockImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.body.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.body.ACT_SMT;
import io.ciera.tool.sql.ooaofooa.body.ACT_SMTSet;
import io.ciera.tool.sql.ooaofooa.body.Block;
import io.ciera.tool.sql.ooaofooa.body.Body;
import io.ciera.tool.sql.ooaofooa.body.ElseIfStmt;
import io.ciera.tool.sql.ooaofooa.body.ElseStmt;
import io.ciera.tool.sql.ooaofooa.body.ForStmt;
import io.ciera.tool.sql.ooaofooa.body.IfStmt;
import io.ciera.tool.sql.ooaofooa.body.WhileStmt;
import io.ciera.tool.sql.ooaofooa.body.impl.ACT_SMTSetImpl;
import io.ciera.tool.sql.ooaofooa.body.impl.BodyImpl;
import io.ciera.tool.sql.ooaofooa.body.impl.ElseIfStmtImpl;
import io.ciera.tool.sql.ooaofooa.body.impl.ElseStmtImpl;
import io.ciera.tool.sql.ooaofooa.body.impl.ForStmtImpl;
import io.ciera.tool.sql.ooaofooa.body.impl.IfStmtImpl;
import io.ciera.tool.sql.ooaofooa.body.impl.WhileStmtImpl;
import io.ciera.tool.sql.ooaofooa.instance.BlockInStackFrame;
import io.ciera.tool.sql.ooaofooa.instance.BlockInStackFrameSet;
import io.ciera.tool.sql.ooaofooa.instance.impl.BlockInStackFrameSetImpl;
import io.ciera.tool.sql.ooaofooa.value.V_VAR;
import io.ciera.tool.sql.ooaofooa.value.V_VARSet;
import io.ciera.tool.sql.ooaofooa.value.Value;
import io.ciera.tool.sql.ooaofooa.value.ValueSet;
import io.ciera.tool.sql.ooaofooa.value.impl.V_VARSetImpl;
import io.ciera.tool.sql.ooaofooa.value.impl.ValueSetImpl;
public class BlockImpl extends ModelInstance implements Block {
public static final String KEY_LETTERS = "ACT_BLK";
public static final Block EMPTY_BLOCK = new EmptyBlock();
private Sql context;
// constructors
private BlockImpl( Sql context ) {
this.context = context;
m_Block_ID = UniqueId.random();
m_WhereSpecOK = false;
m_InWhereSpec = false;
m_SelectedFound = false;
m_TempBuffer = "";
m_SupData1 = "";
m_SupData2 = "";
m_CurrentLine = 0;
m_CurrentCol = 0;
m_currentKeyLettersLineNumber = 0;
m_currentKeyLettersColumn = 0;
m_currentParameterAssignmentNameLineNumber = 0;
m_currentParameterAssignmentNameColumn = 0;
m_currentAssociationNumberLineNumber = 0;
m_currentAssociationNumberColumn = 0;
m_currentAssociationPhraseLineNumber = 0;
m_currentAssociationPhraseColumn = 0;
m_currentDataTypeNameLineNumber = 0;
m_currentDataTypeNameColumn = 0;
m_blockInStackFrameCreated = false;
ref_Action_ID = UniqueId.random();
ref_Parsed_Action_ID = UniqueId.random();
R2923_is_executed_within_the_context_of_BlockInStackFrame_set = new BlockInStackFrameSetImpl();
R601_is_committed_from_Body_inst = BodyImpl.EMPTY_BODY;
R602_contained_by_ACT_SMT_set = new ACT_SMTSetImpl();
R605_ForStmt_inst = ForStmtImpl.EMPTY_FORSTMT;
R606_ElseStmt_inst = ElseStmtImpl.EMPTY_ELSESTMT;
R607_IfStmt_inst = IfStmtImpl.EMPTY_IFSTMT;
R608_WhileStmt_inst = WhileStmtImpl.EMPTY_WHILESTMT;
R612_is_parsed_from_Body_inst = BodyImpl.EMPTY_BODY;
R650_is_outer_parse_level_of_Body_inst = BodyImpl.EMPTY_BODY;
R658_ElseIfStmt_inst = ElseIfStmtImpl.EMPTY_ELSEIFSTMT;
R666_is_outer_committed_level_of_Body_inst = BodyImpl.EMPTY_BODY;
R699_is_current_scope_for_Body_inst = BodyImpl.EMPTY_BODY;
R823_is_scope_for_V_VAR_set = new V_VARSetImpl();
R826_defines_scope_of_Value_set = new ValueSetImpl();
}
private BlockImpl( Sql context, UniqueId instanceId, UniqueId m_Block_ID, boolean m_WhereSpecOK, boolean m_InWhereSpec, boolean m_SelectedFound, String m_TempBuffer, String m_SupData1, String m_SupData2, int m_CurrentLine, int m_CurrentCol, int m_currentKeyLettersLineNumber, int m_currentKeyLettersColumn, int m_currentParameterAssignmentNameLineNumber, int m_currentParameterAssignmentNameColumn, int m_currentAssociationNumberLineNumber, int m_currentAssociationNumberColumn, int m_currentAssociationPhraseLineNumber, int m_currentAssociationPhraseColumn, int m_currentDataTypeNameLineNumber, int m_currentDataTypeNameColumn, boolean m_blockInStackFrameCreated, UniqueId ref_Action_ID, UniqueId ref_Parsed_Action_ID ) {
super(instanceId);
this.context = context;
this.m_Block_ID = m_Block_ID;
this.m_WhereSpecOK = m_WhereSpecOK;
this.m_InWhereSpec = m_InWhereSpec;
this.m_SelectedFound = m_SelectedFound;
this.m_TempBuffer = m_TempBuffer;
this.m_SupData1 = m_SupData1;
this.m_SupData2 = m_SupData2;
this.m_CurrentLine = m_CurrentLine;
this.m_CurrentCol = m_CurrentCol;
this.m_currentKeyLettersLineNumber = m_currentKeyLettersLineNumber;
this.m_currentKeyLettersColumn = m_currentKeyLettersColumn;
this.m_currentParameterAssignmentNameLineNumber = m_currentParameterAssignmentNameLineNumber;
this.m_currentParameterAssignmentNameColumn = m_currentParameterAssignmentNameColumn;
this.m_currentAssociationNumberLineNumber = m_currentAssociationNumberLineNumber;
this.m_currentAssociationNumberColumn = m_currentAssociationNumberColumn;
this.m_currentAssociationPhraseLineNumber = m_currentAssociationPhraseLineNumber;
this.m_currentAssociationPhraseColumn = m_currentAssociationPhraseColumn;
this.m_currentDataTypeNameLineNumber = m_currentDataTypeNameLineNumber;
this.m_currentDataTypeNameColumn = m_currentDataTypeNameColumn;
this.m_blockInStackFrameCreated = m_blockInStackFrameCreated;
this.ref_Action_ID = ref_Action_ID;
this.ref_Parsed_Action_ID = ref_Parsed_Action_ID;
R2923_is_executed_within_the_context_of_BlockInStackFrame_set = new BlockInStackFrameSetImpl();
R601_is_committed_from_Body_inst = BodyImpl.EMPTY_BODY;
R602_contained_by_ACT_SMT_set = new ACT_SMTSetImpl();
R605_ForStmt_inst = ForStmtImpl.EMPTY_FORSTMT;
R606_ElseStmt_inst = ElseStmtImpl.EMPTY_ELSESTMT;
R607_IfStmt_inst = IfStmtImpl.EMPTY_IFSTMT;
R608_WhileStmt_inst = WhileStmtImpl.EMPTY_WHILESTMT;
R612_is_parsed_from_Body_inst = BodyImpl.EMPTY_BODY;
R650_is_outer_parse_level_of_Body_inst = BodyImpl.EMPTY_BODY;
R658_ElseIfStmt_inst = ElseIfStmtImpl.EMPTY_ELSEIFSTMT;
R666_is_outer_committed_level_of_Body_inst = BodyImpl.EMPTY_BODY;
R699_is_current_scope_for_Body_inst = BodyImpl.EMPTY_BODY;
R823_is_scope_for_V_VAR_set = new V_VARSetImpl();
R826_defines_scope_of_Value_set = new ValueSetImpl();
}
public static Block create( Sql context ) throws XtumlException {
Block newBlock = new BlockImpl( context );
if ( context.addInstance( newBlock ) ) {
newBlock.getRunContext().addChange(new InstanceCreatedDelta(newBlock, KEY_LETTERS));
return newBlock;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Block create( Sql context, UniqueId m_Block_ID, boolean m_WhereSpecOK, boolean m_InWhereSpec, boolean m_SelectedFound, String m_TempBuffer, String m_SupData1, String m_SupData2, int m_CurrentLine, int m_CurrentCol, int m_currentKeyLettersLineNumber, int m_currentKeyLettersColumn, int m_currentParameterAssignmentNameLineNumber, int m_currentParameterAssignmentNameColumn, int m_currentAssociationNumberLineNumber, int m_currentAssociationNumberColumn, int m_currentAssociationPhraseLineNumber, int m_currentAssociationPhraseColumn, int m_currentDataTypeNameLineNumber, int m_currentDataTypeNameColumn, boolean m_blockInStackFrameCreated, UniqueId ref_Action_ID, UniqueId ref_Parsed_Action_ID ) throws XtumlException {
return create(context, UniqueId.random(), m_Block_ID, m_WhereSpecOK, m_InWhereSpec, m_SelectedFound, m_TempBuffer, m_SupData1, m_SupData2, m_CurrentLine, m_CurrentCol, m_currentKeyLettersLineNumber, m_currentKeyLettersColumn, m_currentParameterAssignmentNameLineNumber, m_currentParameterAssignmentNameColumn, m_currentAssociationNumberLineNumber, m_currentAssociationNumberColumn, m_currentAssociationPhraseLineNumber, m_currentAssociationPhraseColumn, m_currentDataTypeNameLineNumber, m_currentDataTypeNameColumn, m_blockInStackFrameCreated, ref_Action_ID, ref_Parsed_Action_ID);
}
public static Block create( Sql context, UniqueId instanceId, UniqueId m_Block_ID, boolean m_WhereSpecOK, boolean m_InWhereSpec, boolean m_SelectedFound, String m_TempBuffer, String m_SupData1, String m_SupData2, int m_CurrentLine, int m_CurrentCol, int m_currentKeyLettersLineNumber, int m_currentKeyLettersColumn, int m_currentParameterAssignmentNameLineNumber, int m_currentParameterAssignmentNameColumn, int m_currentAssociationNumberLineNumber, int m_currentAssociationNumberColumn, int m_currentAssociationPhraseLineNumber, int m_currentAssociationPhraseColumn, int m_currentDataTypeNameLineNumber, int m_currentDataTypeNameColumn, boolean m_blockInStackFrameCreated, UniqueId ref_Action_ID, UniqueId ref_Parsed_Action_ID ) throws XtumlException {
Block newBlock = new BlockImpl( context, instanceId, m_Block_ID, m_WhereSpecOK, m_InWhereSpec, m_SelectedFound, m_TempBuffer, m_SupData1, m_SupData2, m_CurrentLine, m_CurrentCol, m_currentKeyLettersLineNumber, m_currentKeyLettersColumn, m_currentParameterAssignmentNameLineNumber, m_currentParameterAssignmentNameColumn, m_currentAssociationNumberLineNumber, m_currentAssociationNumberColumn, m_currentAssociationPhraseLineNumber, m_currentAssociationPhraseColumn, m_currentDataTypeNameLineNumber, m_currentDataTypeNameColumn, m_blockInStackFrameCreated, ref_Action_ID, ref_Parsed_Action_ID );
if ( context.addInstance( newBlock ) ) {
return newBlock;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Block_ID;
@Override
public void setBlock_ID(UniqueId m_Block_ID) throws XtumlException {
checkLiving();
if (m_Block_ID.inequality( this.m_Block_ID)) {
final UniqueId oldValue = this.m_Block_ID;
this.m_Block_ID = m_Block_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Block_ID", oldValue, this.m_Block_ID));
if ( !R602_contained_by_ACT_SMT().isEmpty() ) R602_contained_by_ACT_SMT().setBlock_ID( m_Block_ID );
if ( !R607_IfStmt().isEmpty() ) R607_IfStmt().setBlock_ID( m_Block_ID );
if ( !R699_is_current_scope_for_Body().isEmpty() ) R699_is_current_scope_for_Body().setCurrentScope_ID( m_Block_ID );
if ( !R608_WhileStmt().isEmpty() ) R608_WhileStmt().setBlock_ID( m_Block_ID );
if ( !R606_ElseStmt().isEmpty() ) R606_ElseStmt().setBlock_ID( m_Block_ID );
if ( !R826_defines_scope_of_Value().isEmpty() ) R826_defines_scope_of_Value().setBlock_ID( m_Block_ID );
if ( !R2923_is_executed_within_the_context_of_BlockInStackFrame().isEmpty() ) R2923_is_executed_within_the_context_of_BlockInStackFrame().setBlock_ID( m_Block_ID );
if ( !R650_is_outer_parse_level_of_Body().isEmpty() ) R650_is_outer_parse_level_of_Body().setParsed_Block_ID( m_Block_ID );
if ( !R605_ForStmt().isEmpty() ) R605_ForStmt().setBlock_ID( m_Block_ID );
if ( !R658_ElseIfStmt().isEmpty() ) R658_ElseIfStmt().setBlock_ID( m_Block_ID );
if ( !R823_is_scope_for_V_VAR().isEmpty() ) R823_is_scope_for_V_VAR().setBlock_ID( m_Block_ID );
if ( !R666_is_outer_committed_level_of_Body().isEmpty() ) R666_is_outer_committed_level_of_Body().setBlock_ID( m_Block_ID );
}
}
@Override
public UniqueId getBlock_ID() throws XtumlException {
checkLiving();
return m_Block_ID;
}
private boolean m_WhereSpecOK;
@Override
public boolean getWhereSpecOK() throws XtumlException {
checkLiving();
return m_WhereSpecOK;
}
@Override
public void setWhereSpecOK(boolean m_WhereSpecOK) throws XtumlException {
checkLiving();
if (m_WhereSpecOK != this.m_WhereSpecOK) {
final boolean oldValue = this.m_WhereSpecOK;
this.m_WhereSpecOK = m_WhereSpecOK;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_WhereSpecOK", oldValue, this.m_WhereSpecOK));
}
}
private boolean m_InWhereSpec;
@Override
public void setInWhereSpec(boolean m_InWhereSpec) throws XtumlException {
checkLiving();
if (m_InWhereSpec != this.m_InWhereSpec) {
final boolean oldValue = this.m_InWhereSpec;
this.m_InWhereSpec = m_InWhereSpec;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_InWhereSpec", oldValue, this.m_InWhereSpec));
}
}
@Override
public boolean getInWhereSpec() throws XtumlException {
checkLiving();
return m_InWhereSpec;
}
private boolean m_SelectedFound;
@Override
public void setSelectedFound(boolean m_SelectedFound) throws XtumlException {
checkLiving();
if (m_SelectedFound != this.m_SelectedFound) {
final boolean oldValue = this.m_SelectedFound;
this.m_SelectedFound = m_SelectedFound;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_SelectedFound", oldValue, this.m_SelectedFound));
}
}
@Override
public boolean getSelectedFound() throws XtumlException {
checkLiving();
return m_SelectedFound;
}
private String m_TempBuffer;
@Override
public String getTempBuffer() throws XtumlException {
checkLiving();
return m_TempBuffer;
}
@Override
public void setTempBuffer(String m_TempBuffer) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_TempBuffer, this.m_TempBuffer)) {
final String oldValue = this.m_TempBuffer;
this.m_TempBuffer = m_TempBuffer;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_TempBuffer", oldValue, this.m_TempBuffer));
}
}
private String m_SupData1;
@Override
public void setSupData1(String m_SupData1) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_SupData1, this.m_SupData1)) {
final String oldValue = this.m_SupData1;
this.m_SupData1 = m_SupData1;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_SupData1", oldValue, this.m_SupData1));
}
}
@Override
public String getSupData1() throws XtumlException {
checkLiving();
return m_SupData1;
}
private String m_SupData2;
@Override
public void setSupData2(String m_SupData2) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_SupData2, this.m_SupData2)) {
final String oldValue = this.m_SupData2;
this.m_SupData2 = m_SupData2;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_SupData2", oldValue, this.m_SupData2));
}
}
@Override
public String getSupData2() throws XtumlException {
checkLiving();
return m_SupData2;
}
private int m_CurrentLine;
@Override
public void setCurrentLine(int m_CurrentLine) throws XtumlException {
checkLiving();
if (m_CurrentLine != this.m_CurrentLine) {
final int oldValue = this.m_CurrentLine;
this.m_CurrentLine = m_CurrentLine;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_CurrentLine", oldValue, this.m_CurrentLine));
}
}
@Override
public int getCurrentLine() throws XtumlException {
checkLiving();
return m_CurrentLine;
}
private int m_CurrentCol;
@Override
public int getCurrentCol() throws XtumlException {
checkLiving();
return m_CurrentCol;
}
@Override
public void setCurrentCol(int m_CurrentCol) throws XtumlException {
checkLiving();
if (m_CurrentCol != this.m_CurrentCol) {
final int oldValue = this.m_CurrentCol;
this.m_CurrentCol = m_CurrentCol;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_CurrentCol", oldValue, this.m_CurrentCol));
}
}
private int m_currentKeyLettersLineNumber;
@Override
public int getCurrentKeyLettersLineNumber() throws XtumlException {
checkLiving();
return m_currentKeyLettersLineNumber;
}
@Override
public void setCurrentKeyLettersLineNumber(int m_currentKeyLettersLineNumber) throws XtumlException {
checkLiving();
if (m_currentKeyLettersLineNumber != this.m_currentKeyLettersLineNumber) {
final int oldValue = this.m_currentKeyLettersLineNumber;
this.m_currentKeyLettersLineNumber = m_currentKeyLettersLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentKeyLettersLineNumber", oldValue, this.m_currentKeyLettersLineNumber));
}
}
private int m_currentKeyLettersColumn;
@Override
public int getCurrentKeyLettersColumn() throws XtumlException {
checkLiving();
return m_currentKeyLettersColumn;
}
@Override
public void setCurrentKeyLettersColumn(int m_currentKeyLettersColumn) throws XtumlException {
checkLiving();
if (m_currentKeyLettersColumn != this.m_currentKeyLettersColumn) {
final int oldValue = this.m_currentKeyLettersColumn;
this.m_currentKeyLettersColumn = m_currentKeyLettersColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentKeyLettersColumn", oldValue, this.m_currentKeyLettersColumn));
}
}
private int m_currentParameterAssignmentNameLineNumber;
@Override
public int getCurrentParameterAssignmentNameLineNumber() throws XtumlException {
checkLiving();
return m_currentParameterAssignmentNameLineNumber;
}
@Override
public void setCurrentParameterAssignmentNameLineNumber(int m_currentParameterAssignmentNameLineNumber) throws XtumlException {
checkLiving();
if (m_currentParameterAssignmentNameLineNumber != this.m_currentParameterAssignmentNameLineNumber) {
final int oldValue = this.m_currentParameterAssignmentNameLineNumber;
this.m_currentParameterAssignmentNameLineNumber = m_currentParameterAssignmentNameLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentParameterAssignmentNameLineNumber", oldValue, this.m_currentParameterAssignmentNameLineNumber));
}
}
private int m_currentParameterAssignmentNameColumn;
@Override
public int getCurrentParameterAssignmentNameColumn() throws XtumlException {
checkLiving();
return m_currentParameterAssignmentNameColumn;
}
@Override
public void setCurrentParameterAssignmentNameColumn(int m_currentParameterAssignmentNameColumn) throws XtumlException {
checkLiving();
if (m_currentParameterAssignmentNameColumn != this.m_currentParameterAssignmentNameColumn) {
final int oldValue = this.m_currentParameterAssignmentNameColumn;
this.m_currentParameterAssignmentNameColumn = m_currentParameterAssignmentNameColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentParameterAssignmentNameColumn", oldValue, this.m_currentParameterAssignmentNameColumn));
}
}
private int m_currentAssociationNumberLineNumber;
@Override
public void setCurrentAssociationNumberLineNumber(int m_currentAssociationNumberLineNumber) throws XtumlException {
checkLiving();
if (m_currentAssociationNumberLineNumber != this.m_currentAssociationNumberLineNumber) {
final int oldValue = this.m_currentAssociationNumberLineNumber;
this.m_currentAssociationNumberLineNumber = m_currentAssociationNumberLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentAssociationNumberLineNumber", oldValue, this.m_currentAssociationNumberLineNumber));
}
}
@Override
public int getCurrentAssociationNumberLineNumber() throws XtumlException {
checkLiving();
return m_currentAssociationNumberLineNumber;
}
private int m_currentAssociationNumberColumn;
@Override
public int getCurrentAssociationNumberColumn() throws XtumlException {
checkLiving();
return m_currentAssociationNumberColumn;
}
@Override
public void setCurrentAssociationNumberColumn(int m_currentAssociationNumberColumn) throws XtumlException {
checkLiving();
if (m_currentAssociationNumberColumn != this.m_currentAssociationNumberColumn) {
final int oldValue = this.m_currentAssociationNumberColumn;
this.m_currentAssociationNumberColumn = m_currentAssociationNumberColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentAssociationNumberColumn", oldValue, this.m_currentAssociationNumberColumn));
}
}
private int m_currentAssociationPhraseLineNumber;
@Override
public int getCurrentAssociationPhraseLineNumber() throws XtumlException {
checkLiving();
return m_currentAssociationPhraseLineNumber;
}
@Override
public void setCurrentAssociationPhraseLineNumber(int m_currentAssociationPhraseLineNumber) throws XtumlException {
checkLiving();
if (m_currentAssociationPhraseLineNumber != this.m_currentAssociationPhraseLineNumber) {
final int oldValue = this.m_currentAssociationPhraseLineNumber;
this.m_currentAssociationPhraseLineNumber = m_currentAssociationPhraseLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentAssociationPhraseLineNumber", oldValue, this.m_currentAssociationPhraseLineNumber));
}
}
private int m_currentAssociationPhraseColumn;
@Override
public void setCurrentAssociationPhraseColumn(int m_currentAssociationPhraseColumn) throws XtumlException {
checkLiving();
if (m_currentAssociationPhraseColumn != this.m_currentAssociationPhraseColumn) {
final int oldValue = this.m_currentAssociationPhraseColumn;
this.m_currentAssociationPhraseColumn = m_currentAssociationPhraseColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentAssociationPhraseColumn", oldValue, this.m_currentAssociationPhraseColumn));
}
}
@Override
public int getCurrentAssociationPhraseColumn() throws XtumlException {
checkLiving();
return m_currentAssociationPhraseColumn;
}
private int m_currentDataTypeNameLineNumber;
@Override
public int getCurrentDataTypeNameLineNumber() throws XtumlException {
checkLiving();
return m_currentDataTypeNameLineNumber;
}
@Override
public void setCurrentDataTypeNameLineNumber(int m_currentDataTypeNameLineNumber) throws XtumlException {
checkLiving();
if (m_currentDataTypeNameLineNumber != this.m_currentDataTypeNameLineNumber) {
final int oldValue = this.m_currentDataTypeNameLineNumber;
this.m_currentDataTypeNameLineNumber = m_currentDataTypeNameLineNumber;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentDataTypeNameLineNumber", oldValue, this.m_currentDataTypeNameLineNumber));
}
}
private int m_currentDataTypeNameColumn;
@Override
public void setCurrentDataTypeNameColumn(int m_currentDataTypeNameColumn) throws XtumlException {
checkLiving();
if (m_currentDataTypeNameColumn != this.m_currentDataTypeNameColumn) {
final int oldValue = this.m_currentDataTypeNameColumn;
this.m_currentDataTypeNameColumn = m_currentDataTypeNameColumn;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_currentDataTypeNameColumn", oldValue, this.m_currentDataTypeNameColumn));
}
}
@Override
public int getCurrentDataTypeNameColumn() throws XtumlException {
checkLiving();
return m_currentDataTypeNameColumn;
}
private boolean m_blockInStackFrameCreated;
@Override
public void setBlockInStackFrameCreated(boolean m_blockInStackFrameCreated) throws XtumlException {
checkLiving();
if (m_blockInStackFrameCreated != this.m_blockInStackFrameCreated) {
final boolean oldValue = this.m_blockInStackFrameCreated;
this.m_blockInStackFrameCreated = m_blockInStackFrameCreated;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_blockInStackFrameCreated", oldValue, this.m_blockInStackFrameCreated));
}
}
@Override
public boolean getBlockInStackFrameCreated() throws XtumlException {
checkLiving();
return m_blockInStackFrameCreated;
}
private UniqueId ref_Action_ID;
@Override
public UniqueId getAction_ID() throws XtumlException {
checkLiving();
return ref_Action_ID;
}
@Override
public void setAction_ID(UniqueId ref_Action_ID) throws XtumlException {
checkLiving();
if (ref_Action_ID.inequality( this.ref_Action_ID)) {
final UniqueId oldValue = this.ref_Action_ID;
this.ref_Action_ID = ref_Action_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Action_ID", oldValue, this.ref_Action_ID));
}
}
private UniqueId ref_Parsed_Action_ID;
@Override
public void setParsed_Action_ID(UniqueId ref_Parsed_Action_ID) throws XtumlException {
checkLiving();
if (ref_Parsed_Action_ID.inequality( this.ref_Parsed_Action_ID)) {
final UniqueId oldValue = this.ref_Parsed_Action_ID;
this.ref_Parsed_Action_ID = ref_Parsed_Action_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Parsed_Action_ID", oldValue, this.ref_Parsed_Action_ID));
}
}
@Override
public UniqueId getParsed_Action_ID() throws XtumlException {
checkLiving();
return ref_Parsed_Action_ID;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getBlock_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private BlockInStackFrameSet R2923_is_executed_within_the_context_of_BlockInStackFrame_set;
@Override
public void addR2923_is_executed_within_the_context_of_BlockInStackFrame( BlockInStackFrame inst ) {
R2923_is_executed_within_the_context_of_BlockInStackFrame_set.add(inst);
}
@Override
public void removeR2923_is_executed_within_the_context_of_BlockInStackFrame( BlockInStackFrame inst ) {
R2923_is_executed_within_the_context_of_BlockInStackFrame_set.remove(inst);
}
@Override
public BlockInStackFrameSet R2923_is_executed_within_the_context_of_BlockInStackFrame() throws XtumlException {
return R2923_is_executed_within_the_context_of_BlockInStackFrame_set;
}
private Body R601_is_committed_from_Body_inst;
@Override
public void setR601_is_committed_from_Body( Body inst ) {
R601_is_committed_from_Body_inst = inst;
}
@Override
public Body R601_is_committed_from_Body() throws XtumlException {
return R601_is_committed_from_Body_inst;
}
private ACT_SMTSet R602_contained_by_ACT_SMT_set;
@Override
public void addR602_contained_by_ACT_SMT( ACT_SMT inst ) {
R602_contained_by_ACT_SMT_set.add(inst);
}
@Override
public void removeR602_contained_by_ACT_SMT( ACT_SMT inst ) {
R602_contained_by_ACT_SMT_set.remove(inst);
}
@Override
public ACT_SMTSet R602_contained_by_ACT_SMT() throws XtumlException {
return R602_contained_by_ACT_SMT_set;
}
private ForStmt R605_ForStmt_inst;
@Override
public void setR605_ForStmt( ForStmt inst ) {
R605_ForStmt_inst = inst;
}
@Override
public ForStmt R605_ForStmt() throws XtumlException {
return R605_ForStmt_inst;
}
private ElseStmt R606_ElseStmt_inst;
@Override
public void setR606_ElseStmt( ElseStmt inst ) {
R606_ElseStmt_inst = inst;
}
@Override
public ElseStmt R606_ElseStmt() throws XtumlException {
return R606_ElseStmt_inst;
}
private IfStmt R607_IfStmt_inst;
@Override
public void setR607_IfStmt( IfStmt inst ) {
R607_IfStmt_inst = inst;
}
@Override
public IfStmt R607_IfStmt() throws XtumlException {
return R607_IfStmt_inst;
}
private WhileStmt R608_WhileStmt_inst;
@Override
public void setR608_WhileStmt( WhileStmt inst ) {
R608_WhileStmt_inst = inst;
}
@Override
public WhileStmt R608_WhileStmt() throws XtumlException {
return R608_WhileStmt_inst;
}
private Body R612_is_parsed_from_Body_inst;
@Override
public void setR612_is_parsed_from_Body( Body inst ) {
R612_is_parsed_from_Body_inst = inst;
}
@Override
public Body R612_is_parsed_from_Body() throws XtumlException {
return R612_is_parsed_from_Body_inst;
}
private Body R650_is_outer_parse_level_of_Body_inst;
@Override
public void setR650_is_outer_parse_level_of_Body( Body inst ) {
R650_is_outer_parse_level_of_Body_inst = inst;
}
@Override
public Body R650_is_outer_parse_level_of_Body() throws XtumlException {
return R650_is_outer_parse_level_of_Body_inst;
}
private ElseIfStmt R658_ElseIfStmt_inst;
@Override
public void setR658_ElseIfStmt( ElseIfStmt inst ) {
R658_ElseIfStmt_inst = inst;
}
@Override
public ElseIfStmt R658_ElseIfStmt() throws XtumlException {
return R658_ElseIfStmt_inst;
}
private Body R666_is_outer_committed_level_of_Body_inst;
@Override
public void setR666_is_outer_committed_level_of_Body( Body inst ) {
R666_is_outer_committed_level_of_Body_inst = inst;
}
@Override
public Body R666_is_outer_committed_level_of_Body() throws XtumlException {
return R666_is_outer_committed_level_of_Body_inst;
}
private Body R699_is_current_scope_for_Body_inst;
@Override
public void setR699_is_current_scope_for_Body( Body inst ) {
R699_is_current_scope_for_Body_inst = inst;
}
@Override
public Body R699_is_current_scope_for_Body() throws XtumlException {
return R699_is_current_scope_for_Body_inst;
}
private V_VARSet R823_is_scope_for_V_VAR_set;
@Override
public void addR823_is_scope_for_V_VAR( V_VAR inst ) {
R823_is_scope_for_V_VAR_set.add(inst);
}
@Override
public void removeR823_is_scope_for_V_VAR( V_VAR inst ) {
R823_is_scope_for_V_VAR_set.remove(inst);
}
@Override
public V_VARSet R823_is_scope_for_V_VAR() throws XtumlException {
return R823_is_scope_for_V_VAR_set;
}
private ValueSet R826_defines_scope_of_Value_set;
@Override
public void addR826_defines_scope_of_Value( Value inst ) {
R826_defines_scope_of_Value_set.add(inst);
}
@Override
public void removeR826_defines_scope_of_Value( Value inst ) {
R826_defines_scope_of_Value_set.remove(inst);
}
@Override
public ValueSet R826_defines_scope_of_Value() throws XtumlException {
return R826_defines_scope_of_Value_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Block self() {
return this;
}
@Override
public Block oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_BLOCK;
}
}
class EmptyBlock extends ModelInstance implements Block {
// attributes
public void setBlock_ID( UniqueId m_Block_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getBlock_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public boolean getWhereSpecOK() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setWhereSpecOK( boolean m_WhereSpecOK ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setInWhereSpec( boolean m_InWhereSpec ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getInWhereSpec() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSelectedFound( boolean m_SelectedFound ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getSelectedFound() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getTempBuffer() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setTempBuffer( String m_TempBuffer ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setSupData1( String m_SupData1 ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getSupData1() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setSupData2( String m_SupData2 ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getSupData2() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentLine( int m_CurrentLine ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentLine() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getCurrentCol() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentCol( int m_CurrentCol ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentKeyLettersLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentKeyLettersLineNumber( int m_currentKeyLettersLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentKeyLettersColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentKeyLettersColumn( int m_currentKeyLettersColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentParameterAssignmentNameLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentParameterAssignmentNameLineNumber( int m_currentParameterAssignmentNameLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentParameterAssignmentNameColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentParameterAssignmentNameColumn( int m_currentParameterAssignmentNameColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setCurrentAssociationNumberLineNumber( int m_currentAssociationNumberLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentAssociationNumberLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getCurrentAssociationNumberColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentAssociationNumberColumn( int m_currentAssociationNumberColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentAssociationPhraseLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentAssociationPhraseLineNumber( int m_currentAssociationPhraseLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setCurrentAssociationPhraseColumn( int m_currentAssociationPhraseColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentAssociationPhraseColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getCurrentDataTypeNameLineNumber() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setCurrentDataTypeNameLineNumber( int m_currentDataTypeNameLineNumber ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setCurrentDataTypeNameColumn( int m_currentDataTypeNameColumn ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getCurrentDataTypeNameColumn() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setBlockInStackFrameCreated( boolean m_blockInStackFrameCreated ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getBlockInStackFrameCreated() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getAction_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAction_ID( UniqueId ref_Action_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setParsed_Action_ID( UniqueId ref_Parsed_Action_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getParsed_Action_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public BlockInStackFrameSet R2923_is_executed_within_the_context_of_BlockInStackFrame() {
return (new BlockInStackFrameSetImpl());
}
@Override
public Body R601_is_committed_from_Body() {
return BodyImpl.EMPTY_BODY;
}
@Override
public ACT_SMTSet R602_contained_by_ACT_SMT() {
return (new ACT_SMTSetImpl());
}
@Override
public ForStmt R605_ForStmt() {
return ForStmtImpl.EMPTY_FORSTMT;
}
@Override
public ElseStmt R606_ElseStmt() {
return ElseStmtImpl.EMPTY_ELSESTMT;
}
@Override
public IfStmt R607_IfStmt() {
return IfStmtImpl.EMPTY_IFSTMT;
}
@Override
public WhileStmt R608_WhileStmt() {
return WhileStmtImpl.EMPTY_WHILESTMT;
}
@Override
public Body R612_is_parsed_from_Body() {
return BodyImpl.EMPTY_BODY;
}
@Override
public Body R650_is_outer_parse_level_of_Body() {
return BodyImpl.EMPTY_BODY;
}
@Override
public ElseIfStmt R658_ElseIfStmt() {
return ElseIfStmtImpl.EMPTY_ELSEIFSTMT;
}
@Override
public Body R666_is_outer_committed_level_of_Body() {
return BodyImpl.EMPTY_BODY;
}
@Override
public Body R699_is_current_scope_for_Body() {
return BodyImpl.EMPTY_BODY;
}
@Override
public V_VARSet R823_is_scope_for_V_VAR() {
return (new V_VARSetImpl());
}
@Override
public ValueSet R826_defines_scope_of_Value() {
return (new ValueSetImpl());
}
@Override
public String getKeyLetters() {
return BlockImpl.KEY_LETTERS;
}
@Override
public Block self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Block oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return BlockImpl.EMPTY_BLOCK;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy