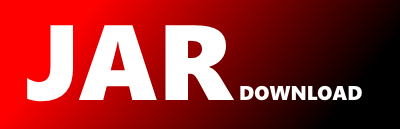
io.ciera.tool.sql.ooaofooa.component.impl.C_POImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.component.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.architecture.interfaces.Port;
import io.ciera.tool.sql.architecture.interfaces.impl.PortImpl;
import io.ciera.tool.sql.ooaofooa.component.C_C;
import io.ciera.tool.sql.ooaofooa.component.C_PO;
import io.ciera.tool.sql.ooaofooa.component.InterfaceReference;
import io.ciera.tool.sql.ooaofooa.component.InterfaceReferenceSet;
import io.ciera.tool.sql.ooaofooa.component.componentlibrary.PortReference;
import io.ciera.tool.sql.ooaofooa.component.componentlibrary.PortReferenceSet;
import io.ciera.tool.sql.ooaofooa.component.componentlibrary.impl.PortReferenceSetImpl;
import io.ciera.tool.sql.ooaofooa.component.impl.C_CImpl;
import io.ciera.tool.sql.ooaofooa.component.impl.InterfaceReferenceSetImpl;
public class C_POImpl extends ModelInstance implements C_PO {
public static final String KEY_LETTERS = "C_PO";
public static final C_PO EMPTY_C_PO = new EmptyC_PO();
private Sql context;
// constructors
private C_POImpl( Sql context ) {
this.context = context;
m_Id = UniqueId.random();
ref_Component_Id = UniqueId.random();
m_Name = "";
m_Mult = 0;
m_DoNotShowPortOnCanvas = false;
m_Key_Lett = "";
R4010_appears_in_C_C_inst = C_CImpl.EMPTY_C_C;
R4016_exposes_InterfaceReference_set = new InterfaceReferenceSetImpl();
R422_Port_inst = PortImpl.EMPTY_PORT;
R4709_is_referenced_by_PortReference_set = new PortReferenceSetImpl();
}
private C_POImpl( Sql context, UniqueId instanceId, UniqueId m_Id, UniqueId ref_Component_Id, String m_Name, int m_Mult, boolean m_DoNotShowPortOnCanvas, String m_Key_Lett ) {
super(instanceId);
this.context = context;
this.m_Id = m_Id;
this.ref_Component_Id = ref_Component_Id;
this.m_Name = m_Name;
this.m_Mult = m_Mult;
this.m_DoNotShowPortOnCanvas = m_DoNotShowPortOnCanvas;
this.m_Key_Lett = m_Key_Lett;
R4010_appears_in_C_C_inst = C_CImpl.EMPTY_C_C;
R4016_exposes_InterfaceReference_set = new InterfaceReferenceSetImpl();
R422_Port_inst = PortImpl.EMPTY_PORT;
R4709_is_referenced_by_PortReference_set = new PortReferenceSetImpl();
}
public static C_PO create( Sql context ) throws XtumlException {
C_PO newC_PO = new C_POImpl( context );
if ( context.addInstance( newC_PO ) ) {
newC_PO.getRunContext().addChange(new InstanceCreatedDelta(newC_PO, KEY_LETTERS));
return newC_PO;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static C_PO create( Sql context, UniqueId m_Id, UniqueId ref_Component_Id, String m_Name, int m_Mult, boolean m_DoNotShowPortOnCanvas, String m_Key_Lett ) throws XtumlException {
return create(context, UniqueId.random(), m_Id, ref_Component_Id, m_Name, m_Mult, m_DoNotShowPortOnCanvas, m_Key_Lett);
}
public static C_PO create( Sql context, UniqueId instanceId, UniqueId m_Id, UniqueId ref_Component_Id, String m_Name, int m_Mult, boolean m_DoNotShowPortOnCanvas, String m_Key_Lett ) throws XtumlException {
C_PO newC_PO = new C_POImpl( context, instanceId, m_Id, ref_Component_Id, m_Name, m_Mult, m_DoNotShowPortOnCanvas, m_Key_Lett );
if ( context.addInstance( newC_PO ) ) {
return newC_PO;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId m_Id;
@Override
public void setId(UniqueId m_Id) throws XtumlException {
checkLiving();
if (m_Id.inequality( this.m_Id)) {
final UniqueId oldValue = this.m_Id;
this.m_Id = m_Id;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Id", oldValue, this.m_Id));
if ( !R4016_exposes_InterfaceReference().isEmpty() ) R4016_exposes_InterfaceReference().setPort_Id( m_Id );
if ( !R4709_is_referenced_by_PortReference().isEmpty() ) R4709_is_referenced_by_PortReference().setC_PO_Id( m_Id );
}
}
@Override
public UniqueId getId() throws XtumlException {
checkLiving();
return m_Id;
}
private UniqueId ref_Component_Id;
@Override
public void setComponent_Id(UniqueId ref_Component_Id) throws XtumlException {
checkLiving();
if (ref_Component_Id.inequality( this.ref_Component_Id)) {
final UniqueId oldValue = this.ref_Component_Id;
this.ref_Component_Id = ref_Component_Id;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Component_Id", oldValue, this.ref_Component_Id));
}
}
@Override
public UniqueId getComponent_Id() throws XtumlException {
checkLiving();
return ref_Component_Id;
}
private String m_Name;
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
private int m_Mult;
@Override
public int getMult() throws XtumlException {
checkLiving();
return m_Mult;
}
@Override
public void setMult(int m_Mult) throws XtumlException {
checkLiving();
if (m_Mult != this.m_Mult) {
final int oldValue = this.m_Mult;
this.m_Mult = m_Mult;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Mult", oldValue, this.m_Mult));
}
}
private boolean m_DoNotShowPortOnCanvas;
@Override
public boolean getDoNotShowPortOnCanvas() throws XtumlException {
checkLiving();
return m_DoNotShowPortOnCanvas;
}
@Override
public void setDoNotShowPortOnCanvas(boolean m_DoNotShowPortOnCanvas) throws XtumlException {
checkLiving();
if (m_DoNotShowPortOnCanvas != this.m_DoNotShowPortOnCanvas) {
final boolean oldValue = this.m_DoNotShowPortOnCanvas;
this.m_DoNotShowPortOnCanvas = m_DoNotShowPortOnCanvas;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_DoNotShowPortOnCanvas", oldValue, this.m_DoNotShowPortOnCanvas));
}
}
private String m_Key_Lett;
@Override
public void setKey_Lett(String m_Key_Lett) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Key_Lett, this.m_Key_Lett)) {
final String oldValue = this.m_Key_Lett;
this.m_Key_Lett = m_Key_Lett;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Key_Lett", oldValue, this.m_Key_Lett));
}
}
@Override
public String getKey_Lett() throws XtumlException {
checkLiving();
return m_Key_Lett;
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getId());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private C_C R4010_appears_in_C_C_inst;
@Override
public void setR4010_appears_in_C_C( C_C inst ) {
R4010_appears_in_C_C_inst = inst;
}
@Override
public C_C R4010_appears_in_C_C() throws XtumlException {
return R4010_appears_in_C_C_inst;
}
private InterfaceReferenceSet R4016_exposes_InterfaceReference_set;
@Override
public void addR4016_exposes_InterfaceReference( InterfaceReference inst ) {
R4016_exposes_InterfaceReference_set.add(inst);
}
@Override
public void removeR4016_exposes_InterfaceReference( InterfaceReference inst ) {
R4016_exposes_InterfaceReference_set.remove(inst);
}
@Override
public InterfaceReferenceSet R4016_exposes_InterfaceReference() throws XtumlException {
return R4016_exposes_InterfaceReference_set;
}
private Port R422_Port_inst;
@Override
public void setR422_Port( Port inst ) {
R422_Port_inst = inst;
}
@Override
public Port R422_Port() throws XtumlException {
return R422_Port_inst;
}
private PortReferenceSet R4709_is_referenced_by_PortReference_set;
@Override
public void addR4709_is_referenced_by_PortReference( PortReference inst ) {
R4709_is_referenced_by_PortReference_set.add(inst);
}
@Override
public void removeR4709_is_referenced_by_PortReference( PortReference inst ) {
R4709_is_referenced_by_PortReference_set.remove(inst);
}
@Override
public PortReferenceSet R4709_is_referenced_by_PortReference() throws XtumlException {
return R4709_is_referenced_by_PortReference_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public C_PO self() {
return this;
}
@Override
public C_PO oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_C_PO;
}
}
class EmptyC_PO extends ModelInstance implements C_PO {
// attributes
public void setId( UniqueId m_Id ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getId() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setComponent_Id( UniqueId ref_Component_Id ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getComponent_Id() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public int getMult() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setMult( int m_Mult ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public boolean getDoNotShowPortOnCanvas() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDoNotShowPortOnCanvas( boolean m_DoNotShowPortOnCanvas ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setKey_Lett( String m_Key_Lett ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getKey_Lett() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
// operations
// selections
@Override
public C_C R4010_appears_in_C_C() {
return C_CImpl.EMPTY_C_C;
}
@Override
public InterfaceReferenceSet R4016_exposes_InterfaceReference() {
return (new InterfaceReferenceSetImpl());
}
@Override
public Port R422_Port() {
return PortImpl.EMPTY_PORT;
}
@Override
public PortReferenceSet R4709_is_referenced_by_PortReference() {
return (new PortReferenceSetImpl());
}
@Override
public String getKeyLetters() {
return C_POImpl.KEY_LETTERS;
}
@Override
public C_PO self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public C_PO oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return C_POImpl.EMPTY_C_PO;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy