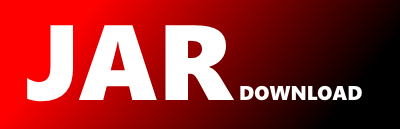
io.ciera.tool.sql.ooaofooa.communication.impl.CommunicationLinkImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.communication.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.association.Association;
import io.ciera.tool.sql.ooaofooa.association.impl.AssociationImpl;
import io.ciera.tool.sql.ooaofooa.communication.CommunicationLink;
import io.ciera.tool.sql.ooaofooa.interaction.InteractionParticipant;
import io.ciera.tool.sql.ooaofooa.interaction.impl.InteractionParticipantImpl;
import ooaofooa.datatypes.Visibility;
public class CommunicationLinkImpl extends ModelInstance implements CommunicationLink {
public static final String KEY_LETTERS = "COMM_LNK";
public static final CommunicationLink EMPTY_COMMUNICATIONLINK = new EmptyCommunicationLink();
private Sql context;
// constructors
private CommunicationLinkImpl( Sql context ) {
this.context = context;
m_Link_ID = UniqueId.random();
ref_Rel_ID = UniqueId.random();
m_Numb = "";
m_Descrip = "";
m_StartText = "";
m_EndText = "";
m_isFormal = false;
m_StartVisibility = Visibility.UNINITIALIZED_ENUM;
m_EndVisibility = Visibility.UNINITIALIZED_ENUM;
ref_Start_Part_ID = UniqueId.random();
ref_Destination_Part_ID = UniqueId.random();
R1128_may_be_formalized_against_Association_inst = AssociationImpl.EMPTY_ASSOCIATION;
R1133_starts_at_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
R1134_is_destined_for_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
}
private CommunicationLinkImpl( Sql context, UniqueId instanceId, UniqueId m_Link_ID, UniqueId ref_Rel_ID, String m_Numb, String m_Descrip, String m_StartText, String m_EndText, boolean m_isFormal, Visibility m_StartVisibility, Visibility m_EndVisibility, UniqueId ref_Start_Part_ID, UniqueId ref_Destination_Part_ID ) {
super(instanceId);
this.context = context;
this.m_Link_ID = m_Link_ID;
this.ref_Rel_ID = ref_Rel_ID;
this.m_Numb = m_Numb;
this.m_Descrip = m_Descrip;
this.m_StartText = m_StartText;
this.m_EndText = m_EndText;
this.m_isFormal = m_isFormal;
this.m_StartVisibility = m_StartVisibility;
this.m_EndVisibility = m_EndVisibility;
this.ref_Start_Part_ID = ref_Start_Part_ID;
this.ref_Destination_Part_ID = ref_Destination_Part_ID;
R1128_may_be_formalized_against_Association_inst = AssociationImpl.EMPTY_ASSOCIATION;
R1133_starts_at_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
R1134_is_destined_for_InteractionParticipant_inst = InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
}
public static CommunicationLink create( Sql context ) throws XtumlException {
CommunicationLink newCommunicationLink = new CommunicationLinkImpl( context );
if ( context.addInstance( newCommunicationLink ) ) {
newCommunicationLink.getRunContext().addChange(new InstanceCreatedDelta(newCommunicationLink, KEY_LETTERS));
return newCommunicationLink;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static CommunicationLink create( Sql context, UniqueId m_Link_ID, UniqueId ref_Rel_ID, String m_Numb, String m_Descrip, String m_StartText, String m_EndText, boolean m_isFormal, Visibility m_StartVisibility, Visibility m_EndVisibility, UniqueId ref_Start_Part_ID, UniqueId ref_Destination_Part_ID ) throws XtumlException {
return create(context, UniqueId.random(), m_Link_ID, ref_Rel_ID, m_Numb, m_Descrip, m_StartText, m_EndText, m_isFormal, m_StartVisibility, m_EndVisibility, ref_Start_Part_ID, ref_Destination_Part_ID);
}
public static CommunicationLink create( Sql context, UniqueId instanceId, UniqueId m_Link_ID, UniqueId ref_Rel_ID, String m_Numb, String m_Descrip, String m_StartText, String m_EndText, boolean m_isFormal, Visibility m_StartVisibility, Visibility m_EndVisibility, UniqueId ref_Start_Part_ID, UniqueId ref_Destination_Part_ID ) throws XtumlException {
CommunicationLink newCommunicationLink = new CommunicationLinkImpl( context, instanceId, m_Link_ID, ref_Rel_ID, m_Numb, m_Descrip, m_StartText, m_EndText, m_isFormal, m_StartVisibility, m_EndVisibility, ref_Start_Part_ID, ref_Destination_Part_ID );
if ( context.addInstance( newCommunicationLink ) ) {
return newCommunicationLink;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Link_ID;
@Override
public UniqueId getLink_ID() throws XtumlException {
checkLiving();
return m_Link_ID;
}
@Override
public void setLink_ID(UniqueId m_Link_ID) throws XtumlException {
checkLiving();
if (m_Link_ID.inequality( this.m_Link_ID)) {
final UniqueId oldValue = this.m_Link_ID;
this.m_Link_ID = m_Link_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Link_ID", oldValue, this.m_Link_ID));
}
}
private UniqueId ref_Rel_ID;
@Override
public UniqueId getRel_ID() throws XtumlException {
checkLiving();
return ref_Rel_ID;
}
@Override
public void setRel_ID(UniqueId ref_Rel_ID) throws XtumlException {
checkLiving();
if (ref_Rel_ID.inequality( this.ref_Rel_ID)) {
final UniqueId oldValue = this.ref_Rel_ID;
this.ref_Rel_ID = ref_Rel_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Rel_ID", oldValue, this.ref_Rel_ID));
}
}
private String m_Numb;
@Override
public void setNumb(String m_Numb) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Numb, this.m_Numb)) {
final String oldValue = this.m_Numb;
this.m_Numb = m_Numb;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Numb", oldValue, this.m_Numb));
}
}
@Override
public String getNumb() throws XtumlException {
checkLiving();
return m_Numb;
}
private String m_Descrip;
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
private String m_StartText;
@Override
public String getStartText() throws XtumlException {
checkLiving();
return m_StartText;
}
@Override
public void setStartText(String m_StartText) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_StartText, this.m_StartText)) {
final String oldValue = this.m_StartText;
this.m_StartText = m_StartText;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_StartText", oldValue, this.m_StartText));
}
}
private String m_EndText;
@Override
public String getEndText() throws XtumlException {
checkLiving();
return m_EndText;
}
@Override
public void setEndText(String m_EndText) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_EndText, this.m_EndText)) {
final String oldValue = this.m_EndText;
this.m_EndText = m_EndText;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_EndText", oldValue, this.m_EndText));
}
}
private boolean m_isFormal;
@Override
public void setIsFormal(boolean m_isFormal) throws XtumlException {
checkLiving();
if (m_isFormal != this.m_isFormal) {
final boolean oldValue = this.m_isFormal;
this.m_isFormal = m_isFormal;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_isFormal", oldValue, this.m_isFormal));
}
}
@Override
public boolean getIsFormal() throws XtumlException {
checkLiving();
return m_isFormal;
}
private Visibility m_StartVisibility;
@Override
public void setStartVisibility(Visibility m_StartVisibility) throws XtumlException {
checkLiving();
if (m_StartVisibility.inequality( this.m_StartVisibility)) {
final Visibility oldValue = this.m_StartVisibility;
this.m_StartVisibility = m_StartVisibility;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_StartVisibility", oldValue, this.m_StartVisibility));
}
}
@Override
public Visibility getStartVisibility() throws XtumlException {
checkLiving();
return m_StartVisibility;
}
private Visibility m_EndVisibility;
@Override
public Visibility getEndVisibility() throws XtumlException {
checkLiving();
return m_EndVisibility;
}
@Override
public void setEndVisibility(Visibility m_EndVisibility) throws XtumlException {
checkLiving();
if (m_EndVisibility.inequality( this.m_EndVisibility)) {
final Visibility oldValue = this.m_EndVisibility;
this.m_EndVisibility = m_EndVisibility;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_EndVisibility", oldValue, this.m_EndVisibility));
}
}
private UniqueId ref_Start_Part_ID;
@Override
public UniqueId getStart_Part_ID() throws XtumlException {
checkLiving();
return ref_Start_Part_ID;
}
@Override
public void setStart_Part_ID(UniqueId ref_Start_Part_ID) throws XtumlException {
checkLiving();
if (ref_Start_Part_ID.inequality( this.ref_Start_Part_ID)) {
final UniqueId oldValue = this.ref_Start_Part_ID;
this.ref_Start_Part_ID = ref_Start_Part_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Start_Part_ID", oldValue, this.ref_Start_Part_ID));
}
}
private UniqueId ref_Destination_Part_ID;
@Override
public void setDestination_Part_ID(UniqueId ref_Destination_Part_ID) throws XtumlException {
checkLiving();
if (ref_Destination_Part_ID.inequality( this.ref_Destination_Part_ID)) {
final UniqueId oldValue = this.ref_Destination_Part_ID;
this.ref_Destination_Part_ID = ref_Destination_Part_ID;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Destination_Part_ID", oldValue, this.ref_Destination_Part_ID));
}
}
@Override
public UniqueId getDestination_Part_ID() throws XtumlException {
checkLiving();
return ref_Destination_Part_ID;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getLink_ID());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private Association R1128_may_be_formalized_against_Association_inst;
@Override
public void setR1128_may_be_formalized_against_Association( Association inst ) {
R1128_may_be_formalized_against_Association_inst = inst;
}
@Override
public Association R1128_may_be_formalized_against_Association() throws XtumlException {
return R1128_may_be_formalized_against_Association_inst;
}
private InteractionParticipant R1133_starts_at_InteractionParticipant_inst;
@Override
public void setR1133_starts_at_InteractionParticipant( InteractionParticipant inst ) {
R1133_starts_at_InteractionParticipant_inst = inst;
}
@Override
public InteractionParticipant R1133_starts_at_InteractionParticipant() throws XtumlException {
return R1133_starts_at_InteractionParticipant_inst;
}
private InteractionParticipant R1134_is_destined_for_InteractionParticipant_inst;
@Override
public void setR1134_is_destined_for_InteractionParticipant( InteractionParticipant inst ) {
R1134_is_destined_for_InteractionParticipant_inst = inst;
}
@Override
public InteractionParticipant R1134_is_destined_for_InteractionParticipant() throws XtumlException {
return R1134_is_destined_for_InteractionParticipant_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public CommunicationLink self() {
return this;
}
@Override
public CommunicationLink oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_COMMUNICATIONLINK;
}
}
class EmptyCommunicationLink extends ModelInstance implements CommunicationLink {
// attributes
public UniqueId getLink_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setLink_ID( UniqueId m_Link_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getRel_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setRel_ID( UniqueId ref_Rel_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setNumb( String m_Numb ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getNumb() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getStartText() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setStartText( String m_StartText ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getEndText() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setEndText( String m_EndText ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setIsFormal( boolean m_isFormal ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getIsFormal() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setStartVisibility( Visibility m_StartVisibility ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public Visibility getStartVisibility() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public Visibility getEndVisibility() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setEndVisibility( Visibility m_EndVisibility ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getStart_Part_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setStart_Part_ID( UniqueId ref_Start_Part_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setDestination_Part_ID( UniqueId ref_Destination_Part_ID ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getDestination_Part_ID() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public Association R1128_may_be_formalized_against_Association() {
return AssociationImpl.EMPTY_ASSOCIATION;
}
@Override
public InteractionParticipant R1133_starts_at_InteractionParticipant() {
return InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
}
@Override
public InteractionParticipant R1134_is_destined_for_InteractionParticipant() {
return InteractionParticipantImpl.EMPTY_INTERACTIONPARTICIPANT;
}
@Override
public String getKeyLetters() {
return CommunicationLinkImpl.KEY_LETTERS;
}
@Override
public CommunicationLink self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public CommunicationLink oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return CommunicationLinkImpl.EMPTY_COMMUNICATIONLINK;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy