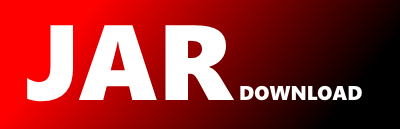
io.ciera.tool.sql.ooaofooa.component.componentlibrary.impl.ComponentReferenceImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.component.componentlibrary.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.component.C_C;
import io.ciera.tool.sql.ooaofooa.component.componentlibrary.ComponentReference;
import io.ciera.tool.sql.ooaofooa.component.componentlibrary.PortReference;
import io.ciera.tool.sql.ooaofooa.component.componentlibrary.PortReferenceSet;
import io.ciera.tool.sql.ooaofooa.component.componentlibrary.impl.PortReferenceSetImpl;
import io.ciera.tool.sql.ooaofooa.component.impl.C_CImpl;
import io.ciera.tool.sql.ooaofooa.instance.ComponentInstance;
import io.ciera.tool.sql.ooaofooa.instance.ComponentInstanceSet;
import io.ciera.tool.sql.ooaofooa.instance.impl.ComponentInstanceSetImpl;
import io.ciera.tool.sql.ooaofooa.packageableelement.PackageableElement;
import io.ciera.tool.sql.ooaofooa.packageableelement.impl.PackageableElementImpl;
public class ComponentReferenceImpl extends ModelInstance implements ComponentReference {
public static final String KEY_LETTERS = "CL_IC";
public static final ComponentReference EMPTY_COMPONENTREFERENCE = new EmptyComponentReference();
private Sql context;
// constructors
private ComponentReferenceImpl( Sql context ) {
this.context = context;
ref_Id = UniqueId.random();
ref_AssignedComp_Id = UniqueId.random();
ref_ParentComp_Id = UniqueId.random();
m_Component_Package_IDdeprecated = UniqueId.random();
m_Mult = 0;
m_ClassifierName = "";
m_Name = "";
m_Descrip = "";
R2963_instance_being_verified_by_ComponentInstance_set = new ComponentInstanceSetImpl();
R4201_represents_C_C_inst = C_CImpl.EMPTY_C_C;
R4205_nested_in_C_C_inst = C_CImpl.EMPTY_C_C;
R4707_communicates_through_PortReference_set = new PortReferenceSetImpl();
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
private ComponentReferenceImpl( Sql context, UniqueId instanceId, UniqueId ref_Id, UniqueId ref_AssignedComp_Id, UniqueId ref_ParentComp_Id, UniqueId m_Component_Package_IDdeprecated, int m_Mult, String m_ClassifierName, String m_Name, String m_Descrip ) {
super(instanceId);
this.context = context;
this.ref_Id = ref_Id;
this.ref_AssignedComp_Id = ref_AssignedComp_Id;
this.ref_ParentComp_Id = ref_ParentComp_Id;
this.m_Component_Package_IDdeprecated = m_Component_Package_IDdeprecated;
this.m_Mult = m_Mult;
this.m_ClassifierName = m_ClassifierName;
this.m_Name = m_Name;
this.m_Descrip = m_Descrip;
R2963_instance_being_verified_by_ComponentInstance_set = new ComponentInstanceSetImpl();
R4201_represents_C_C_inst = C_CImpl.EMPTY_C_C;
R4205_nested_in_C_C_inst = C_CImpl.EMPTY_C_C;
R4707_communicates_through_PortReference_set = new PortReferenceSetImpl();
R8001_is_a_PackageableElement_inst = PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
public static ComponentReference create( Sql context ) throws XtumlException {
ComponentReference newComponentReference = new ComponentReferenceImpl( context );
if ( context.addInstance( newComponentReference ) ) {
newComponentReference.getRunContext().addChange(new InstanceCreatedDelta(newComponentReference, KEY_LETTERS));
return newComponentReference;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static ComponentReference create( Sql context, UniqueId ref_Id, UniqueId ref_AssignedComp_Id, UniqueId ref_ParentComp_Id, UniqueId m_Component_Package_IDdeprecated, int m_Mult, String m_ClassifierName, String m_Name, String m_Descrip ) throws XtumlException {
return create(context, UniqueId.random(), ref_Id, ref_AssignedComp_Id, ref_ParentComp_Id, m_Component_Package_IDdeprecated, m_Mult, m_ClassifierName, m_Name, m_Descrip);
}
public static ComponentReference create( Sql context, UniqueId instanceId, UniqueId ref_Id, UniqueId ref_AssignedComp_Id, UniqueId ref_ParentComp_Id, UniqueId m_Component_Package_IDdeprecated, int m_Mult, String m_ClassifierName, String m_Name, String m_Descrip ) throws XtumlException {
ComponentReference newComponentReference = new ComponentReferenceImpl( context, instanceId, ref_Id, ref_AssignedComp_Id, ref_ParentComp_Id, m_Component_Package_IDdeprecated, m_Mult, m_ClassifierName, m_Name, m_Descrip );
if ( context.addInstance( newComponentReference ) ) {
return newComponentReference;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Id;
@Override
public void setId(UniqueId ref_Id) throws XtumlException {
checkLiving();
if (ref_Id.inequality( this.ref_Id)) {
final UniqueId oldValue = this.ref_Id;
this.ref_Id = ref_Id;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Id", oldValue, this.ref_Id));
if ( !R2963_instance_being_verified_by_ComponentInstance().isEmpty() ) R2963_instance_being_verified_by_ComponentInstance().setImportedComponent_Id( ref_Id );
if ( !R4707_communicates_through_PortReference().isEmpty() ) R4707_communicates_through_PortReference().setCL_IC_Id( ref_Id );
}
}
@Override
public UniqueId getId() throws XtumlException {
checkLiving();
return ref_Id;
}
private UniqueId ref_AssignedComp_Id;
@Override
public UniqueId getAssignedComp_Id() throws XtumlException {
checkLiving();
return ref_AssignedComp_Id;
}
@Override
public void setAssignedComp_Id(UniqueId ref_AssignedComp_Id) throws XtumlException {
checkLiving();
if (ref_AssignedComp_Id.inequality( this.ref_AssignedComp_Id)) {
final UniqueId oldValue = this.ref_AssignedComp_Id;
this.ref_AssignedComp_Id = ref_AssignedComp_Id;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_AssignedComp_Id", oldValue, this.ref_AssignedComp_Id));
}
}
private UniqueId ref_ParentComp_Id;
@Override
public UniqueId getParentComp_Id() throws XtumlException {
checkLiving();
return ref_ParentComp_Id;
}
@Override
public void setParentComp_Id(UniqueId ref_ParentComp_Id) throws XtumlException {
checkLiving();
if (ref_ParentComp_Id.inequality( this.ref_ParentComp_Id)) {
final UniqueId oldValue = this.ref_ParentComp_Id;
this.ref_ParentComp_Id = ref_ParentComp_Id;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_ParentComp_Id", oldValue, this.ref_ParentComp_Id));
}
}
private UniqueId m_Component_Package_IDdeprecated;
@Override
public void setComponent_Package_IDdeprecated(UniqueId m_Component_Package_IDdeprecated) throws XtumlException {
checkLiving();
if (m_Component_Package_IDdeprecated.inequality( this.m_Component_Package_IDdeprecated)) {
final UniqueId oldValue = this.m_Component_Package_IDdeprecated;
this.m_Component_Package_IDdeprecated = m_Component_Package_IDdeprecated;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Component_Package_IDdeprecated", oldValue, this.m_Component_Package_IDdeprecated));
}
}
@Override
public UniqueId getComponent_Package_IDdeprecated() throws XtumlException {
checkLiving();
return m_Component_Package_IDdeprecated;
}
private int m_Mult;
@Override
public int getMult() throws XtumlException {
checkLiving();
return m_Mult;
}
@Override
public void setMult(int m_Mult) throws XtumlException {
checkLiving();
if (m_Mult != this.m_Mult) {
final int oldValue = this.m_Mult;
this.m_Mult = m_Mult;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Mult", oldValue, this.m_Mult));
}
}
private String m_ClassifierName;
@Override
public void setClassifierName(String m_ClassifierName) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_ClassifierName, this.m_ClassifierName)) {
final String oldValue = this.m_ClassifierName;
this.m_ClassifierName = m_ClassifierName;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_ClassifierName", oldValue, this.m_ClassifierName));
}
}
@Override
public String getClassifierName() throws XtumlException {
checkLiving();
return m_ClassifierName;
}
private String m_Name;
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
private String m_Descrip;
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getId());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private ComponentInstanceSet R2963_instance_being_verified_by_ComponentInstance_set;
@Override
public void addR2963_instance_being_verified_by_ComponentInstance( ComponentInstance inst ) {
R2963_instance_being_verified_by_ComponentInstance_set.add(inst);
}
@Override
public void removeR2963_instance_being_verified_by_ComponentInstance( ComponentInstance inst ) {
R2963_instance_being_verified_by_ComponentInstance_set.remove(inst);
}
@Override
public ComponentInstanceSet R2963_instance_being_verified_by_ComponentInstance() throws XtumlException {
return R2963_instance_being_verified_by_ComponentInstance_set;
}
private C_C R4201_represents_C_C_inst;
@Override
public void setR4201_represents_C_C( C_C inst ) {
R4201_represents_C_C_inst = inst;
}
@Override
public C_C R4201_represents_C_C() throws XtumlException {
return R4201_represents_C_C_inst;
}
private C_C R4205_nested_in_C_C_inst;
@Override
public void setR4205_nested_in_C_C( C_C inst ) {
R4205_nested_in_C_C_inst = inst;
}
@Override
public C_C R4205_nested_in_C_C() throws XtumlException {
return R4205_nested_in_C_C_inst;
}
private PortReferenceSet R4707_communicates_through_PortReference_set;
@Override
public void addR4707_communicates_through_PortReference( PortReference inst ) {
R4707_communicates_through_PortReference_set.add(inst);
}
@Override
public void removeR4707_communicates_through_PortReference( PortReference inst ) {
R4707_communicates_through_PortReference_set.remove(inst);
}
@Override
public PortReferenceSet R4707_communicates_through_PortReference() throws XtumlException {
return R4707_communicates_through_PortReference_set;
}
private PackageableElement R8001_is_a_PackageableElement_inst;
@Override
public void setR8001_is_a_PackageableElement( PackageableElement inst ) {
R8001_is_a_PackageableElement_inst = inst;
}
@Override
public PackageableElement R8001_is_a_PackageableElement() throws XtumlException {
return R8001_is_a_PackageableElement_inst;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public ComponentReference self() {
return this;
}
@Override
public ComponentReference oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_COMPONENTREFERENCE;
}
}
class EmptyComponentReference extends ModelInstance implements ComponentReference {
// attributes
public void setId( UniqueId ref_Id ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getId() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public UniqueId getAssignedComp_Id() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setAssignedComp_Id( UniqueId ref_AssignedComp_Id ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getParentComp_Id() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setParentComp_Id( UniqueId ref_ParentComp_Id ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setComponent_Package_IDdeprecated( UniqueId m_Component_Package_IDdeprecated ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getComponent_Package_IDdeprecated() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public int getMult() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setMult( int m_Mult ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setClassifierName( String m_ClassifierName ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getClassifierName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public ComponentInstanceSet R2963_instance_being_verified_by_ComponentInstance() {
return (new ComponentInstanceSetImpl());
}
@Override
public C_C R4201_represents_C_C() {
return C_CImpl.EMPTY_C_C;
}
@Override
public C_C R4205_nested_in_C_C() {
return C_CImpl.EMPTY_C_C;
}
@Override
public PortReferenceSet R4707_communicates_through_PortReference() {
return (new PortReferenceSetImpl());
}
@Override
public PackageableElement R8001_is_a_PackageableElement() {
return PackageableElementImpl.EMPTY_PACKAGEABLEELEMENT;
}
@Override
public String getKeyLetters() {
return ComponentReferenceImpl.KEY_LETTERS;
}
@Override
public ComponentReference self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public ComponentReference oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return ComponentReferenceImpl.EMPTY_COMPONENTREFERENCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy