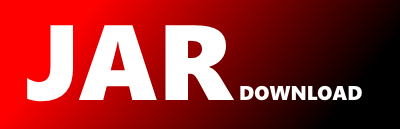
io.ciera.tool.sql.ooaofooa.component.impl.ProvisionImpl Maven / Gradle / Ivy
package io.ciera.tool.sql.ooaofooa.component.impl;
import io.ciera.runtime.instanceloading.AttributeChangedDelta;
import io.ciera.runtime.instanceloading.InstanceCreatedDelta;
import io.ciera.runtime.summit.application.IRunContext;
import io.ciera.runtime.summit.classes.IInstanceIdentifier;
import io.ciera.runtime.summit.classes.InstanceIdentifier;
import io.ciera.runtime.summit.classes.ModelInstance;
import io.ciera.runtime.summit.exceptions.EmptyInstanceException;
import io.ciera.runtime.summit.exceptions.InstancePopulationException;
import io.ciera.runtime.summit.exceptions.XtumlException;
import io.ciera.runtime.summit.types.IWhere;
import io.ciera.runtime.summit.types.IXtumlType;
import io.ciera.runtime.summit.types.StringUtil;
import io.ciera.runtime.summit.types.UniqueId;
import io.ciera.tool.Sql;
import io.ciera.tool.sql.ooaofooa.component.InterfaceReference;
import io.ciera.tool.sql.ooaofooa.component.Provision;
import io.ciera.tool.sql.ooaofooa.component.Satisfaction;
import io.ciera.tool.sql.ooaofooa.component.SatisfactionSet;
import io.ciera.tool.sql.ooaofooa.component.impl.InterfaceReferenceImpl;
import io.ciera.tool.sql.ooaofooa.component.impl.SatisfactionSetImpl;
import io.ciera.tool.sql.ooaofooa.component.signalprovisionsandrequirements.ProvidedExecutableProperty;
import io.ciera.tool.sql.ooaofooa.component.signalprovisionsandrequirements.ProvidedExecutablePropertySet;
import io.ciera.tool.sql.ooaofooa.component.signalprovisionsandrequirements.impl.ProvidedExecutablePropertySetImpl;
public class ProvisionImpl extends ModelInstance implements Provision {
public static final String KEY_LETTERS = "C_P";
public static final Provision EMPTY_PROVISION = new EmptyProvision();
private Sql context;
// constructors
private ProvisionImpl( Sql context ) {
this.context = context;
ref_Provision_Id = UniqueId.random();
m_Name = "";
m_InformalName = "";
m_Descrip = "";
m_pathFromComponent = "";
R4002_defines_required_satisfication_Satisfaction_set = new SatisfactionSetImpl();
R4009_is_a_InterfaceReference_inst = InterfaceReferenceImpl.EMPTY_INTERFACEREFERENCE;
R4501_implements_ProvidedExecutableProperty_set = new ProvidedExecutablePropertySetImpl();
}
private ProvisionImpl( Sql context, UniqueId instanceId, UniqueId ref_Provision_Id, String m_Name, String m_InformalName, String m_Descrip, String m_pathFromComponent ) {
super(instanceId);
this.context = context;
this.ref_Provision_Id = ref_Provision_Id;
this.m_Name = m_Name;
this.m_InformalName = m_InformalName;
this.m_Descrip = m_Descrip;
this.m_pathFromComponent = m_pathFromComponent;
R4002_defines_required_satisfication_Satisfaction_set = new SatisfactionSetImpl();
R4009_is_a_InterfaceReference_inst = InterfaceReferenceImpl.EMPTY_INTERFACEREFERENCE;
R4501_implements_ProvidedExecutableProperty_set = new ProvidedExecutablePropertySetImpl();
}
public static Provision create( Sql context ) throws XtumlException {
Provision newProvision = new ProvisionImpl( context );
if ( context.addInstance( newProvision ) ) {
newProvision.getRunContext().addChange(new InstanceCreatedDelta(newProvision, KEY_LETTERS));
return newProvision;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
public static Provision create( Sql context, UniqueId ref_Provision_Id, String m_Name, String m_InformalName, String m_Descrip, String m_pathFromComponent ) throws XtumlException {
return create(context, UniqueId.random(), ref_Provision_Id, m_Name, m_InformalName, m_Descrip, m_pathFromComponent);
}
public static Provision create( Sql context, UniqueId instanceId, UniqueId ref_Provision_Id, String m_Name, String m_InformalName, String m_Descrip, String m_pathFromComponent ) throws XtumlException {
Provision newProvision = new ProvisionImpl( context, instanceId, ref_Provision_Id, m_Name, m_InformalName, m_Descrip, m_pathFromComponent );
if ( context.addInstance( newProvision ) ) {
return newProvision;
}
else throw new InstancePopulationException( "Instance already exists within this population." );
}
// attributes
private UniqueId ref_Provision_Id;
@Override
public void setProvision_Id(UniqueId ref_Provision_Id) throws XtumlException {
checkLiving();
if (ref_Provision_Id.inequality( this.ref_Provision_Id)) {
final UniqueId oldValue = this.ref_Provision_Id;
this.ref_Provision_Id = ref_Provision_Id;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "ref_Provision_Id", oldValue, this.ref_Provision_Id));
if ( !R4002_defines_required_satisfication_Satisfaction().isEmpty() ) R4002_defines_required_satisfication_Satisfaction().setProvision_Id( ref_Provision_Id );
if ( !R4501_implements_ProvidedExecutableProperty().isEmpty() ) R4501_implements_ProvidedExecutableProperty().setProvision_Id( ref_Provision_Id );
}
}
@Override
public UniqueId getProvision_Id() throws XtumlException {
checkLiving();
return ref_Provision_Id;
}
private String m_Name;
@Override
public String getName() throws XtumlException {
checkLiving();
return m_Name;
}
@Override
public void setName(String m_Name) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Name, this.m_Name)) {
final String oldValue = this.m_Name;
this.m_Name = m_Name;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Name", oldValue, this.m_Name));
}
}
private String m_InformalName;
@Override
public void setInformalName(String m_InformalName) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_InformalName, this.m_InformalName)) {
final String oldValue = this.m_InformalName;
this.m_InformalName = m_InformalName;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_InformalName", oldValue, this.m_InformalName));
}
}
@Override
public String getInformalName() throws XtumlException {
checkLiving();
return m_InformalName;
}
private String m_Descrip;
@Override
public void setDescrip(String m_Descrip) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_Descrip, this.m_Descrip)) {
final String oldValue = this.m_Descrip;
this.m_Descrip = m_Descrip;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_Descrip", oldValue, this.m_Descrip));
}
}
@Override
public String getDescrip() throws XtumlException {
checkLiving();
return m_Descrip;
}
private String m_pathFromComponent;
@Override
public String getPathFromComponent() throws XtumlException {
checkLiving();
return m_pathFromComponent;
}
@Override
public void setPathFromComponent(String m_pathFromComponent) throws XtumlException {
checkLiving();
if (StringUtil.inequality(m_pathFromComponent, this.m_pathFromComponent)) {
final String oldValue = this.m_pathFromComponent;
this.m_pathFromComponent = m_pathFromComponent;
getRunContext().addChange(new AttributeChangedDelta(this, KEY_LETTERS, "m_pathFromComponent", oldValue, this.m_pathFromComponent));
}
}
// instance identifiers
@Override
public IInstanceIdentifier getId1() {
try {
return new InstanceIdentifier(getProvision_Id());
}
catch ( XtumlException e ) {
getRunContext().getLog().error(e);
System.exit(1);
return null;
}
}
// operations
// static operations
// events
// selections
private SatisfactionSet R4002_defines_required_satisfication_Satisfaction_set;
@Override
public void addR4002_defines_required_satisfication_Satisfaction( Satisfaction inst ) {
R4002_defines_required_satisfication_Satisfaction_set.add(inst);
}
@Override
public void removeR4002_defines_required_satisfication_Satisfaction( Satisfaction inst ) {
R4002_defines_required_satisfication_Satisfaction_set.remove(inst);
}
@Override
public SatisfactionSet R4002_defines_required_satisfication_Satisfaction() throws XtumlException {
return R4002_defines_required_satisfication_Satisfaction_set;
}
private InterfaceReference R4009_is_a_InterfaceReference_inst;
@Override
public void setR4009_is_a_InterfaceReference( InterfaceReference inst ) {
R4009_is_a_InterfaceReference_inst = inst;
}
@Override
public InterfaceReference R4009_is_a_InterfaceReference() throws XtumlException {
return R4009_is_a_InterfaceReference_inst;
}
private ProvidedExecutablePropertySet R4501_implements_ProvidedExecutableProperty_set;
@Override
public void addR4501_implements_ProvidedExecutableProperty( ProvidedExecutableProperty inst ) {
R4501_implements_ProvidedExecutableProperty_set.add(inst);
}
@Override
public void removeR4501_implements_ProvidedExecutableProperty( ProvidedExecutableProperty inst ) {
R4501_implements_ProvidedExecutableProperty_set.remove(inst);
}
@Override
public ProvidedExecutablePropertySet R4501_implements_ProvidedExecutableProperty() throws XtumlException {
return R4501_implements_ProvidedExecutableProperty_set;
}
@Override
public IRunContext getRunContext() {
return context().getRunContext();
}
@Override
public Sql context() {
return context;
}
@Override
public String getKeyLetters() {
return KEY_LETTERS;
}
@Override
public Provision self() {
return this;
}
@Override
public Provision oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
if (condition.evaluate(this)) return this;
else return EMPTY_PROVISION;
}
}
class EmptyProvision extends ModelInstance implements Provision {
// attributes
public void setProvision_Id( UniqueId ref_Provision_Id ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public UniqueId getProvision_Id() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setName( String m_Name ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public void setInformalName( String m_InformalName ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getInformalName() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setDescrip( String m_Descrip ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
public String getDescrip() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public String getPathFromComponent() throws XtumlException {
throw new EmptyInstanceException( "Cannot get attribute of empty instance." );
}
public void setPathFromComponent( String m_pathFromComponent ) throws XtumlException {
throw new EmptyInstanceException( "Cannot set attribute of empty instance." );
}
// operations
// selections
@Override
public SatisfactionSet R4002_defines_required_satisfication_Satisfaction() {
return (new SatisfactionSetImpl());
}
@Override
public InterfaceReference R4009_is_a_InterfaceReference() {
return InterfaceReferenceImpl.EMPTY_INTERFACEREFERENCE;
}
@Override
public ProvidedExecutablePropertySet R4501_implements_ProvidedExecutableProperty() {
return (new ProvidedExecutablePropertySetImpl());
}
@Override
public String getKeyLetters() {
return ProvisionImpl.KEY_LETTERS;
}
@Override
public Provision self() {
return this;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public Provision oneWhere(IWhere condition) throws XtumlException {
if (null == condition) throw new XtumlException("Null condition passed to selection.");
return ProvisionImpl.EMPTY_PROVISION;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy